Tutorial: Button
This tutorial tells you how to use Buttons in QuorumAn Introduction to Buttons in Quorum
In this tutorial, we will learn how to use Buttons in the Quorum Game Engine. A Button is a simple interface tool which executes a Behavior when clicked.
For this tutorial, we will create a Button that says "Hello world!" aloud and outputs it as text each time it is pressed. To start, create a new Game Application project.
Standard Buttons
In order to use a Button, we must include the Button library with the following use statement:
use Libraries.Interface.Controls.Button
Since we want the Button to be usable as soon as the game begins, we'll create it in the CreateGame action. After we create the Button, we can set its position like we would a Drawable, using the SetPosition action. We can also set a name which will be the text the Button displays on the screen. Then we can add it to the game, giving us the following lines of code:
Button button
button:SetName("Click me!")
button:SetPosition(300, 300)
SetFocus(button)
Add(button)
We also added a SetFocus call with the Button as a parameter. We do this so that the Button is accessible, which means we can activate it using only the keyboard. When the Button is focused, you can either click it or press the Spacebar to activate.
So far, the Main class is as follows:
use Libraries.Game.Game
use Libraries.Interface.Controls.Button
use Libraries.Interface.Layouts.ManualLayout
class Main is Game
action Main
StartGame()
end
action CreateGame
ManualLayout layout
SetLayout(layout)
Button button
button:SetName("Click me!")
button:SetPosition(300, 300)
SetFocus(button)
Add(button)
end
end
When we run the program, the Button appears in the Game window as shown below:
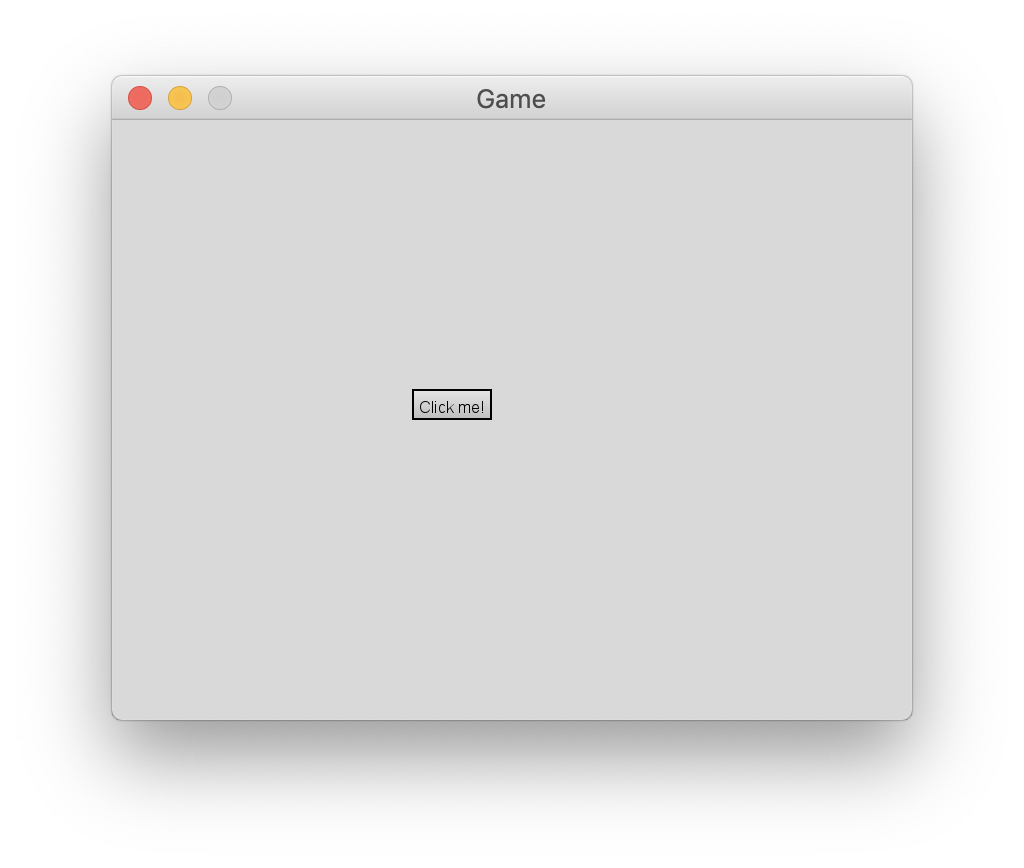
You might notice that the Button seems small for the screen and might be wondering how we could resize it. Right now the Button is sized according the text it displays, and to resize we need to alter the Button's LayoutProperties. However, this is not necessary for the purposes of this tutorial, so for now we'll simply say that Buttons use a Layout to organize their contents. The specifics on how to resize and alter a Button's Layout will be covered later in the Layout tutorial.
Adding Behavior to a Button
Right now, nothing happens when the Button is clicked. To have an action activate when we click the button, we must add a Behavior to the Button.
To begin, create a new Quorum Class, which we will name "OutputBehavior.quorum." This class will use the libraries for Behavior, BehaviorEvent, and Speech. The class itself will inherit the Behavior class, and we will override the Run action to say and output the desired text. This gives the following OutputBehavior class:
use Libraries.Interface.Behaviors.Behavior
use Libraries.Interface.Events.BehaviorEvent
use Libraries.Sound.Speech
class OutputBehavior is Behavior
action Run(BehaviorEvent event)
Speech speech
speech:Say("Hello world!", false)
output "Hello world!"
end
end
Now that we have our completed OutputBehavior class, we can add it to the Button in our Main class using the Button's SetBehavior action. To do so, return to the CreateGame action, and add the following lines:
OutputBehavior behavior
button:SetBehavior(behavior)
This complete Main class is as follows:
use Libraries.Game.Game
use Libraries.Interface.Controls.Button
class Main is Game
action Main
StartGame()
end
action CreateGame
OutputBehavior behavior
ManualLayout layout
SetLayout(layout)
Button button
button:SetName("Click me!")
button:SetPosition(300, 300)
SetFocus(button)
Add(button)
button:SetBehavior(behavior)
end
end
Now, when we run the program, clicking the Button or pressing the Spacebar will say and output the "Hello world!" message.
Accessibility
In our example, we used an action named SetFocus, this action allowed for the Button to be used with only the keyboard. The Focus is covered in a later tutorial, but we use it here to make our User Interface accessible. Accessibility is one of the principles that guides the development of Quorum, and that is why the language Screen Reader is friendly and why Quorum can easily be used to make accessible applications. Focus is what allows the use of a keyboard to interact with the User Interface, and it tells Screen Readers which object's information to read, so use of Focus will be found in most of the User Interface tutorials.
Games made in the Quorum Game engine are made to be compatible with a Screen Reader, and when making accessible applications it is important to understand how a Screen Reader will interact with your application. A Screen Reader will read the name and any text that the Focused object displays. In our Button example, we used the SetName action call, which changes both the display text of the Button and what a Screen Reader will read when the Button has Focus. Using SetName and Focus Cycles allows the creation of accessible applications for users with Screen Readers, or for users that cannot use a mouse.
Run the Program
Next Tutorial
In the next tutorial, we will discuss Layouts, which describes how to use Layouts.