3D Models in Quorum
This tutorial explains loading 3D models in QuorumAn Introduction to Using 3D Models in Quorum
This tutorial will cover how to load 3D Models from a file, where 3D models can be found, and it will discuss the potential problems with loading models created by someone else.
In Quorum, we can load 3D models into our games from files. There are many file formats used for these models, but Quorum needs models that are in either .obj file format or .g3db file format. We will discuss where we can find models in these formats and how to solve potential problems later in the tutorial, but first let’s learn how to add these objects to our game.
Loading 3D Models from a File
The first model we load will be a doorframe. Download this file and let’s place it in the directory of a new Quorum Game project.
Loading a model from a file is no different from loading a primitive shape. If we need a refresher on loading primitives, we can go check the Drawing in 3D tutorial. Instead of calling the Model action LoadBox() or LoadCylinder(), we will call the Model action Load(). This action requires the name of our file in quotation marks. In order to load our door into our project, our code should look like:
use Libraries.Game.Game
use Libraries.Game.Graphics.Model
/*
This is a default class for creating a game in Quorum. Note that the Main
action begins by calling StartGame(), which loads a window a game can
be displayed in. The action CreateGame is where the game should load any
assets, like images to be displayed, frames of animation, or sounds to be
played.
*/
class Main is Game
Model door
/*
This action, Main, starts our computer program. In Quorum, programs always
begin from Main.
*/
action Main
StartGame()
end
/*
This action sets up the default values for a game. For example, we might
use the Libraries.Game.Graphics.Drawable class to load up an image or
a shape, or we might add a Libraries.Interface.Button for making something clickable,
or a Libraries.Interface.TextBox for gathering user input in a game.
*/
action CreateGame
door:Load("door.obj")
Add(door)
end
/*
The update action is called on every "frame" of animation in a game. This
action should be used to do operations on items. So, for example, we
might use this action to change frames of animation, to rotate items,
or to make creatures jump.
*/
action Update(number seconds)
end
end
One thing to remember when we load a model from a file is that we may not know the size of the model. For this reason, when we load models, it can be useful to have our program print the dimensions of the model. In order to do this, we can call the output action and tell it to print the x, y, and z dimensions of our door. Let’s navigate to the CreateGame action and write these three lines of code after we load our model:
output door:GetWidth()
output door:GetHeight()
output door:GetDepth()
Now, let’s run our program. The door is not visible in the game screen, but the program will output 3 numbers. In our case, it prints:
159.4470977783203
303.8571243286133
60.58085823059082
Now we know why our door was not visible: it is huge! This is when the scale action we learned in the Animation in 3D tutorial comes in handy. We need to shrink our door by a lot, so let’s add this line of code to our CreateGame action:
door:Scale(0.01, 0.01, 0.01)
Let’s run our program once again (F6). Now our door is visible. Below is an image of our game screen with the door in place:
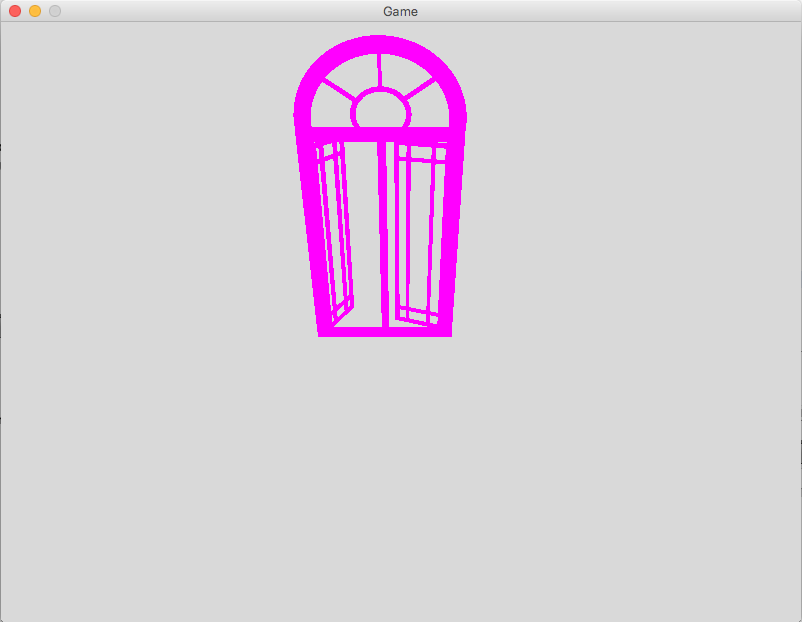
We can now do any of the things we learned in our 3D Animation tutorial (move, scale or rotate) our door.
Where to Find Models
There are many web sites dedicated to providing 3D Models for download (for free or to purchase). Some popular web sites are:
but there are many web sites out there that offer free 3D models. When looking for models, we need to keep in mind that Quorum can only load .obj or .g3db files. One more important thing to consider when downloading models is the license on a model. A license tells us who can use a model, and for what purpose. Just like we need permission to use someone else’s words or images, use of models also requires permission. Many models are free for personal use, but they cannot be shared or used in anything that will be published. Some models are available for commercial use or are in the public domain and we can use them however we want. Whatever the license is on a particular model, we need to understand the restrictions so that we can respect the license.
Licensing and file formats are not our only considerations when using models we did not create ourselves. There are many problems that can happen when we download models from the Internet.
Potential Problems Loading Models
There are hundreds of thousands of models that can be downloaded for free, but we will be unable to load a large portion of these models in a Quorum project. This is a consequence of using something that someone else made. One of the most common errors we will see when loading models involves a missing file. Most .obj files also require a .mtl file that creates a material library. Our door was pink because it did not use any materials, but if the .obj file required a material library, our program would not have been able to load the door without that .mtl file. If a model has a .mtl file, be sure to also place that in the project directory.
So a model could be missing a .mtl file, but even if there is a .mtl file, we could still get an error for a missing file. A material library file will often reference images as textures to put on the model. If the .mtl file uses an image that was not included in the file we downloaded, we will get an error. Sometimes, the author of a model uses images on their own computer to texture their model. If they do not include these images when they upload a model, we will not be able to load the model in our project.
Another thing to consider when we download models is their size. Many models are huge, made up of hundreds of thousands of vertices. Very large models will take a very long time to load in our game, so we need to be conscious of how large a model is.
It can take a long time to find models that have been put together properly, and are not too large. An alternative to downloading models is making our own.
Making our own models
We can also make our own models using tools like Blender or Maya. However, note that model making programs are generally not accessible to people with disabilities, which means we sometimes have to be creative. We can also try experimenting with the primitive shapes in Quorum. For an example of using only primitive shapes in Quorum, check out the next tutorials, on Cameras and Lighting, which only use primitives. In this tutorial, we have learned how to load 3D models, where we can find such models, and some potential problems with downloaded models.
Next Tutorial
In the next tutorial, we will discuss Cameras, which describes how to use cameras.