Variables, Conditionals, and Functions - Lesson 3: Variables Practice
Overview
In this lesson students spend most of their time practicing using the skills and processes they have learned about variables. At the conclusion of the lesson students discuss the main things they realized and still have questions about at the conclusion of this lesson.
Goals
Students will be able to:
- Write programs that use variables and expressions with the support of sample code.
- Debug programs that use variables and expressions
Purpose
This lesson is students primary opportunity to get hands on with variables in code prior to the Make activity in the following lesson. Give students as much class time as you can to work through these. For this lesson it's recommended that you place students in pairs as a support and to encourage discussion about the challenges or concepts they're seeing. In the following lesson students are encouraged to work independently.
Resources
- Debugging Global vs. Local Variables (Video)
- EIPM: A Short Introduction (Teacher Resource)
- CSP-Widgets Repository
Teaching Tip
This is the first official "Practice" lesson in the EIPM model. Review the EIPM model in theEIPM: A Short Introduction (Teacher Resource).
Practice Lessons:
Overview: Students practice using the new concept through a scaffolded series of programming activities.
- Students work independently or in pairs
- Teacher introduces debugging practices that the beginning of the Activity and circulates the room during the lesson to provide targeted support.
Goal: Students gain confidence in writing and debugging programs that use the new concept.
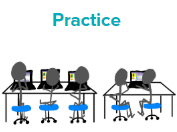
Watch the following videos:
Getting Started (0 minutes)
Remarks
- Today we're going to have a chance to practice programming with a lot of the concepts and patterns we've explored over the last two lessons. I encourage you to go through these with a partner, but pay close attention to what each other is doing. In our next lesson you're going to have to use a lot of these on an independent project, and these activities are good practice for what you'll find there! Alright, let's get to it!
Teaching Tip
Move Quickly to the Activity: There's a lot in the main activity of today's lesson. You may optionally wish to do a quick vocabulary review or address any questions that came up in the last lesson. Otherwise, give students more time to get hands on with some code.
Activity (40 minutes)
Group: It is recommended that students work in pairs for this lesson and a number of the activities feature discussion prompts. Consider using pair programming, having drivers and navigators switch every 3 minutes, not on every project.
Distribute: Optionally pass out a plastic cup or other manipulative they can place on their computer when they are stuck as a signal that they need support.
Remarks
- Today you're mostly going to practice what we've learned about programming with variables. As always you should be using the debugging process to help you as you work on issues.
- Let's review the debugging process first. Debugging is the process of finding and fixing problems in code. We previously talked about these four steps: Describe, Hunt, Try, and Document.
- Today we're going to be focusing on our debugging skills while we practice. Don't forget to use
output
to check the values of variables!
Open a Project: Students will use a number of projects for this lesson, beginning with Lesson3_App1. Each project has instructions in a comment that describes what students should do for that project. When they finish one project, they can proceed to the next one.
Teaching Tip
Providing Support: Circulate around the room through the lesson encouraging students to use the strategies introduced in the previous lessons. Students have a number of supports at their fingertips, so a big part of your role is helping build their independence in using those resources.
Projects 1-2 Assigning Numbers and Strings: These projects only use the output
commands which prints text in the console. Some key points in these levels:
- Students are asked to discuss what they see with a partner
- These projects heavily reinforce language around assigning and creating variables
- Project 2 is a debugging-heavy level which introduces more rules about syntax.
Projects 3-7 Variables and Operators: These projects have students practice writing more complex expressions and using operators. A few tricky things to look out for
- These levels transition from using only
output
to using full apps with user interfaces. - Project 3 has a tricky question regarding the difference between
1.0/7.0
and1/7
. In the first case, the numbers have a decimal component, so the operation will create a fraction. In the second case, it's dividing by whole integers with no fraction, so 1 divided by 7 equals 0! - Project 5 introduces the concept of multi-line text
- Students continue to be encouraged to discuss what they are learning with a neighbor
- Projects 6 and 7 introduce the use of the counter pattern with numbers and strings. Students may need to be reminded of the pattern.
Regroup: Bring the class back together to watch the Scope Practice Video.
Teaching Tip
Teaching Tip: The concept of variable scope can be difficult for students to understand. It is highly recommended that you watch the video prior to the lesson. Slides are available for this lesson if you would like to optionally review as a class.
Display: Show the Scope Practice video.
Do This: Discuss these differences between global and local scope, and debugging tips for dealing with them.
Create Variables Once At the Top of Your Codes
When you create variables you should:
- Create each variable only once. You don't need to create variables twice and this can cause errors.
- Create your variables at the top of the
class
section. This keeps your code organized and easier to read for you and others. - Avoid creating variables inside of actions. Read on to understand why.
Global vs. Local Variables
There's two types of variables, global and local, and so far we've only focused on global variables. Here's the main difference between global and local variables.
- Global Variables are permanent. They can be used anywhere in your code. Variables created outside of an
action
section are global. - Local Variables are temporary. They can be used only in the part of the code where they were created, like inside an
action
. These are deleted once code section is done running. Variables created insideaction
orif
blocks are local.
Avoiding Local Variables and Debugging
Local variables will eventually be useful but for now they're most likely to just be confusing. The biggest issue you'll run into right now with local variables is accidentally creating a variable in an action
while trying to use a global variable. Here's what the code usually looks like:
integer count = 0
action ButtonActivated(Button button)
integer count = count + 1
end
This code is pretty confusing. While it looks like there's only one variable being used, it actually has two variables, one local, and one global, and they're both named count
! Changing the value of one will have no impact on the other. This can cause unexpected behavior in your code and it can get tricky to debug.
The best way to avoid these issues is to make sure for now that you're not using variable creation commands inside of an action
. If you run into a tricky debugging problem, check if you're accidentally creating a local variable.
Resume the Activity
Project 8 Debugging Scope Issues: This project highlights a common bug that can come up when working with variables. While students should be aware of this bug, they don't quite have the background they'd need to fully understand it. For now support students in following the three main takeaways.
- Create variables once
- Create variables at the top of the
class
section - Don't create variables inside
action
blocks
Project 9 Putting It All Together: This project asks students to put together many of the concepts they've seen so far. This is an opportunity to have a bit more of a "blank screen moment" while still being able to use some starter code as a guide.
Wrap Up (5 minutes)
Discuss: What aspects of working with variables do you feel like clicked today? What do you still feel like you have trouble with?
Discussion Goal
Discussion Goal: Use this opportunity to address any lingering questions or misconceptions in the room. You can also use this as a source of discussion topics to kick off the following lesson. As you lead the discussion, call out the many resources students have access to help when they're getting stuck.
Discuss: Have students share with one another before sharing with the whole class.
Remarks
- Variables can be a little bit tricky, but I saw a lot of good progress today in nailing down this concept. We may have a few lingering questions, but you also have a lot of resources available. Next time you'll have a chance to put all this together by programming an app that starts with "the blank screen"!
Assessment: Check for Understanding - AP Practice
For Students
Open a word doc or google doc and copy/paste the following question.
Question
What will be displayed after this code segment is run?
myPoints <- 2
myPoints <- 5
myPoints <- myPoints + 1
DISPLAY myPoints
Standards Alignment
- CSTA K-12 Computer Science Standards (2017): 2-AP-11
- CSP 2021: AAP-1.B.2
- CSP 2021: AAP-2.C.2, AAP-2.C.3
- CSP 2021: AAP-3.A.6, AAP-3.E.1
- CSP 2021: DAT-1.B.2
Next Tutorial
In the next tutorial, we will discuss CSP Unit 4 Lesson 4 Variables Make, which describes Practice using variables.