Drawing Images
This tutorial tells you how to draw images on the screen in 2 dimensions with Quorum2D Image Drawing
In addition to shapes, we can also display pre-made images on the screen. This is useful if we want to use external programs like Photoshop or Gimp (a free alternative) to make images ahead of time or if we want to use digital photos we've taken. Similarly, there is a large amount of free art on the Internet that can be used for commercial or non-commercial purposes (under the creative commons license, for example).
Quorum can load such images in two ways, by using: 1) a Drawable directly, or 2) an ImageSheet (a set of combined images). First let us discuss using a Drawable to load images.
To load an image we take the same approach we used before by creating a drawable object first:
Drawable logo
Then we can load an image file stored on our hard drive by calling the Load method of the Drawable class. For this example, we will load the logo for the Quorum Hour of Code:
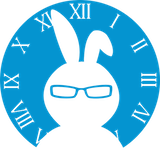
This image is licensed under Creative Commons, so please feel free to download and use it for your own purposes. To load this image, we first need to make a copy of it and place it in the directory of our project. It does not matter where in our project we place it, but for this example we made a new folder named "assets" in the main project directory:
To load the image into our "logo" drawable object, in our CreateGame action we simply call the Load method of the Drawable class with the path and filename of the file in our project and then call the Add action. (Notice that the path of the file is relative to the root of our main project directory.):
logo:Load("assets\hourofcode.png")
Add(logo)
So the whole game code looks like this:
use Libraries.Game.Game
use Libraries.Game.Graphics.Drawable
class Main is Game
Drawable logo
action Main
StartGame()
end
action CreateGame
logo:Load("assets\hourofcode.png")
Add(logo)
end
action Update(number seconds)
end
end
...and when we Run the program (F6) we get a game window that looks something like this:
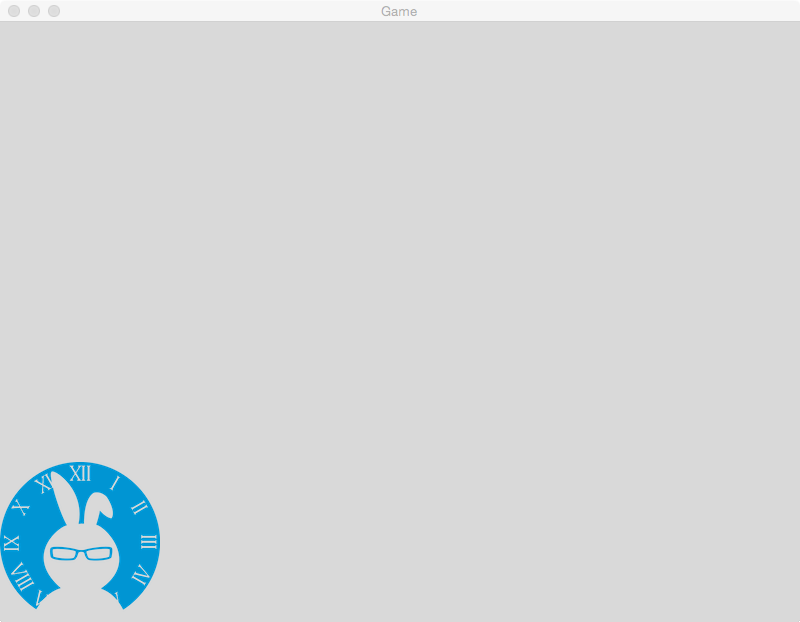
Alternatively, we could use a file class to load the image file into the Drawable. In that case, our code would look like this:
use Libraries.Game.Game
use Libraries.Game.Graphics.Drawable
use Libraries.System.File
class Main is Game
Drawable logo
action Main
StartGame()
end
action CreateGame
File file
file:SetPath("assets\hourofcode.png")
logo:Load(file)
Add(logo)
end
action Update(number seconds)
end
end
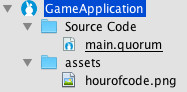
Try it Yourself!
Press the blue run button to execute the code in the code editor. Press the red stop button to end the program. Your program will work when the console outputs "Build Successful!"
Next Tutorial
In the next tutorial, we will discuss Using Image Sheets in Quorum Studio, which describes how to use Image Sheets in Quorum Studio.