Mouse Events
This tutorial shows how to use mouse events in Quorum.Using MouseEvents
We can also use events for mouse input. There are three possible listeners for MouseEvents.
- A MouseListener is used to listen for when mouse buttons are clicked or released.
- A MouseMovementListener is used to listen for when the mouse is moved or dragged.
- A MouseWheelListener is used to detect when the mouse wheel is scrolled up or down.
Using MouseListeners
In this next sample program, we will use a MouseListener to make a square change color while we are clicking on it, and return it to its previous color when we release the mouse button. Try the total code out below in the browser or Quorum Studio:
Notice that when we create our class using the line class Main is Game, MouseListener
, we inherit from MouseListener, similar to how we inherit from KeyboardListener to listen for KeyboardEvents. We also need to call AddMouseListener to notify the engine like we did before.
// Ensure this object will receive mouse events when it is clicked.
AddMouseListener(me)
In this case, instead of adding the listener directly to the Game class, we show an alternative approach where we add the listener directly to an Item (the square). Functionally the engine calls the predefined actions of the class (if they are specified), however in this case, the listener only applies if the square was clicked. If we wanted different behavior for different objects, we can and add them anywhere.
In any class that inherits from MouseListener, the ClickedMouse action will automatically be called when the user clicks the mouse. This action must take a MouseEvent as a parameter. The MouseEvent can contain lots of information, including what mouse button was clicked or the X,Y coordinates of the mouse when the event occurred. To see everything that a MouseEvent can contain, see its full documentation here.
action ClickedMouse(MouseEvent event)
SetColor(clickColor)
end
Similarly, a class that inherits from MouseListener can use the ReleasedMouse action to automatically call code when the user releases a mouse button. This action must also take a MouseEvent as a parameter.
action ReleasedMouse(MouseEvent event)
SetColor(releaseColor)
end
The image on the left is when the game starts and the image on the right is when the image is clicked on
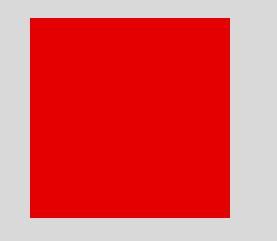
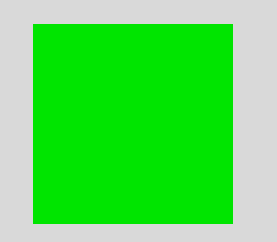
The code in Main is used to additionally position and draw our square on the screen.
Using MouseMovement
MouseEvents can also be used with MouseMovementListeners to detect when the mouse is moved or dragged (which means that the mouse was moved while a mouse button was held down). In this next sample, we will make a square rotate when the mouse when the mouse is moved and make the square move when dragged.
Just like the other listeners, when we create our class with the line class Main is Game, MouseMovementListener
, we need to inherit from the listener we are using, which in this case is the MouseMovementListener
and the Game. This lets us create our two special actions that will be called automatically for us.
The first of these two actions is MovedMouse. This action will be called anytime the mouse is moved and there are no buttons held down on the mouse. In our example, we use event:GetX()
and event:GetY()
to get the x, y coordinates of the mouse after it has moved.
// This action will be called on a mouse event
// because the mouse was moved with no mouse buttons being held down
action MovedMouse(MouseEvent event)
// This code rotates the square.
number x = event:GetX()
square:Rotate(x * 0.1)
end
The other action that will be automatically called by inheriting from MouseMovementListener is the DraggedMouse action. This action is called when the mouse is moved while holding down a button. In our example, we use event:GetMovementX()
and event:GetMovementY()
to get the distance traveled by the mouse since the last time the mouse was moved or dragged.
// This action is called when moving the mouse while holding down a button.
action DraggedMouse(MouseEvent event)
// This code drags the square
number x = event:GetMovementX()
number y = event:GetMovementY()
square:Move(x, y)
end
The most important thing in our Main class is the line AddMouseMovementListener(me)
. This line tells the game engine to notify us every time the mouse is moved or dragged.
Using MouseMovementListeners
The last type of listener for MouseEvents is the MouseWheelListener. In this sample, we will use a MouseWheelListener to rotate a triangle on the screen when the user scrolls the mouse wheel.
Inside our class there is one action, action ScrolledMouse(MouseEvent event)
. This is the only action that will be called when using a MouseWheelListener. Inside this action we use event:scrollAmount
. (Note that it is lower-case without parenthesis - we are directly using a variable inside event, instead of calling an action.) The scrollAmount
value represents how far the mouse wheel has been scrolled. If the wheel was scrolled up, it will be positive, and if it was scrolled down, it will be negative. The faster the wheel was scrolled, the larger the number will be.
To make the game inform our listener of events, we need to add it. We can see this in our example code on the line AddMouseWheelListener(me)
.
Next Tutorial
In the next tutorial, we will discuss Collision Events in Quorum, which describes How to use collision events events in our games..