Tutorial: Writing Quorum Plugins
How to connect to Java (or other languages) from QuorumCalling Java or C++ from Quorum
It is sometimes necessary, in any programming language, to want to use a feature found in another, like Java or C++. Given this, many languages provide a method for calling from one language to another, and this includes Quorum. In Java, for example, we might use the Java Native Interface (JNI) to call down to C or C++. Or, in C, we might embed assembly code. In Quorum, we accomplish the same kind of idea through plugins. On this page, we describe how we write plugins for the Java programming language. As this is an advanced topic, we recommend being familiar with Quorum before attempting this material. Note as well that this guide barely scratches the surface of what is possible with Quorum's plugin system. Advanced users that want more information should ask through our various social media services (e.g., email list, Facebook) if more information is needed.
Creating System Actions
In order to make a call down to Java, we first must write a Quorum class that defines "system actions." A system action, by definition, is an action that calls down to another programming language.
package Libraries.Mine
class MyPlugin
system action Test
end
In the above code, notice that we have defined a regular class, but we have an action with the word "system"before it, with no "end" at the end of the line. This signifies to the compiler that the content of this action will be defined by Java. Here is a second example with more complex system actions.
package Libraries.Mine
class MyPlugin
system action Test
system action Add(number a, number b) returns number
end
Notice that the second action defines parameters, return values, and otherwise looks like a normal action. This is normal, as all features of a typical action are allowed in system actions.
Creating the Java Plugins
Once we have created our system actions, we need to create corresponding Java plugins, so our system actions have something to call. Let's define a plugin for our second code example to show how this can be done.
package plugins.quorum.Libraries.Mine
public class MyPlugin
public java.lang.Object me_ = null;
public void Test() {
}
public double Add(double a, double b) {
return a + b;
}
end
In the first line of our plugin, notice that the package is defined as plugins.quorum.Libraries.Mine. This is required, as the Quorum compiler will expect that, since we are defining a system action in the class MyPlugin in the package Libraries.Mine, that the Java plugin is also called MyPlugin and that it is located in the package plugins.quorum.Libraries.Mine.
Second, notice that Quorum actions translate directly into public methods in the plugin. The Test action, which in Quorum has no return type, translates into a void method in Java. Similarly, actions in Quorum that have parameters are translated according to their values. With primitives, the translation is integer to int, number to double, boolean to boolean, and text to String. For object types, an object with a fully qualified name of org.Test in Quorum would be quorum.org.Test when used in a parameter.
As one final point, notice the line public java.lang.Object me_ = null;. All Java plugins for Quorum are required to have this line, otherwise we will throw an exception at runtime. This line allows Quorum to pass the original object that will call down to our plugin, in case we need it. This variable, me_, will be a valid value once the plugin first boots.
Using Plugins
We can add plugins directly compiled with other tools directly from the user interface in NetBeans. To do this, we first either use the keyboard hotkey or the mouse to right click on our project and select Properties. This will open a window that looks like the following:
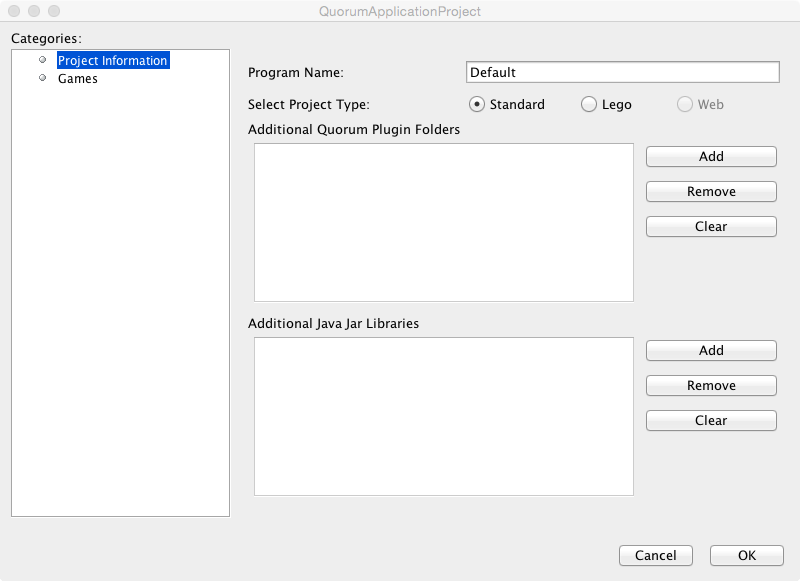
This window contains a variety of options for projects and contains two panes. On the left pane are two options, Project Information and Games, which can be navigated with the up and down arrow keys as well as the mouse. On the right is the project information tab, which allows us to change the project type (normal or Lego), the project compiled name, and has two additional text boxes for plugins or Jar files.
If we have created a plugin, we can add it into the compiled source of our project by selecting the Add button in the tab order of the pugins List. This will open a dialog and we can select the build folder of where our plugin was built. Finally, if all plugins are contained in a jar, we can similarly load a jar file using this window, which will cause Quorum to write it into the path of the executable we are building.
End of Lesson
You have reached the end of the lesson for Libraries and Structures. To view more tutorials, press the button below or you can go back to the previous lesson pages.