Hour 12: Actions
This lesson is about learning to write your own actions.
Overview
In previous lessons, you have seen how to use objects from libraries and how to call actions from those objects. In this lesson, you will learn how to make your own. Learning how to write your own actions is an important first step in making your code more reusable.
Goals
You have the following goals for this lesson:
- Learn how to use action blocks to create an action
- Learn about the optional properties of actions: parameters and return values
- Discuss how scope impacts variables declared in and out of actions
Warm up
Actions allow programs the ability to perform a task. In the previous lesson, while you were working on Form apps, recall the kinds of actions you were calling:
page:AddBanner("banner1", "About me", "Welcome to my page")
page:AddButton("Click me")
Each of these calls is an action. What might the benefit be of learning to write your own action?
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Action | A named sequence of code that can be reused later in a program. |
Action Call | Code that tells an action to run. |
Argument | A declaration in the signature for an action saying a variable must be passed when calling. |
Parameter | A special variable inside an action that was provided to the action when it was called. |
Return Value | The result of an action that can be used like a variable. |
Scope | The area where a variable exists and can be used. |
Code
You will be using the following new pieces of code:
Quorum Code | Code | Explanation |
---|---|---|
action MyAction | action AddNumbers | Creates a function inside of Quorum |
action MyAction (type name) | action AddNumbers(integer a, integer b) | Creates an action that uses parameters, letting you pass in values from the main program to be used in the action. |
action myAction returns type | action AddNumbers(integer a, integer b) returns integer | Creates an action inside of Quorum that returns a value back once the function is finished. |
return value | return 0 | Placed in value returning action that sends back a value upon termination |
CSTA Standards
This lesson covers the following standards:
- 2-AP-14: Create procedures with parameters to organize code and make it easier to reuse.
- 3A-AP-17: Decompose problems into smaller components through systematic analysis, using constructs such as procedures, modules, and/or objects.
- 3A-AP-18: Create artifacts by using procedures within a program, combinations of data and procedures, or independent but interrelated programs.
Explore
When coding, it can quickly become tedious to rewrite the same or similar code. So far, you have learned how to shorten repetitive commands by using loops and conditionals using repeat and if. This lets you reuse code inside of the loop, but does little to let you reuse more advanced code.
You have already seen how useful and powerful code reuse can be when calling actions. You do not, for example, need to know how the Form class's actions work. You can just call them and hope that the person that wrote them did a reasonable job.
The term action has not been standardized. In fact, although the analogy in math that is closest, while not exactly the same, is that of the function, the field of computer science never standardized its terms. In programming languages, common terms for the same or similar concepts include function, procedure, method, action, or although used in somewhat esoteric circumstances: lambda. In Quorum, they are called actions because evidence in the academic literature suggests this name is reasonable [1]. The term implies what a piece of code should do and each action is given an individual name. These names can be used to help you organize your code. Regardless of the language, this concept of actions is a core feature of modern programming. It is critical and you cannot avoid it.
In colloquial words, an action is a chunk of code that has a name. Just like you saw when using objects before, you can run the code in an action by calling its name. Actions are especially useful because they allow you to write down some idea you have, then reuse that idea. They allow others to write down their ideas so that others can use them.
Here are a few of the benefits of using actions:
- Actions allow you to reuse code.
- Actions can abstract code, letting you focus on what task an action performs without worrying about how it does it.
- Actions can break up a program into smaller pieces, which can make it easier to divide responsibilities between different people on a team.
- Actions can allow others to tap into functionality that they might not know how to write themselves.
Consider an example from Math. While most with a formal education are comfortable with basic operators like addition and subtraction, advanced math can sometimes require considerable education, up to a doctorate, to write accurately into a computer program. The actions for many math functions are very complex and writing them is extremely hard. However, if written correctly, calling them is very easy.
Actions are so important that you have been using them in every program you have written so far, even if it was not obvious. When you write a Quorum program that does not include any actions, one is secretly generated behind the scenes for you. This secret action is called Main and it wraps around all the code you have written. The image below shows a program and what it secretly converts to under the hood. These two programs are functionally identical.
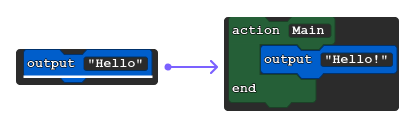
Although evidence in the literature is not definitive, it is at least plausible that Quorum's automatic hiding of this feature is beneficial for some [2]. Once you start to write more complex programs, though, you will need to identify where you want to start your program. This requires you to define your own Main function, which you can do with the following lines of code:
action Main
end
Any program that includes actions must have a Main action and a starting point for which one is the beginning of your program. While likely confusing, computer programs can have many starting points and the term Main is just a common convention. A sample program that includes a Main and another action would be the following:
action Main
SayGreeting()
end
action SayGreeting
say "Hello Friend!"
end
In this code example, the program starts at the Main action and runs each command in order until the program reaches the Main action's end. Consider how this program executes, step by step:
- The program always begins on the first line inside the Main action. In this case, it starts on line 2.
- The SayGreeting action is called. The program jumps to the first line inside the SayGreeting action, on line 5.
- The code on line 5 executes. The program outputs Hello Friend!
- The program advances to line 6. Because this is the end of the action, the program returns to where it was before the action was called, back to line 2.
- The program advances to line 3. Since this is the end of the Main action. There is no more code left to run, so the program terminates.
Actions and Arguments
Actions are closely tied to the concepts of arguments and parameters. An argument is a way of forcing the programming language to require special information is given to the action. A parameter is an actual piece of information passed to an action. The distinction is subtle, so consider an example.
action Test(integer value)
end
In this action, there is a single action with the name of Test. The first line of the action is called a signature and that signature has one argument. The argument is of type integer and has the name value. Actions can have as many arguments as is desired. They can also do things. This action would add two integers, a and b, and output the value to the screen.
action Add(integer a, integer b)
output a + b
end
Actions and Parameters
Arguments are special in that they tell the programming language to force the programmer to give the action information. That information is called parameters. These variables only exist in the scope of an action and their value is set to whatever value you use when you call the action.
Parameters provide a way to give input. This is useful when the action is intended to perform a task on something that could vary. For example, if your action performs math operations, you might need a parameter to specify what value you are performing math on.
The example below shows a program using an action with a parameter. When the code executes, it runs the PrintValue action twice. The first time, the provided parameter is 2, so the value variable is set to 2 inside of PrintValue. The second time it is run, the provided parameter is 4, so the value variable is set to 4.
action Main
PrintValue(2)
PrintValue(4)
end
action PrintValue(integer value)
output value
end
Actions can have multiple arguments and this would require the programmer to pass multiple parameters. To do so, you need to separate each parameter with a comma, like so:
action DefaultAction(type name1, type name2, type name3)
// code
end
Calling that action needs the same number of parameters, separated by commas and the computer will tell you there is an error if this is wrong. The order also matters. The first provided parameter always sets the first variable, the second parameter the second variable, and so on. In the example below, the passed parameters are 10 and 6. The output of the program is 4.
action Main
PrintValue(2)
PrintValue(4)
end
action PrintDifference(integer value1, integer value2)
output value1 - value2
end
Actions with Returns
Actions can also give back a value and this is called returning a value. Return values can be thought of as the result of an action. If an action has a return value, you can use it to set the value of a variable, output to the console, or use the returned value in basically any way that you could use a variable.
The code below shows an example using a return value. On line 2, the action is called, and its return value is assigned to the result variable. The return value itself is on line 6, using the return line. The return line indicates the result of the action.
Different programming languages manage actions and returns in different ways and memorizing them all is generally not helpful. Counterintuitively, evidence in the literature suggests that forcing programmers to declare the types of the parameters, and what they return, is helpful [3]. The reason why is easy to understand. If an action is saying, hey, you have to have this input and this output to be used correctly, then the programming can check everywhere across the entire piece of code you are writing and check for errors automatically. This is called static typing and can be very helpful.
In any case, the return type is a number in this case. This means the value in the return line must match the type in the action. When the program in the example runs, it gets the result from the action, assigns it to a variable, then outputs it. The output is 7. The point is that these types provide a constraint that you can set yourself. Here is the code, now with return values.
action Main
number result = GetLuckyNumber()
output result
end
action GetLuckyNumber returns number
return 7
end
Actions and Scope
In previous lessons, you have learned how multiple line blocks (like repeat and if blocks) have scope, which means that variables declared inside of them cannot be used outside of them. Action blocks also follow this rule, so variables declared inside an action block cannot be used outside of them.
Similarly, items inside of actions obtain the scope of their parent. So, a loop inside a conditional inside an action can use the variables inside the action. These scoping rules are essentially the same throughout Quorum. If a variable is inside of lines, or a block, other things in the same space can see it. If not, they cannot.
Citations
- Andreas Stefik and Susanna Siebert. 2013. An Empirical Investigation into Programming Language Syntax. ACM Transactions on Computing Education 13, 4, Article 19 (November 2013), 40 pages.
- C. L. Corritore and S. Wiedenbeck, Direction and scope of comprehension-related activities by procedural and object-oriented programmers: an empirical study, Proceedings IWPC 2000. 8th International Workshop on Program Comprehension, Limerick, Ireland, 2000, pp. 139-148, doi: 10.1109/WPC.2000.852488.
- Stefan Endrikat, Stefan Hanenberg, Romain Robbes, and Andreas Stefik. 2014. How do API documentation and static typing affect API usability?. In Proceedings of the 36th International Conference on Software Engineering (ICSE 2014). ACM, New York, NY, USA, 632-642.
Engage
While not exactly the same, actions are very similar to the concept of a function in math. The primary difference is that actions, since they are written in a programming language, often have quirky rules related to that language. Practicing writing actions, and learning the strange little rules, can help you understand how to break apart your programs in a way that is easier to follow.
Part of the reason you are thinking about actions is because this lesson is building up to writing what is called behaviors. If a user clicks a button in your app, you have to tell it what to do and actions are the way to do it.
Directions
In these problems, you will engage in scenarios where you need to break apart your program into collections of actions. Each action might do a different thing, like run a calculation, output something to the screen, talk, or give back a value.
Many operations are written as actions so that not every user of the programming language needs to reinvent the wheel. As before with these Parsons problems, you can drag and drop, use the keyboard, or even just write in the editor the solution to the problem and run the code. As a reminder, the hotkey to run the code is ALT + SHIFT + R on Windows and CTRL + SHIFT + R on Mac.
Wrap up
Imagine you are a software developer creating a new app. How would you go about using actions to break apart all the pieces of your app to make it easier to write or maintain?
Next Tutorial
In the next tutorial, we will discuss Classes Online, which describes how to organize your code with classes.