Tutorial: Using Motors with LEGO™ Robots
This tutorial explains how to use motors on robots in Quorum.Using motors with Robots in Quorum
In this tutorial, we will become more familiar with how to use the motors of the robots. The topics discussed will include:
- How motors work in general.
- The differnent ways that you can use motors in a program.
How Motors work
A motor is only able to perform a single specific task: rotation. A motor can either rotate backwards (with the RotateBackwards() action) or forward (with the RotateForward() action). All robot movement is controlled through motors.
It is important to understand the orientation of the motor movement in order to give the robot instructions. The RotateForward() action rotates the motor forward away from the connection port. It may help to think of the "front" of the motor as the part that rotates and the "back" of the motor as the end that has the connection port. The image below illustrates the forward direction of a motor.
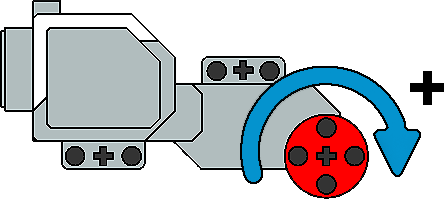
The amount that a motor rotates is measured in degrees, where a rotation of 360 degrees means the motor has completed one full revolution. Rotation can also be specified as a negative number to indicate a reverse rotation. A rotation of -360 degrees will cause the motor to complete one revolution backwards.
With Quorum, the speed at which a motor rotates is measured in degrees per second. So, with a speed of 360 degrees per second (the default setting) a motor will complete one revolution every second. Similarly, a motor speed of 720 will cause the motor to complete two full revolutions every second. The maximum speed for a motor varies since it will depend on battery voltage and the other things that the robot is doing. Generally, the range of speed is between 600-900 degrees per second.
There are two types of motors, large and medium. The large motors are generally used to move a robot along a surface, while the medium motors usually control other parts of the robot. The large motors allow parts to be connected on either side, while the medium motors only allow a component to be connected to its front. An illustration of the medium motor is shown below. Despite their differences, however, any motor action can be used with either type of motor.
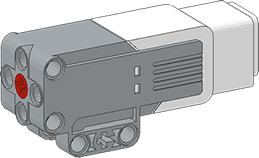
When connecting motors to the robot, it is important to note which motor is plugged into which port. The motor ports on the brick are on top and labeled A, B, C, and D. We will give instructions to the motors using these letters in our code.
Now that we have a better grasp on the basics of motors, we can explore different ways they can be utilized in a program.
Programming Motors
The Quorum Robot Library provides actions for controlling motor movement, speed, and other information.Motor Movement
- RotateForward
- RotateBackward
- RotateByDegrees
- RotateToDegree
- Stop
- Wait
With this set of actions, we can instruct the robot to move a certain amount or to move until we tell it to stop. If we want a motor to finish its rotation before the program moves on to the next line of code, we tell the program to wait for that motor to finish. The following program demonstrates these actions:
use Libraries.Robots.Lego.Motor
Motor motor
//rotates one revolution
motor:RotateByDegrees("C", 360)
motor:Wait("C")
//does nothing, motor is already at 360
motor:RotateToDegree("C", 360)
motor:Wait("C")
//rotates one more revolution
motor:RotateByDegrees("C", 360)
motor:Wait("C")
//rotates backwards two revolutions
motor:RotateToDegree("C", 0)
motor:Wait("C")
Motor Speed
- SetSpeed
As mentioned above, the speed of a motor's rotation is measured in degrees per second. The following program shows a demonstration of how to use the SetSpeed() action.
use Libraries.Robots.Lego.Motor
Motor motor
//motor B will rotate 2 revolutions per second
motor:SetSpeed("B", 720)
//motor B should reach 1440 degrees in 2 seconds
motor:RotateByDegrees("B", 1440)
motor:Wait("B")
Motor Information
- GetRotationTarget
- ResetRotation
- GetRotation
- IsMoving
- GetSpeed
When a program is running, information is stored for each connected motor, including what degree a motor is moving to, how far it has already rotated, and the speed at which it is moving. We can also detect stalls by asking if the motor is currently moving. Lastly, we can reset the motor's rotation information back to 0.
use Libraries.Robots.Lego.Motor
use Libraries.Robots.Lego.Screen
Motor motor
Screen screen
//returns the default of 360, since we haven't set the speed
motor:GetSpeed("A")
motor:RotateByDegrees("A", 1080)
screen:Output("Rotating to: " + motor:GetRotationTarget("A"), 0)
repeat while motor:IsMoving("A")
//displays real-time speed on the screen
screen:Output("Speed: " + motor:GetSpeed("A"), 1)
end
//GetRotation will return 1080, since that's how far it has moved
screen:Output("Rotation: " + motor:GetRotation("A"), 2)
motor:ResetRotation("A")
//now returns 0, since we reset the rotation information
screen:Output("After reset: " + motor:GetRotation("A"), 3)
//do one more rotation so we can look at the screen
motor:RotateByDegrees("A", 1080)
motor:Wait("A")
Additional Information
- The default rotation speed of a motor is 360 degrees per second.
- Class constants can be used to refer to motors when passing them to an action. For example, if we have a Motor object called
motor
, then the codemotor:Stop("A")
is the same thing asmotor:Stop(motor:MOTOR_A)
For documentation on the Motor class, see here.
Next Tutorial
In the next tutorial, we will discuss Sensors, which describes how to use lego sensors.