Drawing in 2D with Quorum
This tutorial tells you how to draw items on the screen in 2 dimensions with QuorumAn Introduction to Drawing in Quorum
In this tutorial, we describe how to draw objects on the game screen in Quorum. This tutorial will cover primary topics: 1) drawing shapes, 2) drawing images, and 3) ImageSheets. The purpose of these topics is to give the reader a primer on how the drawing system works, so that he/she can draw shapes and images in the game correctly and efficiently.
Drawing Shapes
We start this tutorial with the templated Game Application from the New Project window, as described in the Getting Started tutorial. Our basic game code (with comments omitted) looks like this:
use Libraries.Game.Game
class Main is Game
action Main
StartGame()
end
action CreateGame
end
action Update(number seconds)
end
end
This template creates the architecture that tells Quorum to create a new game and begin execution of the Main Loop. Behind the scenes, this connects to a very powerful utility called OpenGL. OpenGL is an industry standard graphics library specifically designed for drawing graphics. While OpenGL is very powerful, it is not particularly easy to use. In order to understand its details one must have an understanding of a mathematical theory called Linear Algebra, which is an advanced topic that is typically taught at universities. The Quorum Game Engine handles all this complexity for us though so we just need to learn how to call the methods of the built in libraries to generate drawable images and shapes.
To draw shapes to our empty game program, we need to add a use statement to our program to tell the compiler where to find the commands for a class in the standard library named Drawable. The code for the library reference is:
use Libraries.Game.Graphics.Drawable
This line of code tells Quorum that we want to use the Drawable class in our application. In other words, it tells the compiler that we want to be able to add items to the screen that will be drawable in the frame drawing portion of the Main Loop. Once we have access to the Drawable class, we need to create and name a drawable object (another word for this is "instantiate"). We can do this by adding another line of code just below the "class Main is Game" line, such as:
use Libraries.Game.Graphics.Drawable
class Main is Game
Drawable rectangle
While this code creates a drawable object, it does not draw anything on the screen or load an image to associate to the object. In order to do that, we need to insert code into the CreateGame action:
action CreateGame
//This line of code draws a rectangle with a width and height of 50.
//By default, the coordinates of this object are 0,0, which is the bottom left corner of our window.
rectangle:LoadFilledRectangle(50, 50)
//This line of code tells Quorum to add our drawable object to our game,
//so that the game engine puts it in the list of items that it draws on each frame.
//If we forget it, Quorum will have no way to know we want to draw this object.
Add(rectangle)
end
Once we have these lines of code in our program we can Run our game (F6) which will now look similar to this:
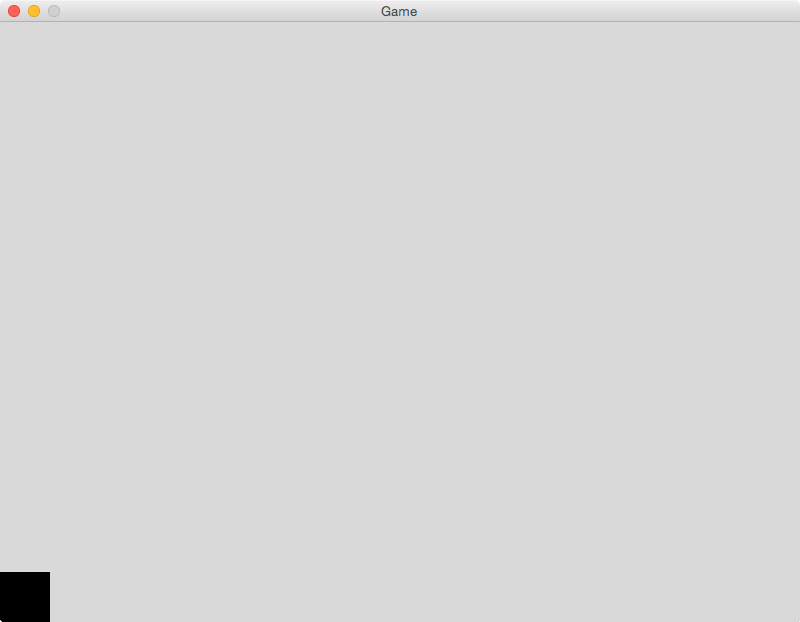
There are many kinds of additional shapes that can be drawn by default. We can use these shapes in combination to create complex pictures. The full list of shapes is in the Drawable documentation. The list includes: 1) circles, 2) triangles, 3) rectangles, and 4) lines. In the case of of circles, triangles, and rectangles, the shapes can either be filled or not and a color can also be specified (if none, the default color is black).
Try it Yourself!
Press the blue run button to execute the code in the code editor. Press the red stop button to end the program. Your program will work when the console outputs "Build Successful!"
Next Tutorial
In the next tutorial, we will discuss Drawing 2D images, which describes how to draw 2D images.