Tutorial: Checkbox
This tutorial tells you how to use Checkboxes in QuorumIntroduction to Checkboxes in Quorum
What is a Checkbox?
In a previous tutorial, we learned how to use Buttons. A Button is simple interface tool that allows Behaviors to run when it is activated. Standard Buttons have many uses, but there is a special class of Buttons that expand functionality beyond a simple press to activate. This special class of Buttons are known as "Toggle Buttons," and they switch between different states. The Checkbox is one type of toggle Button, where activating the box once toggles it "on" and then activating it again toggles it "off."
Having two toggle states differentiates Checkboxes from standard Buttons because now you can have the box behave differently when activated, depending on the current toggle state of the Checkbox. In Quorum, this is accomplished by calling the activation Behavior's Run action when toggled on and the Behavior's Dispose action when toggled off. For more information on these two actions, refer back to the Behavior tutorial.
For this tutorial, we will add a Checkbox to our game and set a Behavior for it to use as we start to activate it. To start, create a new Game application.
Making a Checkbox
In order to use Checkboxes, we must use the Checkbox Library with the following use statement:
use Libraries.Interface.Controls.Checkbox
Since we want the Checkbox to be usable as soon as the game begins, most of our code will be in the CreateGame action. To make the Checkbox, we simply declare it, give it a name, and position it. The name for a Checkbox is similar to the name of a Button, in that the text displayed by the Checkbox will be the same as the name. We will also change the font size to make the Button bigger, since the box will scale with the font size. Don't forget to add the box to the game, and we will also put focus on the Checkbox so that it is accessible and we can use the Space Key to activate it.
Checkbox box
box:SetName("My Checkbox")
box:SetPosition(200, 350)
box:SetFontSize(30)
SetFocus(box)
Add(box)
So far, the main class is as follows:
use Libraries.Game.Game
use Libraries.Interface.Controls.Checkbox
class Main is Game
action Main
StartGame()
end
action CreateGame
Checkbox box
box:SetPosition(200, 350)
box:SetName("My Checkbox")
box:SetFontSize(30)
SetFocus(box)
Add(box)
end
end
Now when you run the program, the Checkbox should be in the window and clicking the box or pressing the Spacebar will cause it to change toggle states. By default, when toggled on the Checkbox icon will change colors and a checkmark will appear inside the box. When toggled off the Checkbox icon will simply be gray and the checkmark will not be visible.
Checkmark Color
The Checkbox also has one little extra customization feature where you can change the color of the checkmark inside the box. This is purely for appearance but if you wish to change that you can accomplish it with these lines of code:
Color c
box:SetCheckColor(c:White())
Setting a Behavior
Like a standard Button we can set a Behavior to run when the Checkbox is activated, but a Checkbox has two states so two different actions that will be called when toggled on or off. Internally, what will happen is that when toggled on the Run action will be called and when toggled off the Dispose action is called.
To show this, we will create a new Quorum class for our Behavior and we name it "CheckBehavior.quorum." Our class will inherit from the Behavior class and we will override the Run and Dispose actions.
All we will the Behaviors actions do is say and output whether they we checked or unchecked. Run will output that the box was checked while Dispose will output that the box was unchecked. The full Behavior is shown in the following code block:
use Libraries.Interface.Behaviors.Behavior
use Libraries.Interface.Events.BehaviorEvent
use Libraries.Sound.Speech
use Libraries.Interface.Item
class CheckBehavior is Behavior
Item item = undefined
Speech speech
action Run(BehaviorEvent event)
item = event:GetItem()
speech:Say(item:GetName() + " is now checked")
output item:GetName() + " is now checked"
end
action Dispose()
speech:Say(item:GetName() + " is now unchecked")
output item:GetName() + " is now unchecked"
end
end
Now we simply need to go back to the main class and set a CheckBehavior to our Checkbox. This is done with the following lines of code:
CheckBehavior behavior
box:SetBehavior(behavior)
Now, when you run the program, activating the Checkbox will make the program say and output that the box was checked. Toggling the Checkbox off will make the program say and output that the box was unchecked.
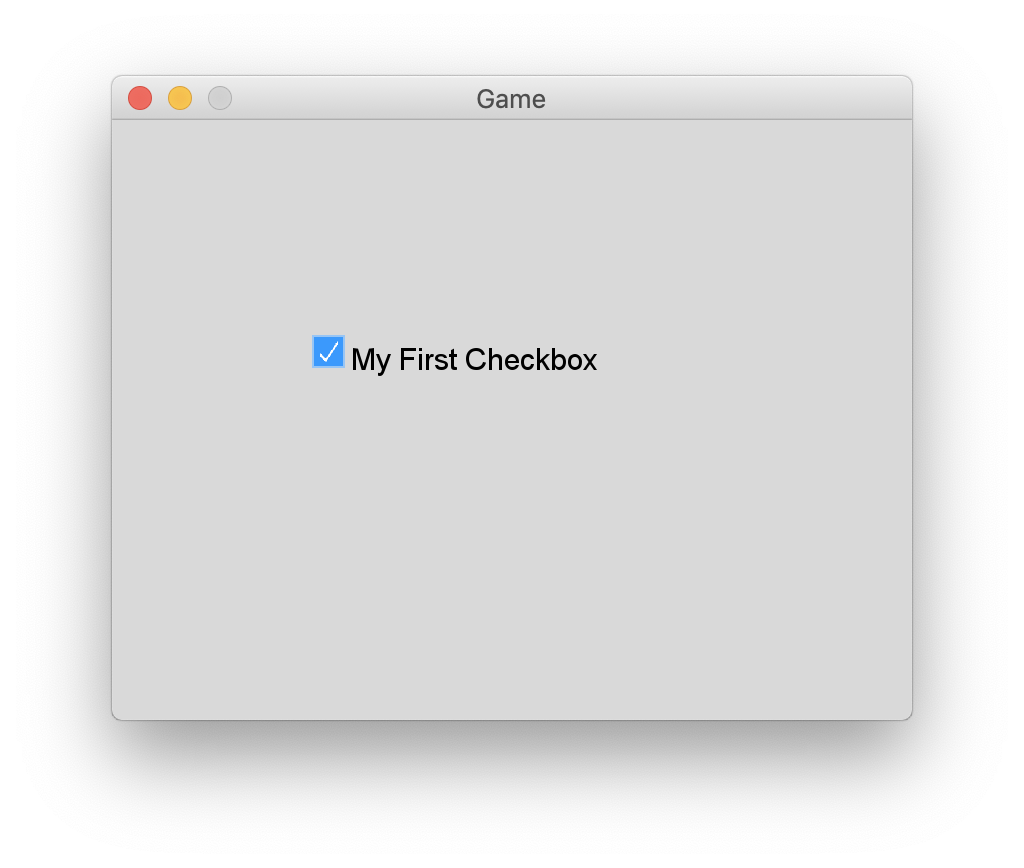
Run the Program
Next Tutorial
In the next tutorial, we will discuss Radio Buttons, which describes how to use Radio Buttons.