Hour 3: Integer and Number Variables
This lesson is to teach you about integer and number values.
Overview
When writing programs, you give the computer a set of instructions to perform. As part of these instructions, you can give it specific data, called variables, at each step. When you hear the term variables, you might relate this topic to saving information. Your computer might save items you download, data you are keeping track of, or information about how you login to a website. In this lesson, you will learn about how to store information in a program using the two remaining variable types you have not yet used: integers and numbers. You will also learn how to use operators for these types and combine them to teach the computer to do math for you.
Goals
You have the following goals for this lesson:
- Learn how to store information with variables
- Learn about blank block templates
- Use numbers and integers
- Use operators to modify variables
Warm up
Consider a few examples of technology in your life. For each of the examples below, what kinds of data do you think are being stored or changed?
- Calculator
- Car speedometer
- Digital light switch
- e-Book
- Microwave
Can you think of other examples of technology that needs to store and change numerical data?
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Variable | A variable is a storage container. It has a name, a data type, and a value. |
integer | Integers are whole numbers. They can never have a decimal point. |
number | A number is a numerical value with a decimal point. |
Primitive Variable | A primitive variable is a variable that stores one of the four basic types of information: integers, numbers, booleans, or text. |
Operator | An operator is a symbol that changes or combines pieces of data. The most common operators are math symbols like +, -, *, /, or mod. |
Code
You will be using the following new pieces of code:
Quorum Code | Code | Explanation |
---|---|---|
integer NAME = VALUE | integer a = 0 | Creates a new variable called NAME that can store integers, and puts VALUE in the container. |
number NAME = VALUE | number b = 1.8 | Creates a new variable called NAME that can store numbers, and puts VALUE in the container. |
CSTA Standards
This lesson covers the following standards:
- 1A-DA-05: Store, copy, search, retrieve, modify, and delete information using a computing device and define the information stored as data.
- 3A-CS-02: Create clearly named variables that represent different data types and perform operations on their values.
- 3A-DA-09: Translate between different bit representations of real-world phenomena, such as characters, numbers, and images.
- 3A-DA-10: Evaluate the tradeoffs in how data elements are organized and where data is stored.
Explore
In this and the previous lesson, you have added on to the use of code like 'output' or 'say' and moved into storing information with variables. You have learned specific words you can use to do this, which before was boolean and text. In this lesson, you will expand on the idea of variables and use two new types: integer and number. Before doing so, consider that variables are fundamental to learning computer science. Consider again what variables are.
A variable is a container for information. You can think of it like a box. The box has a label on it that describes what can go inside. The box also has a name written on it, so you can tell different boxes apart. This name works kind of like a shape where not all shapes can fit in all boxes.
There are several blocks on the left side for variables. To start, think about one:

The above is exactly, mathematically, identical to the line of code:
integer a = 0
While the block initially provides no information, from left to right, the code and the block represent the type, the name, and the value. This is the same as before. However, starting in Quorum 13 and above, the blocks can also remind you what each piece is. To get these values, you can press tab to move the cursor inside one of the fields. Alternatively, you can use the mouse and click inside. When you do so, the visuals of the blocks change:

To describe in more detail, the block creates a variable that has four parts. The first box reads “integer”, which describes the type of information that can be stored in the variable. The second box reads 'a,' which is the variable’s name. After the second box is an equal sign, which indicates assignment (a fancy term for putting something in the variable box). The last box reads '0,' which is the value of the variable, or in other words, the data being put in the box. If the text were discussed, the concepts and meaning would not change. For screen reader users, note that this type, name, value terminology is embedded into the screen reader cues, but varies slightly depending on platform and which screen reader is used. In the image above it is also displayed, which only occurs if the cursor is in a field and not the block. Integers are whole numbers without a decimal point.
Variable Types
The first box of your variable block describes the type of the variable. You have now used four different types of variables:
- Integers are whole numbers. They can be positive or negative and they never have a decimal point.
- Numbers are numerical values with a decimal point.
- Text is any sequence of text, including letters, numbers, special characters, etc. To indicate that something is text information instead of code, text is wrapped in quotation marks.
- Booleans are data with exactly two possible values: true or false.
These four data types are the building blocks of data and sometimes called primitive variables. The reason is a bit complex, but has to do with how the computer stores certain kinds of data in memory as a program executes. It is also somewhat misleading, because text always has somewhat complex storage rules in most programming languages. In any case, these are at least common types. The following shows a larger example of using several types of variables:
integer wholeNumber = 0
number decimalNumber = 0.0
boolean myBooleanValue = true
text greetings = "words"
say wholeNumber
say decimalNumber
say myBooleanValue
say greetings
Blank Block Templates
In the previous lesson, you discussed blank blocks. Inside of them, you can type normal code. Beginners may not know how to type this code, which means they may favor the tray and this is okay. The same code is just being learned in a different way. Professionals may already have the language memorized and thus might ignore the tray and this is also okay. Blank blocks do not, however, require that you have everything memorized. They are smart enough to guess what you probably mean as you are typing. The use of this feature is optional, as is this section, but is called blank block templates and can be very convenient as you learn. These are pre-programmed partial lines of code that can flesh out your code without having to know the nuances. The basic idea is that even if you cannot remember an entire line of code, if you can remember part of it, the system may be able to guess for you.
In addition to typing out full lines of code into a blank block, you can thus use templates. These templates provide yet one more optional mechanism by which you can learn to code. The idea is you can type a short mnemonic and the block system will flesh out an entire line. Almost always, because you are typing code that is not a full line, this would be an error if you ran the code. For example, in the very first Parsons problem for this lesson, if you typed integer into a blank block, it would be like so:
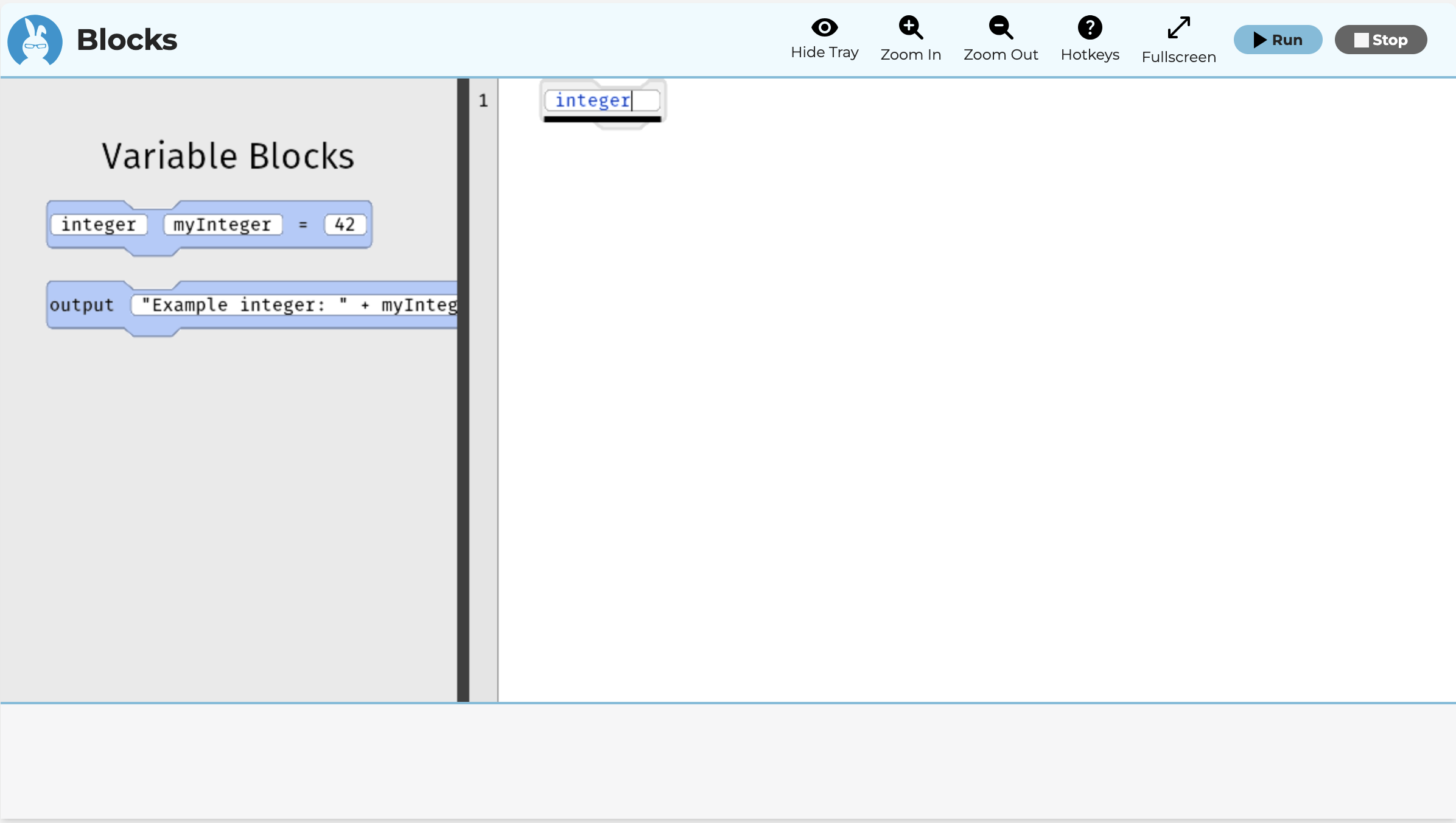
If you press enter, even though you have not completed a full line, the system will fill this out for you. This would be like so:
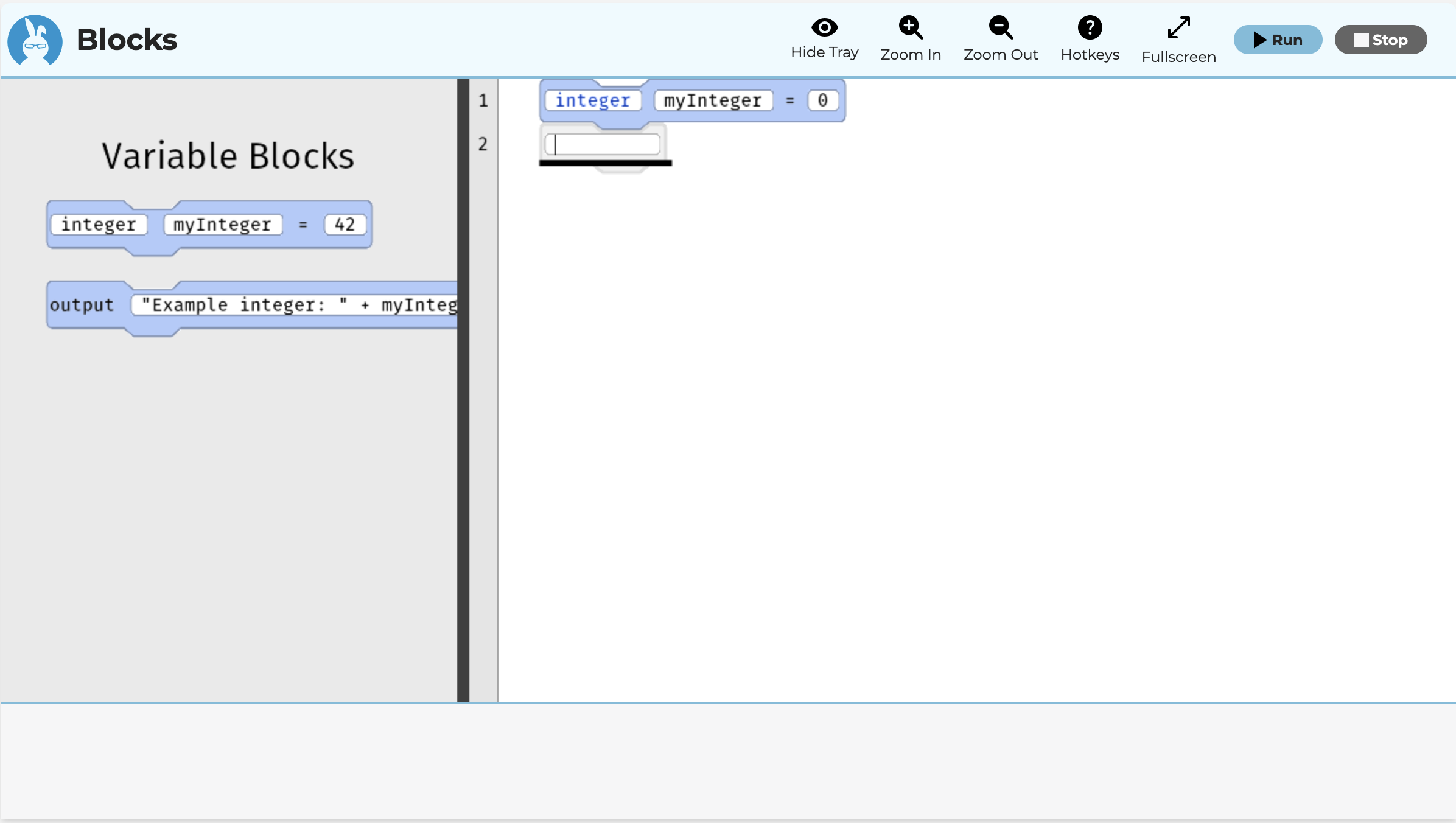
There are many templates that can be used in the system. Tinkering is encouraged, but memorization is not necessary.
Basic Autocomplete Templates
These are basic autocomplete templates that can be used to output to the screen, get input from the user, talk, or define variables. Thus, typing a single word will flesh out a line of code. This is optional, but convenient for learners or experts alike.
Template | Purpose |
---|---|
output | Output to the console |
input | Get information from the console |
say | Talk to the user through the speaker |
integer | Define a variable whole number without a decimal point |
number | Define a variable with a decimal point |
boolean | Define a variable that can only be true or false |
text | Define a variable with characters in it |
Control Autocomplete Templates
These autocomplete templates are different in that they may sometimes have a condition attached. For example, the template repeat (condition) times might be typed as repeat 5 times, which would create a loop that does what the name implies. Tinkering is part of the point with features like these.
Template | Purpose |
---|---|
if | Control the flow of a program by making decisions |
if (condition) | Control the flow with an optional condition |
elseif | Control a second part of an if statement |
elseif (condition) | Control the second part with a condition |
else | Control a fallback part of an if statement |
repeat | Cause the computer to do something over and over |
repeat while | Use a particular kind of loop that executes with a condition |
repeat while (condition) | Same as repeat while, but specifying the condition |
repeat until | Similar to repeat while, except the condition is reversed |
repeat until (condition) | The same as repeat until, but with the condition specified |
repeat (integer) | Cause the computer to repeat a fixed integer number of times |
repeat (integer) times | Same command as before except the word times is used |
check | A rarely used command to check for errors |
detect | A rarely used command used to detect certain kinds of errors |
always | An even more rarely used commands that guarantees some code executes even if there are errors |
Structure Autocomplete Templates
These final autocomplete templates are rather advanced, but it is documented here for completeness. They are used for adjusting higher level structures in more complex computer programs.
Template | Purpose |
---|---|
action | This generates a default action with a default name |
action (full line) | Similar to action, except that you can type a full line of code and the system will respond |
blueprint | Create a placeholder action |
system | Create an action that requires a corresponding plugin. This is an extremely advanced feature and should only be used if careful |
on | Create a constructor |
on create | Create a constructor by typing the full name |
return | Create something to give back from an action |
class | This constructs a class |
shared | An advanced feature that creates a shared class, which the system guarantees there is only one copy of across a program |
Operators
While programming, you often need to use math to run calculations. Fortunately, the operators people learn are largely very similar to what programming languages do. The kind of operators you can use, and what they do depends on the data type.
The operators described here all work in the same way. One value is placed on the left, another on the right, and an operator is placed in the middle. You already saw some operators with the use of and and or with booleans or + with text values. For integers, consider that 1 + 2 is a mathematical expression with the plus operator placed between the integers 1 and 2. The expression calculates how you might expect with an answer of 3.
For integers and numbers, you can use the five basic operations of math: addition (+), subtraction (-), multiplication (*), division (/). Integers also have modulus (mod), which calculates the remainder after integer division.
In the problems for this lesson, you will do operations like changing the values of your integer and number variables or making math expressions in each using operators to calculate a new value. It might be as follows:
integer wholeNumber = 7 + 5
number decimalNumber = 10 / 4
Perhaps the trickiest detail to understand about integers and numbers is how they manage their answers. With integer values, since they do not have decimal points, an equation like 5 / 4 would have a result of 1, not 1.25, and a remainder (called mod) of 1. This might sound like a mistake, but being able to obtain the number of times the divisor goes into the numerator is important in computer science for many kinds of apps. Chopping off the end of the value here is sometimes called truncating. Another word for the same idea is taking the floor.
With numbers, the situation might sound simpler, because 5.0 / 4.0 gives back 1.25. However, the problem is that numbers store information very differently than integers do under the hood and different apps might need one or another. As you practice, you will learn that sometimes even comparing numbers can be tricky and different programming languages do not always follow the same standards. The point is that it might sound like a slam dunk that one of these two types is easier to understand than another from a certain point of view, but in practice you need both for many reasons. Thus, learning about integers and numbers, and their quirks, is a skill that any learner should practice.
Engage
In this first part of the lesson, you will continue using Parsons problems. This time, you will focus on two big ideas, which are integer and number primitives.
Like before, the broad idea behind problems like these is to limit the number of programming options while learning, emphasizing where lines of code go in a program. In these problems, you will focus on practicing with these two types and using them to write calculations. Be sure to understand the differences between the two types, especially how they manage division and remainders.
Directions
In this case, like before there are three sets of Parsons problems. In this case, the first is on integers and the second is on numbers. As these two types are truly crucial to understand in computer science, both are recommended. In each case, you can drag and drop, use the keyboard, or even just write in the editor the solution to the problem and run the code. As a reminder, the hotkey to run the code is ALT + SHIFT + R on Windows and CTRL + SHIFT + R on Mac.
Wrap up
Once you are finished with your program, discuss the apps you think might require the use of integers and numbers. Can you think of any areas of study that would require one or the other and why?
Next Tutorial
In the next tutorial, we will discuss Libraries and math Online, which describes how to manage libraries and math.