Tutorial: Playing Sounds in Quorum
This tutorial teaches you how to play audio files in Quorum.An Introduction to Playing Sounds in Quorum
This tutorial will explain how to add and manipulate sounds by using the Quorum Audio library. In order to access the Audio library, we must include the use Libraries.Sound.Audio
statement at the beginning of our class definition. The Quorum Audio library supports files with either .wav or .ogg extensions.In order to use sounds, we first need to place a copy of the sound file into our project. It does not matter where in our project we place it, but for this example, we make a new folder in the main project folder called "Sounds." It is placed next to the folder labeled "Source Code."This looks like so:
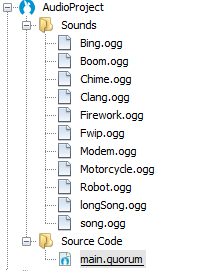
To make things easier to get started in this tutorial, a project containing many different sounds has been provided in this zip file.
Getting Started: Playing Sounds
In order to play a sound in our program, we need to instantiate an Audio object and then load an audio file into it in the same way we loaded an image into a Drawable previously. Once we have created an Audio object, we set the audio file for the object by calling the Load action. There are two ways we can set the file for an Audio object. The easiest way is just to call Load with a file path in quotes (shown below for the object audio1
). The other common way is to create a Quorum File object, set the path of that object to the audio file, and then pass the File object to the Audio object to the Load action (shown below for the object audio2
). Both of these methods are correct.
// these statements tell Quorum we will be using the Audio and File libraries
use Libraries.Sound.Audio
use Libraries.System.File// these statements demonstrate how to load a file into an Audio object by
// passing the path of the audio file as text to the Load action
Audio audio1
audio1:Load("Sounds/Bing.ogg")// these statements demonstrate how to set the file of an Audio object by
// creating a File object, setting the path of that File object, and passing the
// File object to the Audio object's Load action.
Audio audio2
File file
file:SetPath("Sounds/Boom.ogg")
audio2:Load(file)
Once we have our Audio object created and loaded, we can play our Audio object by calling the Play action. It is important that the Audio object we want to play has a file loaded to it; otherwise our program will not work.
// this statement demonstrates how to play an Audio object.
audio1:Play()
You may have noticed that when we have a sound that lasts longer than a few seconds, our program ended before the sound finished playing. If we haven't prevented the program from finishing before our sound is done playing, our program will end before the sound is done and it will cut off. A simple remedy for this is to use the PlayUntilDone action to play our Audio object instead of using the regular Play action. Be aware that any statements after the call to PlayUntilDone will not execute until our sound is done playing. An example using the PlayUntilDone action is below.
// this statement demonstrates how to play an Audio object until it is done
audio2:PlayUntilDone()
Next Tutorial
In the next tutorial, we will discuss Changing the Audio: Controlling Volume and Pitch, which describes how to play audio files Quorum.