Tutorial: Dialog
This tutorial tells you how to use Dialogs in QuorumIntroduction to Dialogs in Quorum
In Quorum, a Dialog is subwindow inside of the main window that is used to carry a temporary information apart from the information in the main application. Many Dialogs present an error message or warning to a user, but Dialogs can present any UI component. A common Dialog is one with a message and then an OK or Yes/No Button that will close the Dialog.
Default Dialogs in Quorum have a top bar that is simply a bar of a set size with the name of the Dialog and then a Button to close the Dialog. Dialogs have an option to make this bar hidden, but for our example we will keep the top bar and we will make a panel with an Icon for the background and then a Button that with a Behavior that will close the Dialog.
To start, make a new Game Application project.
Making a Dialog
Quorum provides a Dialog in the Standard Library that you can use, but the default Dialog has two empty actions, OnShow and OnHide. These actions are called when the Dialog is opened or closed. What we will do is make our own Dialog that inherits from the default Dialog and we will override OnShow and OnHide. For more information on inheritance, see the tutorial on Inheritance.
To begin making our Dialog we will make a new Quorum class in our project and name it "MyDialog.quorum."
In order to make a Dialog, we need to include the Dialog Library. For this Dialog we will also use Buttons, Color, Icon, and Speech Libraries. The class is named MyDialog and it will inherit from Dialog. There will be Button and Speech member variables, and three actions: Setup, OnShow, and OnHide. The template for our Dialog is shown in the code block below:
use Libraries.Interface.Controls.Dialog
use Libraries.Interface.Controls.Button
use Libraries.Game.Graphics.Color
use Libraries.Interface.Controls.Icon
use Libraries.Sound.Speech
class MyDialog is Dialog
Button close
Speech speech
action Setup
end
action OnShow
end
action OnHide
end
end
In our Setup action we will Name our Dialog using SetName, and set its PixelWidth to 300. We will also set the Dialog to be modal. A modal Dialog prevents input from reaching other parts of the application while the Dialog is displayed. For example, clicking outside of the Dialog on a Button will not trigger the Button if the Dialog is modal. The code for this is shown below:
SetName("My Dialog")
SetPixelWidth(300)
SetModal(true)
For the rest of the Setup action we will setup our Icon, Button, and panel. Our Button doesn’t currently have a behavior, but that can be added later. Setting up the panel is the same as making a container which was covered in the Layout tutorial. The code for how we made our panel is given in the following code block:
Color c
Icon diaIcon
diaIcon:LoadFilledRectangle(300, 300, c:LightGray())
close:SetName("Close")
close:SetPosition(130, 140)
Control panel
panel:SetPercentageWidth(1)
panel:SetPixelHeight(300)
panel:Add(diaIcon)
panel:Add(close)
Add(panel)
Now we will add to our OnShow and OnHide actions. These actions come from the Dialog parent and they are called upon opening or closing the Dialog. This Dialog will say and output that the Dialog was opened or closed, although OnShow will have one extra action call. In OnShow we will call Focus on our Button. This is so the Game now focuses on something inside of our Dialog window. This not only allows for the Button to be accessible by keyboard but also now we can use the Keyboard to close the Dialog. By default the escape key will close a Dialog. Both actions are shown in the following code block:
action OnShow
close:Focus()
output GetName() + " opened"
speech:Say("opened")
end
action OnHide
output GetName() + " closed"
speech:Say("closed")
end
Opening a Dialog
Now we have a completed Dialog class, but there’s a problem, how do we open it? To open a Dialog you need to calls its Show action, but where do we call Show? A Dialog can open in a variety of ways but what we will do is in our main class, we will make a Button and we will give it a Behavior to open our Dialog. We will first make the behavior by making a new Quorum class named openDialog.quorum.
In the following code block is the full openDialog class. All the class has is an action that will set what Dialog it will open and a Run action that opens the Dialog.
use Libraries.Interface.Behaviors.Behavior
use Libraries.Interface.Events.BehaviorEvent
use Libraries.Interface.Controls.Dialog
class openDialog is Behavior
Dialog dialog
action SetDialog(Dialog newDialog)
dialog = newDialog
end
action Run(BehaviorEvent event)
dialog:Show()
end
end
You can also make a Behavior for our Dialog’s Button that will close the Dialog. It will be almost identical to this one but it will need a new name and it will call Hide instead of Show.
Finally, in our main class we will make our Dialog and the Button that will Show the Dialog. Remember that we setup our Dialog in the Setup action so we have to call it after we declare our Dialog. Also note that we will set the Focus to our open Button so it is accessible with the keyboard. The full main class is shown in the following code block:
use Libraries.Game.Game
use Libraries.Interface.Controls.Button
class Main is Game
action Main
StartGame()
end
action CreateGame
MyDialog dialog
dialog:Setup()
Button open
openDialog openBehavior
openBehavior:SetDialog(dialog)
open:SetName("Open Dialog")
open:SetBehavior(openBehavior)
open:SetPosition(325, 400)
SetFocus(open)
Add(open)
end
action Update(number seconds)
end
end
Now when we run the program their will a button that you can activate that opens our Dialog. When the Dialog is opened you can press the Escape key to close the Dialog, or if you added the closeDialog behavior you can close the Dialog by pressing Space to activate the Button. Note that you can also click the Buttons or the X on the Dialogs top bar.
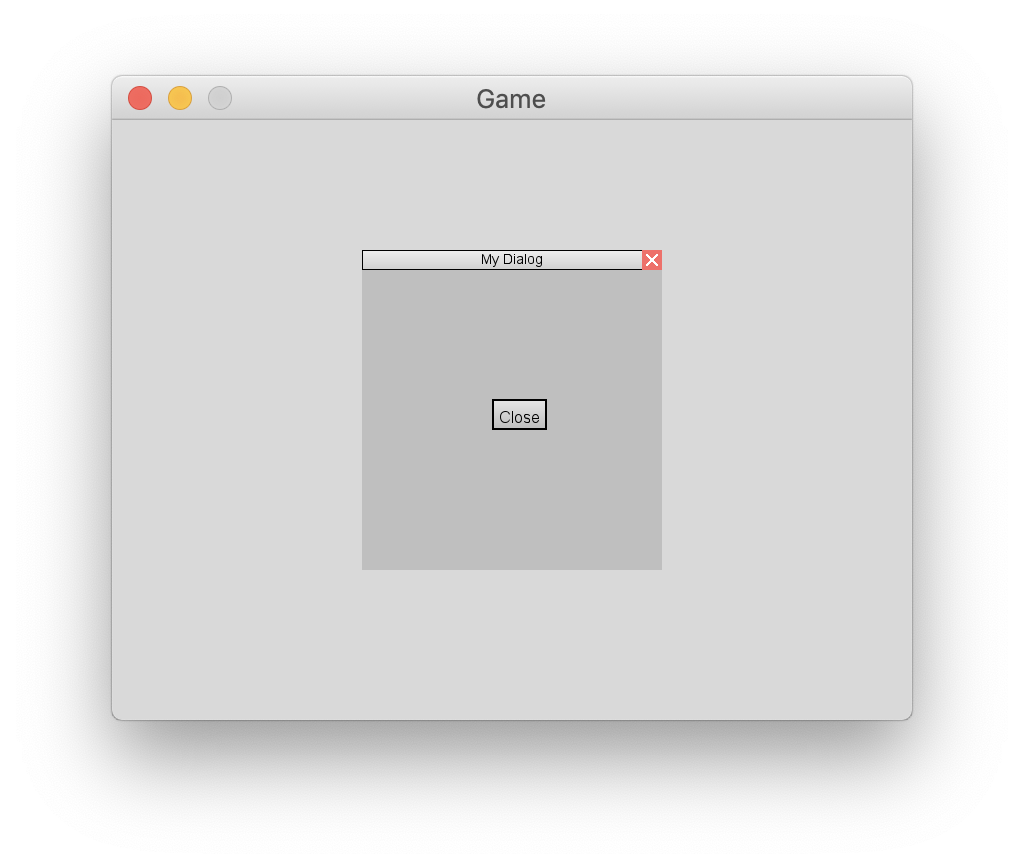
Try making a User Interface
Next Tutorial
In the next tutorial, we will discuss File Choosers, which describes how to use File Choosers.