Hour 9: Conditionals
This lesson is to teach you about conditionals.Overview
So far, you have made programs that are sequential, meaning they always follow the same set of instructions from start to finish. In practice, programs are rarely so linear. Often, you need to do different things depending on different circumstances. In this lesson, you will learn how you can use conditional statements to change what code you run.
Goals
You have the following goals for this lesson:
- Learn how to use "if" statements to control program execution
- Learn how to further control code with "else" and "elseif" blocks
- Learn how "scope" affects variables created inside "if" statements
Warm up
Traveling somewhere new can be fun, but it can also require planning. When you plan for a vacation or other travel, what are some factors that you consider when you start to pack your suitcase? How do you decide what to bring? Now, consider your decision making process. Can you formalize your decision making into rules (e.g. if X, then I will bring Y)?
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Conditional Statements | Block programming is a style where visual blocks represent pieces of the computer code that can be manipulated through various forms of user input. |
Boolean Expression | An expression in programming that evaluates to either true or false. |
Conditional | An expression a program will check if true or false before proceeding to an action |
Code
You will be using the following new pieces of code:
Quorum Code | Code Example | Explanation |
---|---|---|
if (condition) end | if weather = "sunny" //execute A end | The if statement is a conditional statement. If the program evaluates the condition to be true, it will run the block of code contained inside. |
elseif | if weather = "sunny" // execute A elseif weather = "snowy" // execute B end | The elseif block is a conditional that is only checked if the previous conditions in the structure were false. If the previous conditions were false and the elseif’s condition is true, it will execute the code contained within the elseif. |
else | if weather = "sunny" // execute A elseif weather = "snowy" // execute B else // execute C end | This is the default case in a chained if statement. This code will always run if all another above if statements evaluate to false |
CSTA Standards
This lesson covers the following standards:
- 3A-DA-10: Evaluate the tradeoffs in how data elements are organized and where data is stored.
Explore
In previous lessons, you have worked with using an if statement to get started with learning about the different coding structures. In this lesson, you will learn how and why to use them. If statements are the key to making complex programs, as they represent a program’s ability to make decisions.
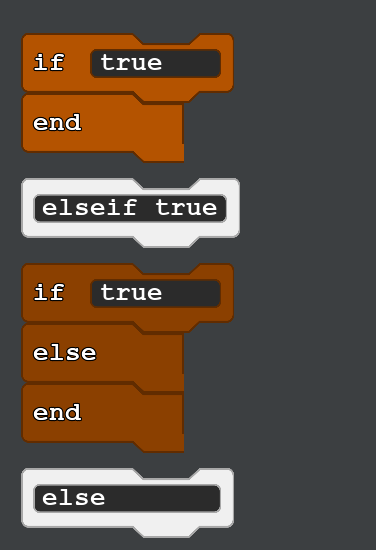
Imagine that you are playing a simple video game. For example, perhaps you are facing an enemy and you have two decisions of what your next move would be: fight or flight. You could decide to formalize your decision making process with some rules. It might look something like this:
if creature = “monster”
if health >= 50
output “time to attack!”
fightXP = fightXP + 1
else
output “run away!”
end
end
Using these rules, you can determine whether you have encountered a monster and whether you have enough health. Otherwise, you choose to run away. Just like you can formalize rules for your decision making, you can also make rules for the computer to follow. If statements allow you to control what code the computer executes depending on the rules or conditions you set.
There are 3 major parts of a conditional: the
- if statement (plus conditional),
- 0 or more elseif statements (plus conditional), and then an
- optional else statement.
Quorum Code | Code | Explanation |
---|---|---|
if condition end | if true output "hello world!" end | If there is only one action being tested, it will run the command if condition is true |
condition | integer score = 87 score >= 90 | Typically a boolean statement used to test a condition and will execute code if condition is met with true |
if condition elseif condition end | integer score = 87 if score >= 90 output "excellent work!" elseif score >= 80 output "good job!" end | If there are two conditions one action being tested, it will run the command if initial condition is true, if false, it will test the next condition |
if condition elseif condition else end | integer score = 87 if score >= 90 output "excellent work!" elseif score >= 80 output "good job!" else output "keep practicing!" end | If there are two conditions, one action being tested, it will run the command if the initial condition is true, if false, it will test the next condition. If the 2nd test is false, it will run a default case (the else). |
Creating a Conditional Statement
Creating a conditional statement involves using the "if" structure to define actions based on certain conditions.
In programming, the actual code for conditional statements varies between languages. For instance, in Quorum, it would look like this:
if conditional
// perform action A
else
// perform action B
end
When you think about how to write a conditional, it is often broken down into decision making where event A happens if the condition is true and event B happens if the condition is false.
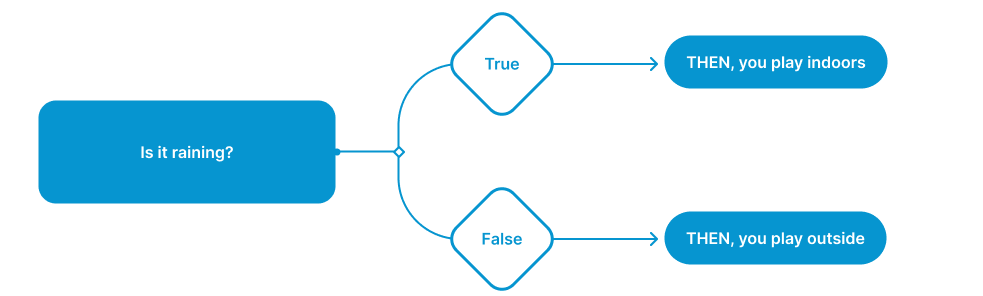
The "condition" typically is a boolean statement that will run either true or false. These conditionals again use any or all of the following boolean operators:
- Equality (=): Checks if two values are equal.
- Inequality (not=): Checks if two values are not equal.
- Greater than (>): Checks if one value is greater than another.
- Less than (<): Checks if one value is less than another.
- Greater than or equal to (>=): Checks if one value is greater than or equal to another.
- Less than or equal to (<=): Checks if one value is less than or equal to another.
Additionally, there are logical operators that are often used with conditional operators to combine multiple conditions:
- Logical AND (and): True if both conditions are true.
- Logical OR (or): True if at least one of the conditions is true.
- Logical NOT (not): Inverts the truth value of a condition.
You can use different types of variables with these conditional operators. For example: "5 < 10" would result in true and "4 < 10 and 7 >= 15" would result in false.
Nested Conditionals and "elseif"
It is possible (and sometimes useful) to place conditionals inside of other conditionals. Be careful when nesting them. Doing so is commonplace in programming, but some discretion to keep your code from getting confusing is warranted.
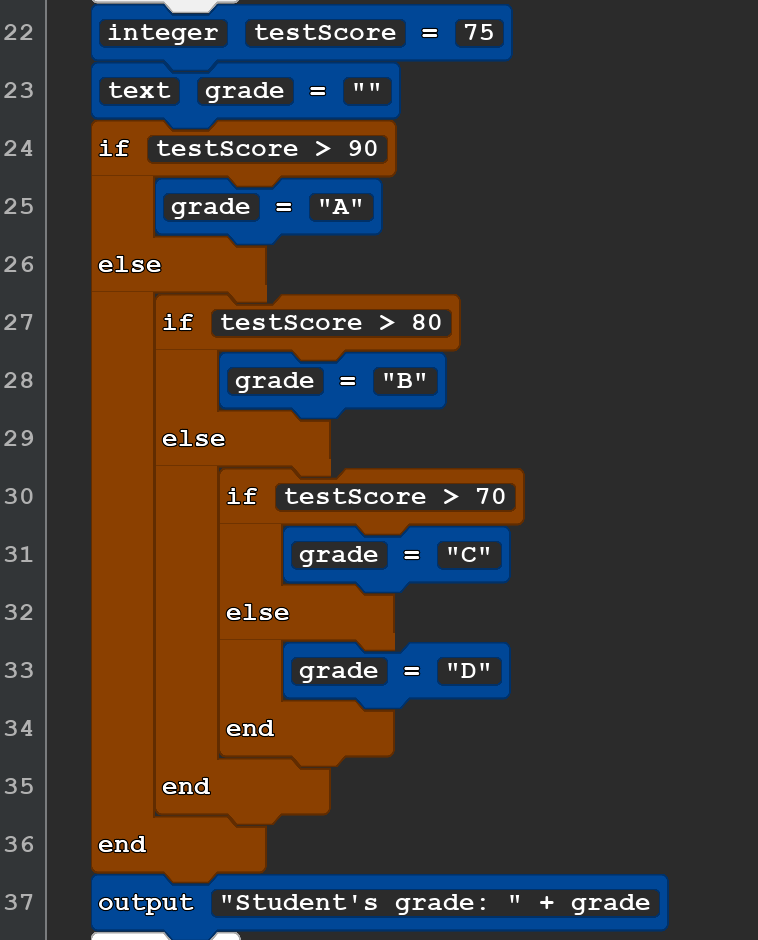
In this example, you have to think more carefully about what is going on in this piece of code because the testScores are so nested. In this example:
- If the testScore is greater than 90, it gets an "A."
- Otherwise, it checks if the score is greater than 80 for a "B."
- If not, it looks at scores above 70 for a "C."
- Finally, anything below 70 gets a "D."
Scoping can be determined multimodally. Visually, some multi-line blocks are inside of each other and shoved to the right. This means they are self-contained in that region. Aurally, for screen reader users, although screen readers vary slightly in what they read, they tend to say first the line of code, in addition to the nesting level and the number of top level blocks inside that region. The idea is that you can get a sense of scope in different ways.
In this particular case, instead of nesting conditionals, you can use the "elseif" block to test extra conditions. Examine the revised example below:
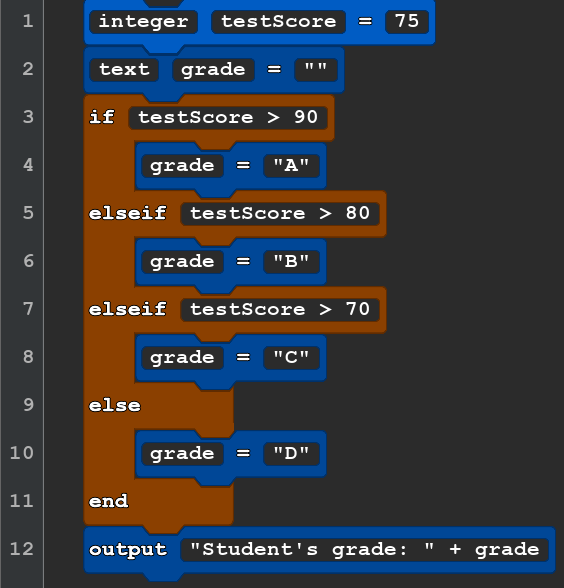
"Elseif" statements are optional extra pieces you can add to an "if" statement. They act as extra conditionals that are only tested if the conditions before them were false. Conditions are always evaluated in order, from top to bottom, and the if statement will never check or run other conditionals or their code if a previous condition was true.
Nothing else worked, so else
As one final block, you can add an else block to an if statement. In text, this might be as follows:
if condition
//do something
elseif condition2
//do a different something
elseif condition3
//do an even more different something
else
//condition, condition2, and condition3 were all false
end
The basic idea is that the computer checks the condition in the "if" first. If it is true, the block executes and then jumps to the final end. If it is false, it then checks condition 2, with the same procedure. The idea is that one, and only one, of the blocks runs.
Engage
Now that you are more familiar with conditionals, it is time to practice using conditionals. Before you make your own program, start by guessing some of the outputs of these conditionals.
Directions
This activity is divided into two parts.
Task 1: Logic it out
When you are creating your conditional statements, you are essentially creating boolean expressions that are either true or false. Practice a few statements for you to determine what the output will be.
1. Does this statement result in true or false?
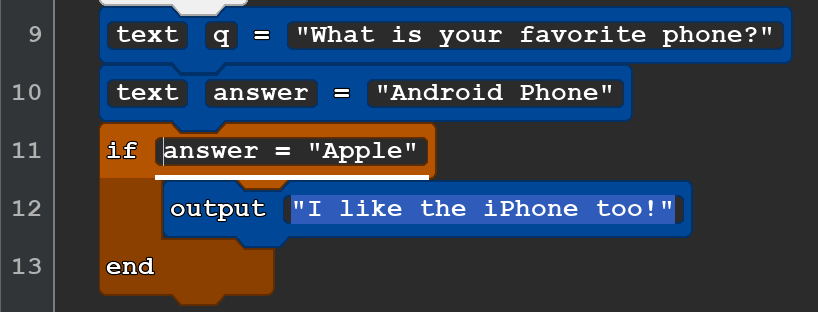
2. What does this code output?
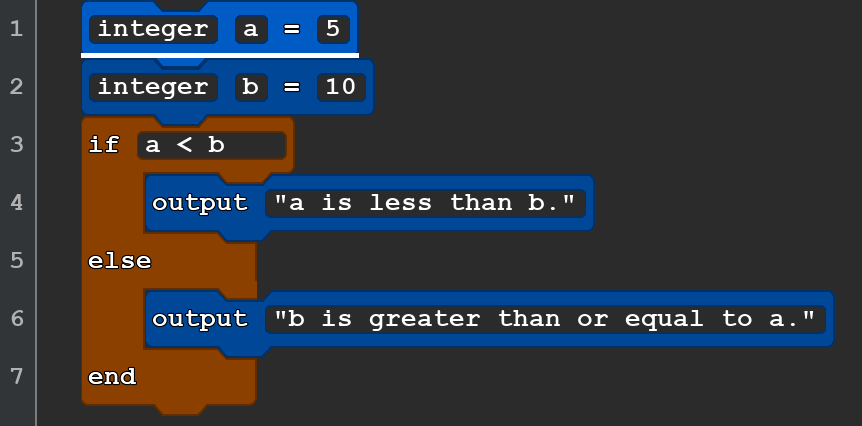
3. What does this code output?
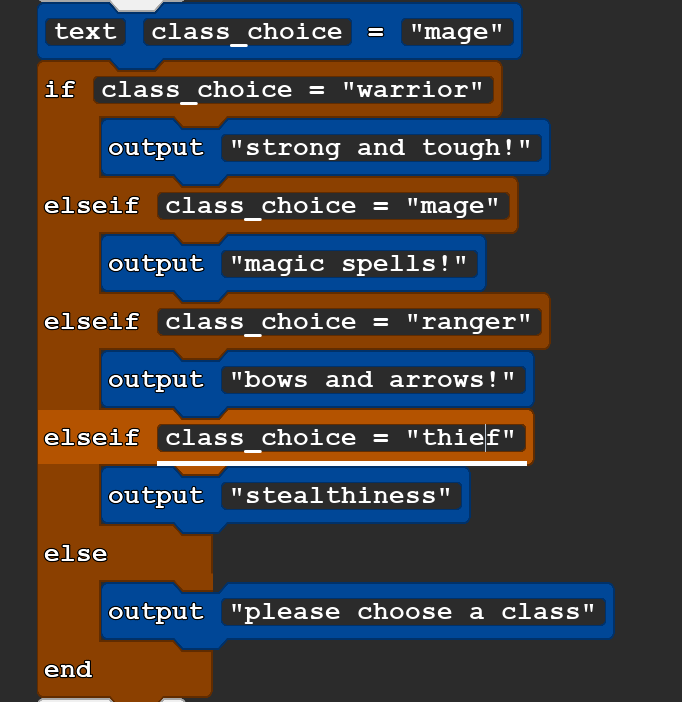
4. How could you change this program in order to make the output statement on line 3 run?
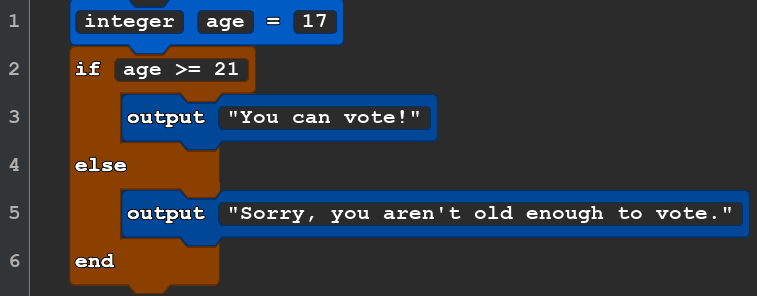
Task 2: Restaurant Menu
In this activity, you will simulate a restaurant, where you will be creating and ordering off of a menu. Your menu should include 4 main courses, 3 drink options, and 2 dessert options.
In your code, you will use a series of "if" statements to evaluate variables containing food orders. To choose your order, assign the food choice in a variable like so:
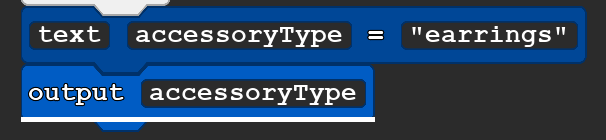
Using your assigned variables and "if" statements, your computer will be your virtual waiter and ask what you would like to eat for dinner tonight. Try changing the values of your main course, drink, and dessert to see how the "if" statements react. Bon appetit!
You are required to include "if" statements, "elseif" statements, and a default "else" statement if a person orders an item not on the menu. At the end, be sure to output all the items that you are ordering.
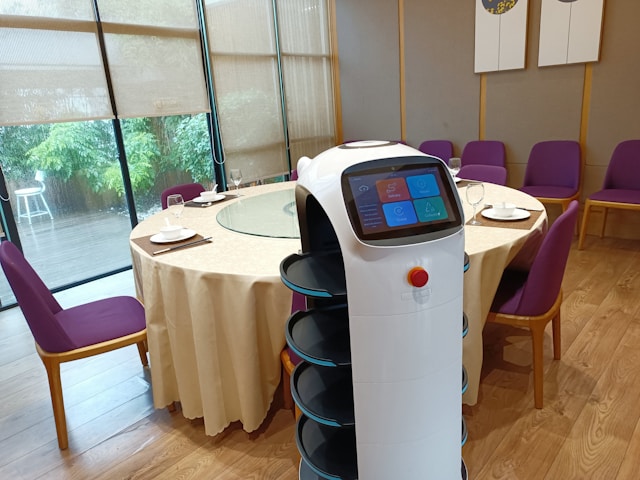
You can use this code sample as a reference:
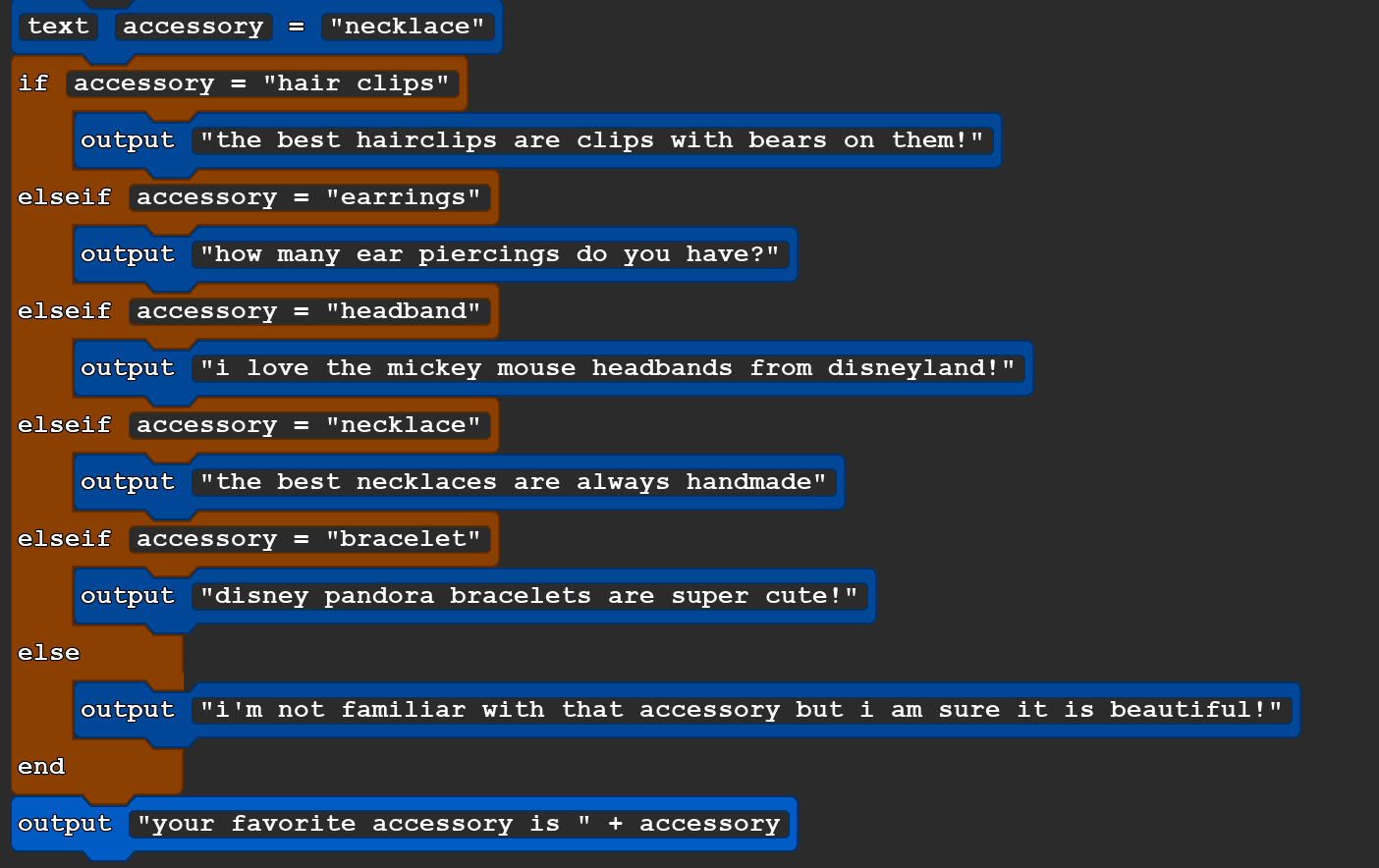
The code example of this below reads:
text accessory = "necklace"
if accessory = "hair clips"
output "the best hairclips are clips with bears on them!"
elseif accessory = "earrings"
output "how many ear piercings do you have?"
elseif accessory = "headband"
output "i love the mickey mouse headbands from disneyland!"
elseif accessory = "necklace"
output "the best necklaces are always handmade"
elseif accessory = "bracelet"
output "disney pandora bracelets are super cute!"
else
output "i'm not familiar with that accessory but i am sure it is beautiful!"
end
output "your favorite accessory is " + accessory
Wrap up
Trade programs with a partner of your choice and have them order off your virtual restaurant. Have a short discussion and answer the following: How do you think an ordering kiosk, such as one in a fast food restaurant, processes orders?
Next Tutorial
In the next tutorial, we will discuss Accessible Form App, which describes how to learn about design and create apps in Quorum.