Getting Started with Games
Understanding and making your first Quorum gameHow a Game Works
Before we can get started making games, we need to understand two basic concepts for the Quorum Game Engine.
Understanding Frames
In films or videos, a frame is a single still image, and the film or video is made of many frames, where the still images are shown one after another very quickly to produce the appearance of motion. Games work under a similar concept except that a game generates it's frames "on the fly" based on what actions the player takes or the game rules dictate instead of a director generating them ahead of time. In a game, at any given point in time the engine must display the current frame to the player based on the state of objects in the game at that moment.
The game engine displays its frames at a certain rate based on the capabilities of the machine it is running on and the activities of the game. Frames are important for timing purposes and updates to objects can be made at each frame by calculating the time that elapses between them.
Understanding the Main Loop
In a movie, every frame that the viewer sees has been made in advance, so playing back a movie is simply a matter of displaying images at a pre-set frame rate. Since games must create a frame before it is shown to the player, the game engine must provide a capability for a programmer to write code to update all of the objects in the game before each frame update. The game program then loops over and over again until the game ends, calling this update function on each frame before it displays the objects in the game. This loop is called the Main Loop and the function that the game engine calls on each frame is called Update. In the Update function, a programmer will update things like the position of displayable objects, monitor events or input, check for collisions between objects play sounds or music, enforce game rules, check the status of certain conditions, or many other things.
When the Update code block has finished execution on a particular frame, the game draws the game objects on the screen, and control goes back to the beginning of the main loop and calls Update again to repeat the process. All of the game's logic is therefore contained in or called from the Update code in the main loop.
Anatomy of a Game Class
Now that we understand these basic concepts, we can create the skeleton of a new game. In NetBeans, we will make a new Quorum project with a "Game Application" template. You can find complete steps for making a new Quorum project at the Getting Started with Quorum tutorial. When the project is made, we have a template game ready to work on. Let’s look at what each key part of the template code does.
Breakdown
Following is a line by line breakdown of the template code:
use Libraries.Game.Game
class Main is Game
This line instructs the compiler to use the Game functionality built into the Quorum Standard Library. For more information on how loading libraries works, see Finding and using Libraries with Quorum.In large projects, we have multiple code files. When a Quorum program is run, it will start in the file that has the "Main" class. The second part of this line, "is Game", makes this class a child of the Game class and allows it to use code from the Game file in the Quorum standard library. For more details on how this concept (called inheritance) works, see Inheritance in Quorum.
action Main
StartGame()
end
The Main action in the template code is very simple, it just calls the StartGame method, which is the command to initiate the Main Loop. By starting the Main Loop the engine will begin its process of drawing the objects in the game to the screen (currently there are none) and then calling the Update method (currently empty) on each frame. Other things that are commonly done in this method are things like setting the screen size or its position or other configuration items.
action CreateGame
end
This CreateGame method is called by the engine immediately after the StartGame command is issued. The code statements inside this action block will be executed one time at the startup of the game. It is often used to initialize variables or load objects or other things that must be done to get the game ready to play.
action Update(number seconds)
end
This is the Update method that the engine calls and is executed on each frame. The parameter of the method ("seconds") is provided by the engine and contains the number of seconds that have passed since the last execution of the loop. The time between frames will vary from frame to frame depending on the amount of processing or drawing or other things that the computer may be doing simultaneously, so this parameter is important for the game logic to decide what to do next (such as how far to move an object or to check if it is time to flip the frame of an animation). The parameter "seconds" will generally be a small fraction of a second. For example, it may contain a number such as 0.021238734715 which is roughly 2/100th's of a second).
Run the Program
If we run this default template program (F6), the Quorum game engine will put up a blank screen that looks something like this:
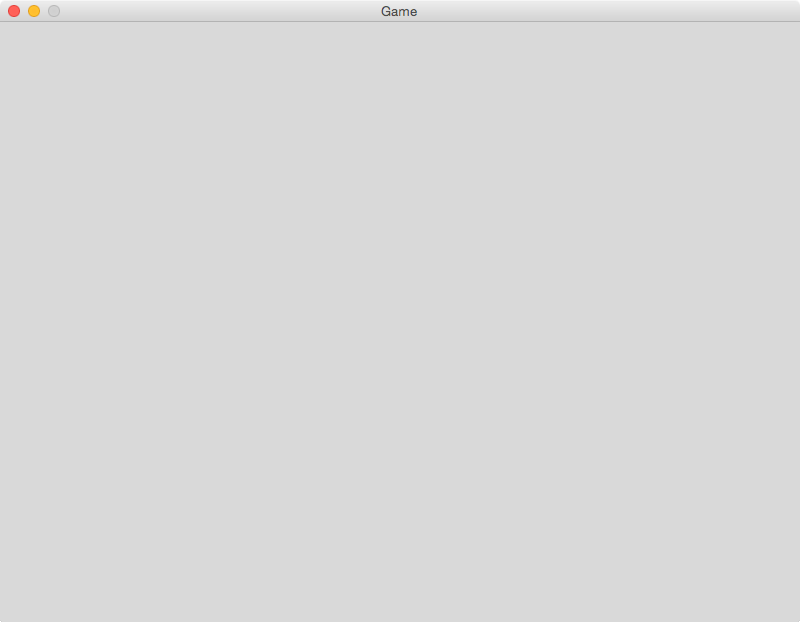
Next Tutorial
In the next tutorial, we will discuss Drawing in 2D, which describes how to draw in 2D.