Goals
In this assignment, you will practice the following computer science concepts:
- Creating and Using Objects
Computer Science Principles Curriculum
- Big Idea: Creativity: EU 1.1, LO 1.1.1, EK 1.1.1B, EU 1.2, LO 1.2.2, EK 1.2.2A, EK 1.2.2B
Common Core Standards
- English Language Arts Standards ยป Science & Technical Subjects: CCSS.ELA-Literacy.RST.9-10.2, CCSS.ELA-Literacy.RST.9-10.3, CCSS.ELA-Literacy.RST.9-10.4, CCSS.ELA-Literacy.RST.9-10.5, CCSS.ELA-Literacy.RST.9-10.6, CCSS.ELA-Literacy.RST.11-12.2, CCSS.ELA-Literacy.RST.11-12.3, CCSS.ELA-Literacy.RST.9-10.1, CCSS.ELA-Literacy.RST.9-10.2, CCSS.ELA-Literacy.RST.9-10.7, CCSS.ELA-Literacy.RST.9-10.8, CCSS.ELA-Literacy.RST.9-10.9
- Standards For Mathmatical Practice: CCSS.Math.Practice.MP1, CCSS.Math.Practice.MP2, CCSS.Math.Practice.MP5, CCSS.Math.Practice.MP6, CCSS.Math.Practice.MP7, CCSS.Math.Practice.MP8, CCSS.Math.Content.HSF.IF.A.1
Overview
In this assignment, you will write a program that positions images in the game window to create a scene. Download this zip folder for a template to help you get started.
Goal 1: Creating and Using Objects
Open the provided template and navigate to the main.quorum file. There are five different image files included in the project template.
They contain images of a bush, a rabbit, a log, a rock, and some trees.
We will use these images as well as some Drawable
shapes, setting their positions in the game window, to create a nature scene.
Activity: Declare the Drawable
objects we will need.
Since there are five image files, we will need five Drawable
objects. In addition to these five objects, we will need an additional three Drawable
objects, two that will load rectangles and one that will load a circle.
The first rectangle will represent the green grass in the scene and the second rectangle will represent the blue sky.
The circle will represent the sun. Finally, we will need a Color
object in order to load our rectangles and circle with colors other than the default of black.
Declare these objects below the line that begins with Class Main is Game
and above the line that begins with action Main
.
Once we have declared our objects, we need to load images to them. Navigate to the action CreateGame
action. This is where we will write our code to load images to the Drawable
objects and position them on the screen.
Example: Load two rectangles and a circle to Drawable
objects and set their position
action CreateGame
// this line loads a filled rectangle of width 800 and height 300 and
// colors it green
ground:LoadFilledRectangle(800, 300, color:Green())
// this line loads a filled rectangle of width 800 and height 300 and
// colors it cyan (light blue)
sky:LoadFilledRectangle(800, 300, color:Cyan())
// this line loads a filled circle of radius 50 and colors it yellow
sun:LoadFilledCircle(50, color:Yellow())
// this line sets the position of our sky to the top half of the game
// window
sky:SetPosition(0, 300)
// this line sets the position of our sun to the top right of the game
// window
sun:SetPosition(570, 450)
// these lines add the objects to our game
Add(ground)
Add(sky)
Add(sun)
end
Activity: Declare an ImageSheet
object.
This assignment makes use of a texture atlas (also known as image sheets in Quorum). To get an introduction to using image sheets, refer to this tutorial . An image sheet has been provided for you in the template, called "forrest.atlas".
To find this image sheet, look in the folder labeled "resources" in our project.
To make use of our image sheet, we had to declare the use statement at the top of our program to let Quorum know that we want access to image sheets.
Notice the statement use Libraries.Game.Graphics.ImageSheet
has already been included at the top of our program.
Declare an ImageSheet
object in the same place we declared our other Drawable
objects.
Now that we have our ImageSheet
declared, we can begin using it to load images to our Drawable
objects. Navigate back down to the action CreateGame
action.
Example: Load an image sheet to our ImageSheet
object
// this line tells our ImageSheet object which image sheet we want to load
sheet:Load("resources/forrest.atlas")
Once we have our ImageSheet
loaded, we can use it to get images for our Drawable
object. We do this by calling the GetDrawable(text name)
action on our ImageSheet
. Images in ImageSheet
objects are named and accessed by the file name of the image minus the extension.
For example, if our ImageSheet contains "dog.png" and we wanted to get the image represented by "dog.png", we get it by asking the ImageSheet
for "dog".
Example: Use an ImageSheet
to load an image to a Drawable
// this line tells our Drawable bush to load the image received from
// the image sheet called "bush". "bush.png" was an image file included
// in the ImageSheet
bush:Load(sheet:GetDrawable("bush"))
Activity: Load images to the other Drawables from the ImageSheet
and set their positions on the screen.
sheet:GetDrawable("bush")
returns a Drawable
object with the image of a bush loaded to it.
We use this Drawable to load the bush image to our bush Drawable.
Each statement to load images to Drawables from ImageSheets will look similar to the above line of code.
Drawables loaded from image files act the exact same way as drawables loaded from ImageSheets.
Once images have been loaded to our Drawable
objects, recall that the positions of our Drawables can be set by using the SetX(number x)
, SetY(number y)
or SetPositon(number x, number y)
actions.
Don't forget to add the Drawables to the game so that they appear on the screen!
Now, it's your turn to be creative! Create a scene by moving the Drawable
objects to different positions on the screen.
Do you want the trees to appear as if they are in the foreground or the background?
Do you want the bunny to be on top of the rock? It's all up to you!
Sample Output
Each student will produce a slightly different image. An example image is shown below.
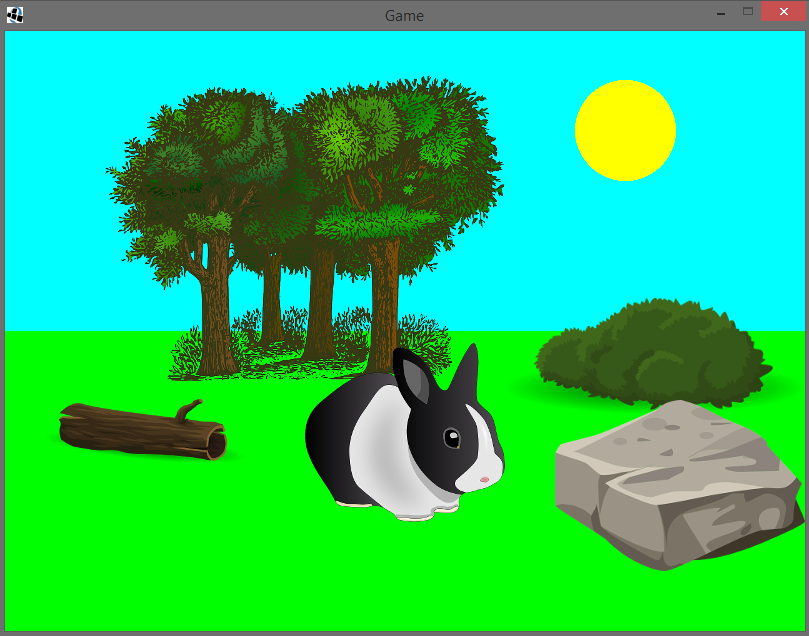
Next Tutorial
In the next tutorial, we will discuss Challenge 2.4, which describes how work Choose your own pattern in Quorum..