Hour 14: Create an Accessible App, Part 2: Behaviors
This lesson is to introduce you to behaviors in your user interface.
Overview
In a previous lesson, you created the interface for an app. While designing the user interface is an important part of the process, pretty apps that do nothing are not particularly useful. In this lesson, you will learn how to make an app respond when the user interacts with it. You do this by adding what is called a behavior.
Goals
You have the following goals for this lesson:
- Learn how to create a Behavior
- Add a Behavior to a Button in your app
- Think about programming in teams
Warm up
Many types of user interface types have behaviors. Buttons can be clicked. Textboxes can be typed in. Apps on tablets can use gestures like swipes, amongst other things. Speculate on how you think it might work to tell user interface items to do things like this.
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Pair Programming | A technique in software engineering where two programmers work together. |
Event Driven Programming | A style of programming where code runs in response to user input. |
Inheritance | A system where a child class gains the properties of a parent class. |
Behaviors | Inside a Form app, behaviors represent code that will run in response to an event occurring. |
Code
You will be using the following new pieces of code:
Quorum Code | Code | Explanation |
---|---|---|
use NAME | use Libraries.Interface.Controls.Button | A use statement for the Button object. A button is a selectable user interface component. |
Button buttonObject | Button button | Creates a button object on the form |
use NAME | use Libraries.Interface.Events.BehaviorEvent | A use statement for the BehaviorEvent object. A BehaviorEvent is sent to you when a button is clicked. |
use NAME | use Libraries.Interface.Forms.FormBehavior | A use statement for the FormBehavior object. FormBehavior is a special class for handling events. |
action Run(BehaviorEvent event) | action Run(BehaviorEvent event) | This action is the heart of a Behavior. It will run if a Button is clicked with this Behavior attached to it. |
GetForm() | Form form = GetForm() | Returns a Form object representing the form in the app. |
GetPage() | Page page = GetPage() | Returns a Page object representing the current page of the app. |
behaviorEvent:GetItem() | event:GetItem() | Identifies the element (perhaps a button) that triggered a Behavior. |
buttonObject:SetBehavior() | button:SetBehavior(behave) | Attaches a behavior to a button. When the button is clicked, the behavior runs. |
buttonObject:GetName() | text typeOfButton = button:GetName() | Retrieves the specific button name from the button click in order to perform a specific action |
CSTA Standards
This lesson covers the following standards:
- 3A-IC-27: Use tools and methods for collaboration on a project to increase connectivity of people in different cultures and career fields.
- 3A-AP-21: Evaluate and refine computational artifacts to make them more usable and accessible.
- 3A-AP-22: Design and develop computational artifacts working in team roles using collaborative tools.
Explore
You have learned a variety of different concepts and techniques, including how to store and modify data, how to use conditional statements to change what code is run, and how to write actions and classes. You also learned how to design the user interface of an app. Now it is time to bring all of your knowledge together to make an app respond to your input. The trick is a concept called behaviors.
Using Behaviors in the Form Library
Recall in your previous Form app where you learned how to create an app. You developed the user interface, adding text, a banner, and buttons. The app itself showed up visually and was accessible, but did nothing. If you pressed the button on the app, for example, it would visually show a small animation related to being clicked, but would not do anything in particular.
Telling your app to do things requires classes and actions, which is why these concepts need to come first. Once you have the basics of them, you can create your own behavior. Behaviors are special classes in Quorum that handle the actions your app takes when a user interacts with it. Behaviors are an example of event-driven programming, meaning code will run when users trigger an event. Behaviors have a special action called Run and this is where you write the code.
Behaviors work using a concept called inheritance. In computer science, the term inheritance means that a class gains the properties of a different class. The custom behaviors you will write for your apps will inherit from the FormBehavior class, which handles a lot of complexity for you. You do not need to know exactly how this class works. For now, you only need to know that inheritance will let you use the event handling functionality of Behaviors without having to write it all yourself. This is another example of abstraction.
You will need Behaviors to make your app respond to user input. Like any class, your custom Behavior class will need to go in a separate file from your Main form app. Consider the following example:
use Libraries.Interface.Events.BehaviorEvent
use Libraries.Interface.Forms.FormBehavior
class MyBehaviorNew is FormBehavior
action Run(BehaviorEvent event)
output "When you click me I perform an action!"
end
end
- Line 1: This line contains a use statement that gives you access to the BehaviorEvent library. BehaviorEvent objects include information about the event that caused the Behavior to run.
- Line 2: This line contains a use statement that gives you access to the FormBehavior library. Inheriting from the FormBehavior library will let your class respond to events.
- Line 3: This line creates a custom class that inherits from FormBehavior, using the is keyword. Your new behavior will gain the properties of the parent class it is inheriting from.
- Line 4: This line creates a new action. Importantly, the name must be Run, and it must take exactly one BehaviorEvent parameter. The class you are inheriting from expects an action with exactly this name and parameters to use when events occur.
- Line 5: When the action is run, it will output the text 'When you click me I perform an action.'
- Line 6: This line encloses the action block.
- Line 7: This line encloses the class block.
All behaviors follow the same code format, so if you have one Behavior written, you can reuse it as a template when you want to add more functionality to your apps. The only parts you need to change are the class name and the code inside the Run action. The idea is that any button you write will use behaviors in the same way.
FormBehavior
The previous Behavior that you wrote inherits from FormBehavior. This is a class in the standard library designed to make it easier for you to know what Page and Form your button or other control is interacting with. Examine this class from the standard library for a moment:
package Libraries.Interface.Forms
use Libraries.Game.GameStateManager
use Libraries.Game.Game
use Libraries.Interface.Behaviors.Behavior
use Libraries.Interface.Events.BehaviorEvent
class FormBehavior is Behavior
action GetForm returns Form
GameStateManager manager
Game game = manager:GetGame()
Form form = cast(Form, game)
return form
end
action GetPage returns Page
Form form = GetForm()
if form not= undefined
return form:GetCurrentPage()
end
return undefined
end
action IsFinished returns boolean
return true
end
end
Notice that the class has three actions, GetForm, GetPage, and IsFinished. In fact, it has more than this because FormBehavior itself inherits from Behavior. In this case, creating a FormBehavior allows you to customize what a button, or another control would do. It also means that if your button is on a page or on a form, it can access that information. This provides a lot of options.
All of the various things you can do with custom behaviors is outside of scope. However, to give a few examples, you can control animation, switch pages in forms, trigger behaviors at certain times, and many other things. The simplest example is just to have a button take an action when clicked or used. Because you used the word is and told the system your class is a FormBehavior, you get all this without having to know how it all works.
Creating an App with Behaviors
Examine the example below. It shows the Main file of a Form program, which includes two buttons named Hi and Bye. You have already seen how to create an interface using Forms before, but there are a few important additions on lines 8 to 14.
First, when the buttons are created on lines 8 and 12, they are now assigned to variables. Since you need to store the Button objects, you also need a use statement for them on line 3, use Libraries.Interface.Controls.Button. Second, on lines 9, 10, 13, and 14, the program creates new MyBehavior objects, and then associates those behaviors with the buttons. Now, when a button is clicked, the behavior will run.
use Libraries.Interface.Forms.Form
use Libraries.Interface.Forms.Page
use Libraries.Interface.Controls.Button
Form form
Page page = form:GetMainPage()
Button button1 = page:AddButton("Hi")
MyBehavior hiBehavior
button1:SetBehavior(hiBehavior)
Button button2 = page:AddButton("Bye")
MyBehavior byeBehavior
button2:SetBehavior(byeBehavior)
form:Display()
Now, examine the code for the MyBehavior class:
use Libraries.Interface.Events.BehaviorEvent
use Libraries.Interface.Forms.FormBehavior
use Libraries.Interface.Forms.Form
use Libraries.Interface.Forms.Page
use Libraries.Interface.Item
class MyBehavior is FormBehavior
action Run(BehaviorEvent event)
Form form = GetForm()
Page page = GetPage()
// retrieve the specific action from our button click
Item button = event:GetItem()
text typeOfButton = button:GetName()
// ADD CODE HERE
end
end
The code in this example is a template, and is incomplete, marked by the ADD CODE HERE comment at the end of the action. Still, there are several important things happening in the Run action.
First, the Run action uses the GetForm and GetPage actions. These return the objects that represent the Form and the currently displayed Page, respectively. These are helpful if you need to get or modify any values in the app or in the user interface.
Second, the action uses GetItem from the BehaviorEvent parameter. The BehaviorEvent is a special object that contains information about the input that triggered your behavior. In this case, GetItem returns an object representing the interface element triggered the behavior. Here, this will be your Button.
The action also gets the button’s name and stores it in a variable. A button’s name will be the text that you provided when you made it in the Form. In the current example, because there are only two buttons, the name will either be Hi if the first button was clicked, or Bye if the second button was clicked. You can use this information to decide how your behavior should react, depending on which button it is. The end of the template, with the comment that reads ADD CODE HERE, is where you will add more code to determine what your behavior actually does.
Code Templates
Here is another set of templates for a form and its behavior file. Again, copy pasting this code turns it into blocks automatically and vice versa. If the name of the button was Hi, it would then output hello.
Main.quorum
use Libraries.Interface.Forms.Form
use Libraries.Interface.Forms.Page
use Libraries.Interface.Controls.Button
Form form
MyBehavior behave
Page page = form:GetMainPage()
Button button = page:AddButton("Hi")
button:SetBehavior(behave)
form:Display()
MyBehavior.quorum
use Libraries.Interface.Events.BehaviorEvent
use Libraries.Interface.Forms.FormBehavior
use Libraries.Interface.Forms.Form
use Libraries.Interface.Forms.Page
use Libraries.Interface.Item
class MyBehavior is FormBehavior
action Run(BehaviorEvent event)
Form form = GetForm()
Page page = GetPage()
// retrieve the specific action from our button click
Item button = event:GetItem()
text typeOfButton = button:GetName()
// ADD CODE HERE
if typeOfButton = "Hi"
output "Hello"
end
end
end
Code Templates
As code becomes more complex, collaborating with others can be helpful. When dealing with commercial software, there are often large teams of software engineers that work together on the same project. This often means splitting up work across multiple people, but team members are also often encouraged to collaborate and work together to solve difficult problems.
A practice that is common among software engineers is a collaboration method known as pair programming. To Pair Program means that two programmers work together on a single computer. It is a similar practice in learning how to drive: one person writes the code (referred as the driver) and the other person reviews the teammate’s code and offers feedback (referred as the navigator).
Engage
Previously, you designed the interface of an app, but it was not yet interactive. Now, you will work together with a partner to make apps that can be controlled by a user. Specifically, you will first create a lightswitch app and then move to a thermostat app. The approach for this lesson is the same as behavior, meaning you will create the apps step by step in Parsons problems.
Directions
You are an app developer and you want to build some smart products that allow you to control a lightswitch or thermostat from your phone. You have created the look and feel for the app, but when you try to interact with the buttons, they do not do anything yet. Your next job is to examine the existing code with a partner and make the apps work.
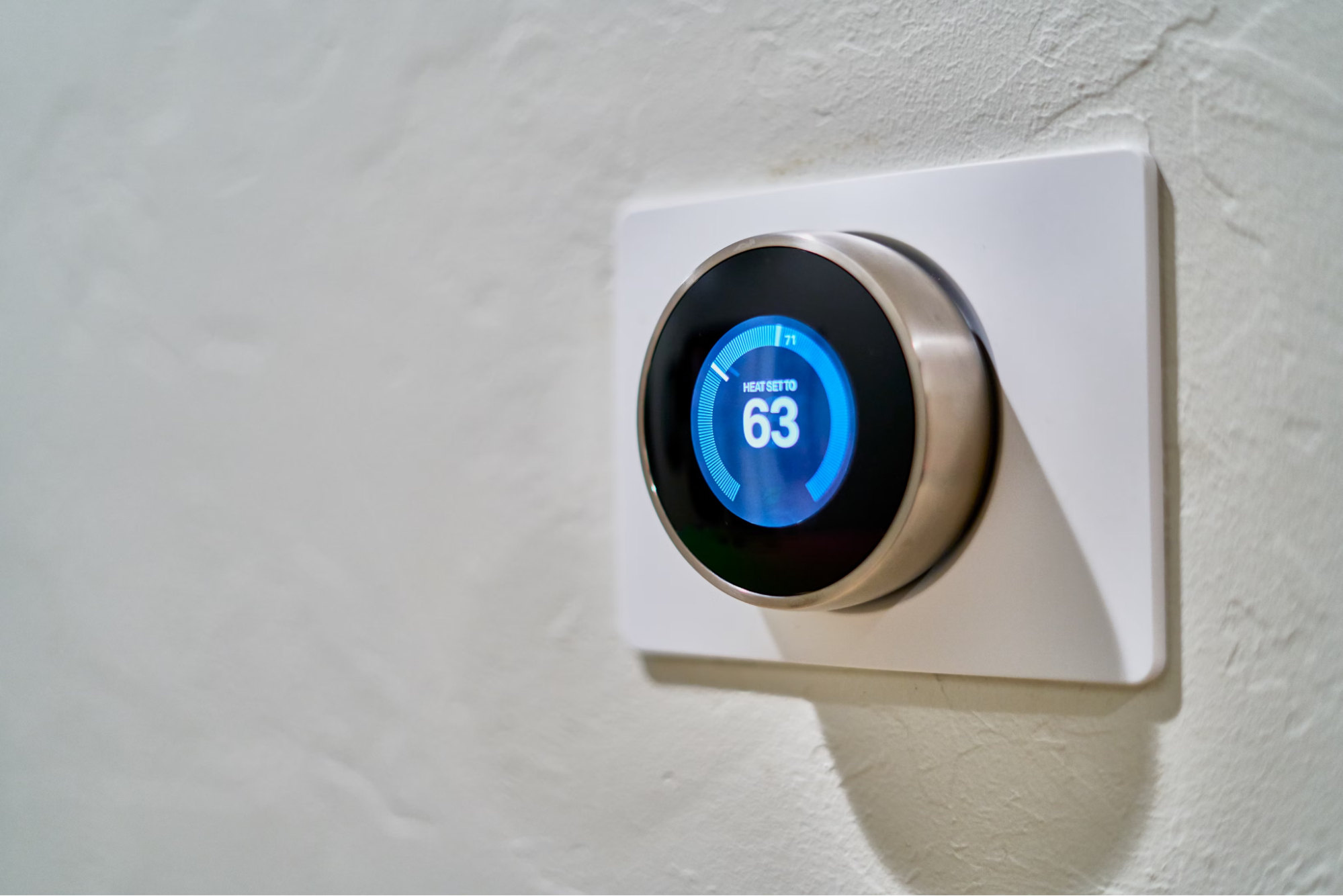
For these problems, you will be working through parts of the lightswitch and thermostat code one piece at a time. As before with these Parsons problems, you can drag and drop, use the keyboard, or even just write in the editor the solution to the problem and run the code. As a reminder, the hotkey to run the code is ALT + SHIFT + R on Windows and CTRL + SHIFT + R on Mac.
Wrap up
This completes the chapter of learning block-based Quorum Language online. Consider reflecting on the topics that you feel that are the most important. Put another way, computer science is a vast discipline with many things to be learned. Like other disciplines, though, some concepts are crucial to understand and others less so. Think through what seems important and what can be brushed aside in your context.
End of Lesson
You have reached the end of the lesson for Create an Accessible App, Part 2: Behaviors Online. To view more tutorials, press the button below or you can go back to the previous lesson pages.