Hour 8: Loops
This lesson is to introduce you to loops.
Overview
Perhaps one of the most common aspects of programming is automation. Computers often excel not just at fast number crunching, but also repetition and decision making. In this lesson, you will explore how to tell computers to repeat themselves. There are many reasons for using repetition. It is a hallmark and fundamental skill in computer science.
Goals
You have the following goals for this lesson:
- Learn how to use repeat times statements
- Learn about repeat while and repeat until statements
- Discuss how scope impacts variables defined inside loops
Warm up
One of the benefits of computers is that they can do repetitive tasks that would be tedious or difficult for humans to do. What are some examples of repetitive tasks that technology can handle? What do you think the code for those kinds of tasks might look like?
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Loop | A structure that contains code to be run repeatedly. It runs a certain number of times, or so long as a condition is met. |
Multiple Line Block | A block that takes up more than one line in the editor, and has multiple parts that can be moved and adjusted. |
Scope | The area where a variable exists and can be used. |
Counter | A variable, often an integer, that is used to track progress in a loop over time. |
Code
You will be using the following new pieces of code:
Quorum Code | Code | Explanation |
---|---|---|
repeat INTEGER times | repeat 10 times end | Runs the code inside the repeat block INTEGER times. |
repeat while BOOLEAN | repeat while i < 10 end | Repeatedly runs the code inside the repeat block as long as the BOOLEAN value is true. |
repeat until BOOLEAN | repeat until i >= 10 end | Repeatedly runs the code inside the repeat block as long as the BOOLEAN value is false. |
end | repeat 8 times end | Marks the end of a multiple line block, like repeat blocks. Repeat blocks run code between the start of the block and the end. |
CSTA Standards
This lesson covers the following standards:
- 1B-AP-10: Create programs that include sequences, events, loops, and conditionals.
- 2AP-12: Design and iteratively develop programs that combine control structures, including nested loops and compound conditionals.
- 3A-DA-12: Create computational models that represent the relationships among different elements of data collected from a phenomenon or process.
Explore
When making programs, you often want to run code multiple times. Sometimes it is more convenient to run the same code over and over again instead of writing out duplicate code by hand. In other cases, you do not know ahead of time how many times the code will need to run. This can be used for a seemingly endless, pun intended, number of reasons, like counting, adding, or other operations.
In computer science, there are many ways to accomplish this and programming languages vary in how they describe it. In Quorum specifically, the word repeat is used to imply the concept in the computer of doing something over and over again. While the exact terms vary across the discipline, sometimes these structures are known as loops.
A loop is a section of code with a condition attached to it. That condition is usually either a number of times to run, such as 'do this code 10 times,' or it is a boolean value. If the condition is true, then the loop keeps going. If it is not, then the loop stops.
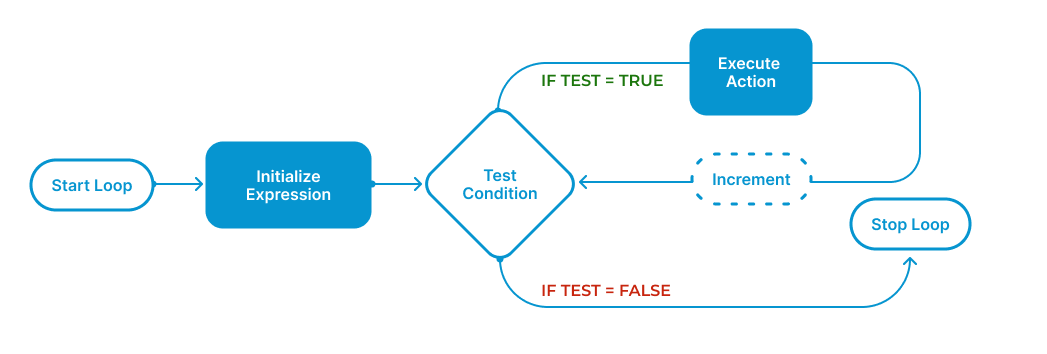
Repeating Yourself
Part of the purpose in doing Parsons problems is to minimize the amount of code a person notices, or in some cases has access to, while coding to assist in learning. In previous lessons, each problem had a bespoke list of blocks that were available, but previously these all represented one single line of code. All of this was real code that actually works in other contexts, but now there is a new concept in the tray to be introduced: multiple line blocks.
Besides taking up more than one line, the important part of these blocks is that other blocks can go inside of them, between the first and the last line. The image below shows an example of a repeat times block with an output block inside of it. In computer science, this is often called a scope. The basic idea is that things inside of a scope only execute under certain conditions. Consider what these three lines of code do.
Repeat blocks are a little different from the other kinds of blocks you have used so far. Previously, each block you have used has been a single line of code. These blocks have two parts on two different lines. There are three different kinds: the repeat times block, the repeat while block, and the repeat until block. In the tray, using multiple line blocks is the same as using single line blocks, except that both lines end up in the source code. Here is a visual example of a multiple line block for repeat times.
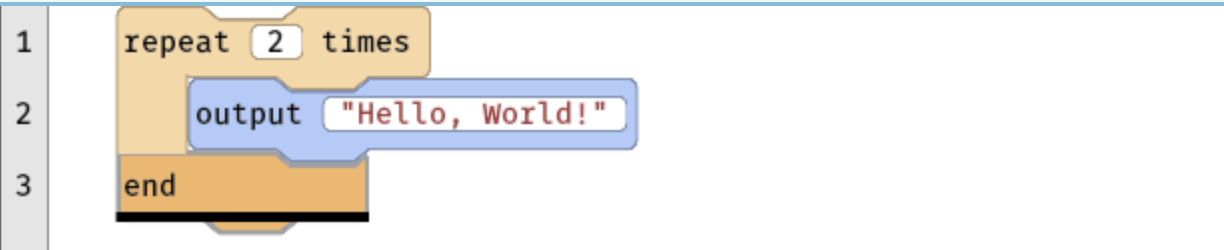
The repeat times block is the simplest, but least flexible, of the loops. While the word block is being used here, remember that every part of a block is actually just a line of code. So this is three lines of code. Blocks like this will run any code inside of it as many times as requested. In the program above, for example, the block will run its code two times. This means that the output block will run twice and thus put words on the screen twice.
In contrast, the repeat while block does not run a fixed number of times. Instead, it checks a boolean condition. If the condition is true, it runs the code. The example below shows some code using a repeat while block. This means it is very possible that this kind of loop will run forever.
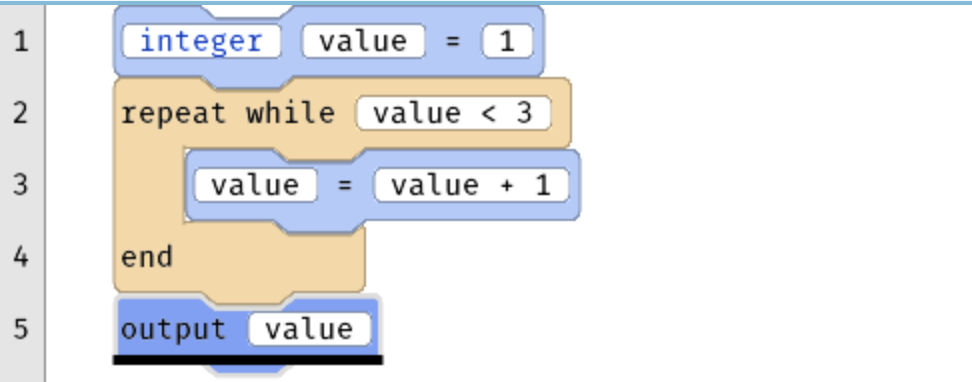
In this example, you start with a variable called value that starts at 1. When the code reaches the repeat while block, it checks the condition. Because 1 is less than 3, the code executes, and increases the value by 1. Then it checks the condition again. Since 2 is still less than 3, it runs again, and the value increases by 1 up to 3. When it checks the condition this time, 3 is not less than 3, so it stops. It then outputs the value, which is 3.
The repeat until block is the opposite of the repeat while block. The repeat while block will run if the condition is true, but the repeat until block runs if the condition is false. Otherwise, they work the same way. It is always possible to phrase the condition in a way that either block will work. There may be small advantages for one or the other, but generally which one to use is personal preference.
Scope in a Loop
When working with multiple line blocks, you can move blocks in or out of the multiple line block by dragging them with the mouse. Alternatively, you can select a block and use cut (CTRL + X on Windows or CMD + X on Mac) and paste (CTRL + V on Windows or CMD + V on Mac) with the keyboard. When pasting a block, the cut block will appear directly after the currently selected block.
Similarly, you can move blocks into the beginning of the repeat block. In fact, so long as the top part of the repeat block remains above the end portion, you can arrange code inside and outside of it however you would like, though the positioning of code will make a difference. The following code program will output the number two six times.
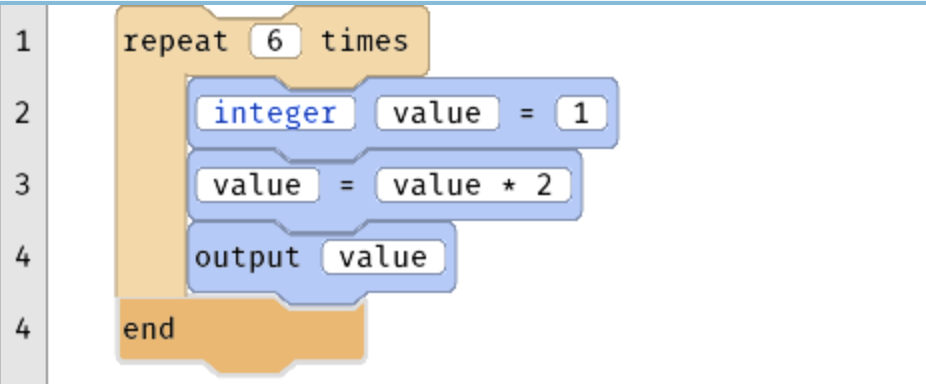
In the example above, the program should now output the number two 6 times. If you were to move the output back down again, out of the repeat block, it would be an error.
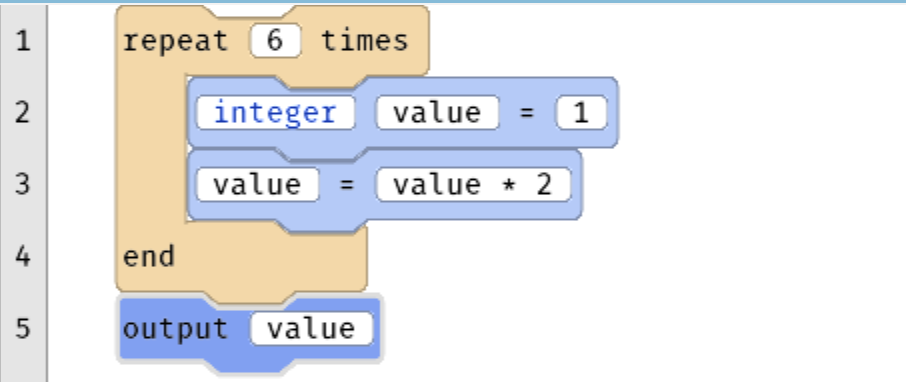
When a variable is created inside a multiple line block like a repeat block, it only exists inside that block. The output statement is now gives the following error:
I did not understand:
Line 5, Column 8: Could not find the variable value
The reason it gives this error is because, outside of the repeat statement, there is no variable with the name value available. This method for controlling variables and how programs run, scoping, is a common and critical to practice concept in computer science. It takes time to grasp, but is used in a very wide number of programs.
Engage
Now that you have spent some time learning about loops, it is time to try them yourself. The examples in these problems will have you practice a variety of concepts. This includes loop unrolling, which is practicing what a loop does without using them directly. This also includes familiar concepts like boolean expressions and finally the loops themselves.
Directions
In this example, you will be focusing on using loops and multiple line blocks. Like before, you can drag and drop, use the keyboard, or even just write in the editor the solution to the problem and run the code. Now with multiple line blocks, you can also adjust the beginning and ending of such connected blocks. As a reminder, the hotkey to run the code is ALT + SHIFT + R on Windows and CTRL + SHIFT + R on Mac.
Parsons Problems
Wrap up
Take a few minutes to consider the three kinds of loops. While it might seem on the surface important to teach or understand all three, which loop you choose is not a big deal. In fact, you can write any program using only repeat while or repeat until loops. Given that, what are the pros and cons of teaching students all three right when they are first learning the concept?
Next Tutorial
In the next tutorial, we will discuss Conditionals Online, which describes how to work with conditionals.