Hour 8: Loops
This lesson will teach you how to use loops with blocks in Quorum.Overview
Perhaps one of the most common aspects of programming is automation. Computers often excel not just at fast number crunching, but also repetition and decision making. In this lesson, you will explore how to tell computers to repeat themselves. There are many reasons for using repetition. It is a hallmark and fundamental skill in computer science.
Goals
You have the following goals for this lesson:
- Learn how to use "repeat ... times" statements.
- Learn about "repeat while" and "repeat until" statements.
- Discuss how "scope" impacts variables defined inside loops.
Warm up
One of the big benefits of computers is that they can do repetitive tasks that would be tedious or difficult for humans to do. What are some examples of repetitive tasks that technology can handle? What do you think the code for those kinds of tasks might look like?
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Loop | A structure that contains code to be run repeatedly. It runs a certain number of times, or so long as a condition is met. |
Multiple Line Block | A block that takes up more than one line in the editor, and has multiple parts that can be moved and adjusted. |
Scope | The area where a variable exists and can be used. |
Code
You will be using the following new pieces of code:
Quorum Code | Code Example | Explanation |
---|---|---|
repeat INTEGER times | repeat 10 times output "hello!" end | Runs the code inside the repeat block INTEGER times. |
repeat while BOOLEAN | repeat while i < 10 output "hello!" i = i + 1 end | Repeatedly runs the code inside the repeat block as long as the BOOLEAN value is true. |
repeat until BOOLEAN | repeat until i < 10 output "hello!" i = i + 1 end | Repeatedly runs the code inside the repeat block as long as the BOOLEAN value is true. |
end | repeat 8 times output "hello!" end | Marks the end of a multiple line block, like repeat blocks. Repeat blocks run code between the start of the block and the end. |
CSTA Standards
This lesson covers the following standards:
- 3A-DA-12: Create computational models that represent the relationships among different elements of data collected from a phenomenon or process.
Explore
When making programs, you often want to run code multiple times. Sometimes it is more convenient to run the same code over and over again instead of writing out duplicate code by hand. In other cases, you do not know ahead of time how many times the code will need to run.
In computer science, you can run code multiple times by using "repeat" blocks. While the exact terms vary across the discipline, sometimes these structures are known as loops.
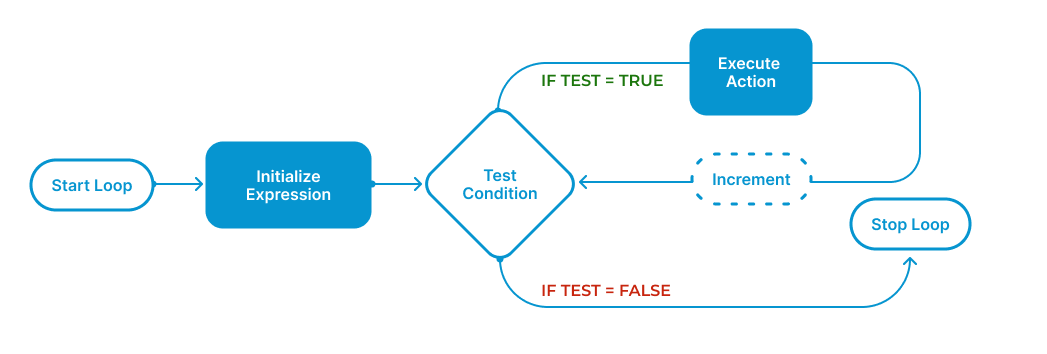
The "Control" Category
In Quorum Studio, the block palette has a category labeled "Control." In this category, there are three different kinds of loops: the "repeat times" block, the "repeat while" block, and the "repeat until" block.
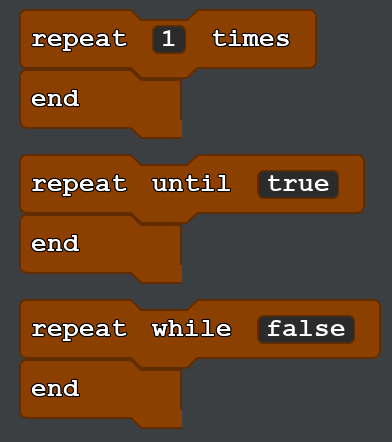
The navigation pane on the left side of Quorum Studio has several tabs, including both the project view and the block palette. To reach the control category using only the keyboard in Quorum Studio, you will need to first to focus the palette, then use the hotkeys to change categories. The table below shows the hotkeys for moving through both the navigation tabs and the block palette with the keyboard on Windows and Mac.
Action | Keys | Description |
---|---|---|
Focus Navigation Pane | CTRL + 1 (Window) or CMD + 1 (Mac) | Focus the navigation pane. This pane has tabs for the project tree as well as the block palette. |
Go to Next Tab/Category | CTRL + Tab (Windows) or CTRL + ] (Mac) | If you are in the block palette, go to the next block category. If not, go to the next tab in the navigation pane. |
Go to Previous Tab/Category | SHIFT + CONTROL + Tab (Windows) or CTRL + [ (Mac) | If you are in the block palette, go to the previous block category. If not, go to the previous tab in the navigation pane. |
(From Block Palette) Focus Navigation Pane Tabs | ESCAPE x2 | While in the block palette, pressing escape once will focus the categories, so they can be tabbed through. Pressing escape twice will focus the navigation tabs so they can be tabbed through instead. |
Repeat blocks are a little different from the other kinds of blocks you have used so far. Previously, each block you have used has been a single line of code. These blocks have two parts on two different lines.
These are multiple line blocks. Besides taking up more than one line, the important part of these blocks is that other blocks can go inside of them, between the first and the last line. The picture below shows an example of a "repeat times" block with something inside of it. In computer science, this is often called a scope.
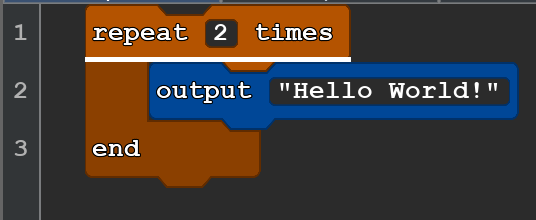
The "repeat times" block is the simplest repeat block. It will run any code inside of it as many times as requested. In the program above, for example, the block will run its code two times. In contrast, the "repeat while" block does not run a fixed number of times. Instead, it checks a boolean condition. If the condition is true, it runs the code. The example below shows some code using a "repeat while" block.
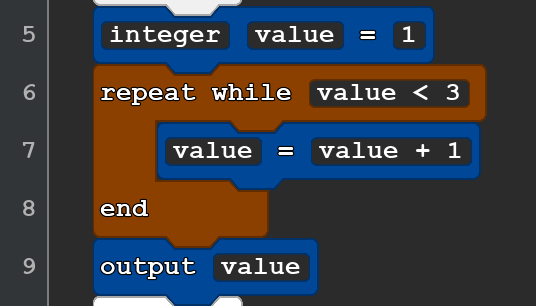
In this example, you start with a variable called "value" that starts at 1. When the code reaches the "repeat while" block, it checks the condition. Because 1 is less than 3, the code executes, and increases the value by 1. Then it checks the condition again.
Since 2 is still less than 3, it runs again, and the value increases by 1 up to 3. When it checks the condition this time, 3 is not less than 3, so it stops. It then outputs the value, which is 3.
The "repeat until" block is the opposite of the "repeat while" block. The "repeat while" block will run if the condition is true, but the "repeat until" block runs if the condition is false. Otherwise, they work the same way. It is always possible to phrase the condition in a way that either block will work. Which one to use is personal preference.
Scope in a Loop
When working with multiple line blocks, you can move blocks in or out of the multiple line block by dragging them with the mouse, or by using cut and paste with the keyboard. Try moving the output block just above the "end" using either the mouse or cut and paste. The picture below shows an example.
When working with multiple line blocks, you can move blocks in or out of the multiple line block by dragging them with the mouse. Alternatively, you can select a block and use cut (CTRL + X on Windows or CMD + X on Mac) and paste (CTRL + V on Windows or CMD + V on Mac) with the keyboard. When pasting a block, the cut block will appear directly after the currently selected block.
Try moving the output block just above the "end" using either the mouse or cut and paste. The picture below shows an example.
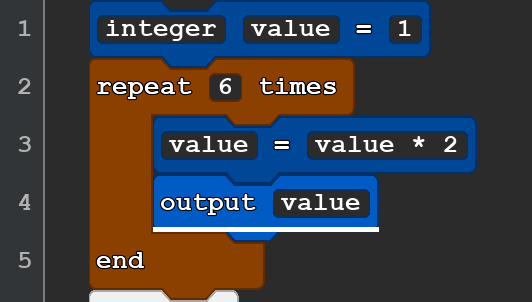
Similarly, you can move blocks into the beginning of the repeat block. In fact, so long as the top part of the repeat block remains above the "end" portion, you can arrange code inside and outside of it however you would like -- though the positioning of code will make a difference. Try moving or cutting and pasting the variable so that it is now created inside the loop, like in the example below.
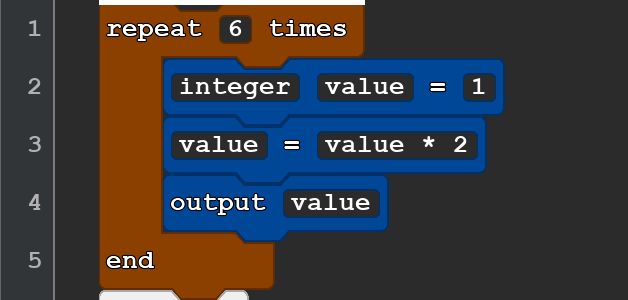
In the example above, the program should now output "2" six times. Now, move the output back down again, out of the repeat block. It now flashes red, and your program has an error.
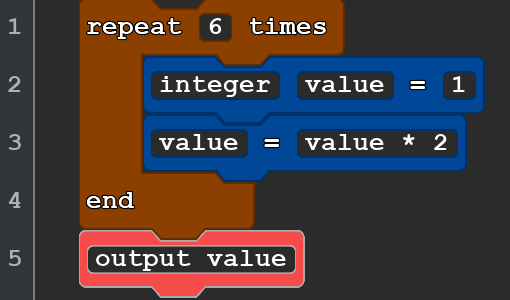
When a variable is created inside a multiple line block like a "repeat" block, it only exists inside that block. The output statement is now an error because, outside of the repeat statement, there is no variable with that name available.
The area that a variable exists in is called its scope. In other words, the scope is the part of the program where a variable is allowed to be used. One of the most common types of scope you may run into is local scope. This occurs when variables are contained within control structures (like "repeat" blocks) and can only be accessed within that code block. If you call that variable anywhere else outside the structure, it will result in an error. This is what you saw earlier with the "output value" statement. Because of this, you must pay careful attention to where your variables are being made and used while working with multiple line blocks.
Engage
Now that you have spent some time learning about loops, it is time to try them yourself. Loops are an important concept in programming. This is also your first time working with multiple line blocks. You will start by interpreting the output of some example loops, and then you will make a program from scratch that uses the different types of loops to solve problems.
Directions
One morning you suddenly wake up and you immediately hear the sound of clanking metal. Weird... you look in your bathroom to discover a small robot. They seem to have gotten stuck reading the "Rinse, Lather, Repeat" instructions on the back of your shampoo bottle over and over.
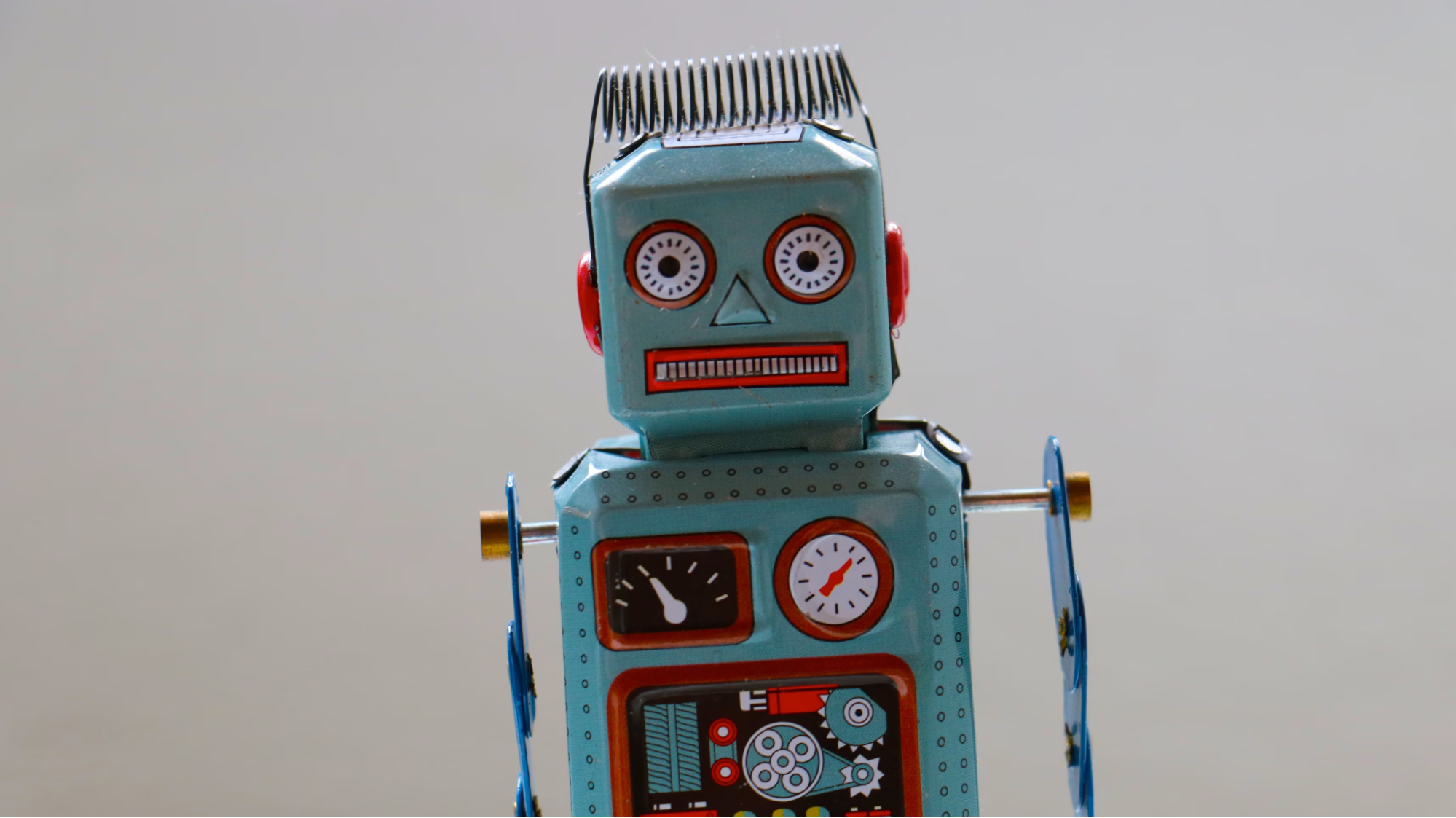
While neither you or the robot know where they came from, they seem harmless, aside from some acute memory issues. You figure that some mental exercises should jog their memory -- or at least keep them from getting stuck reading your shampoo bottle.
Part 1: Identifying Loop Output
In this section, you will step your new robot friend through some examples of loops. Look at each one carefully and determine what the output of the program is. Please write the entire output as if you are running the program. If you need to recreate the loop to see the output, feel free to do so.
1. What is the output of this program using a "repeat times" block?
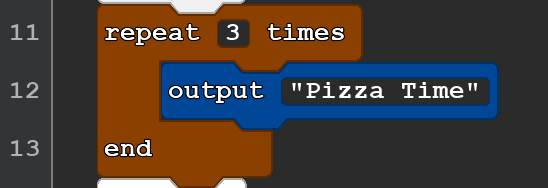
2. What is the output of this program using a "repeat while" block?
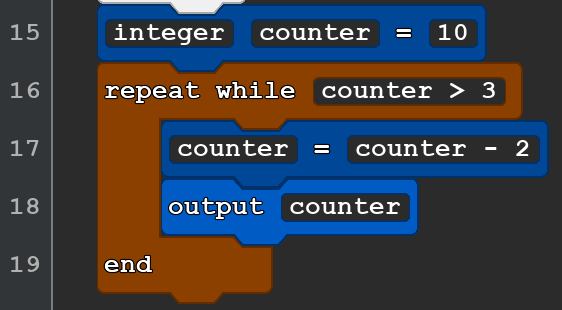
3. What happens in this program where the condition is never met?
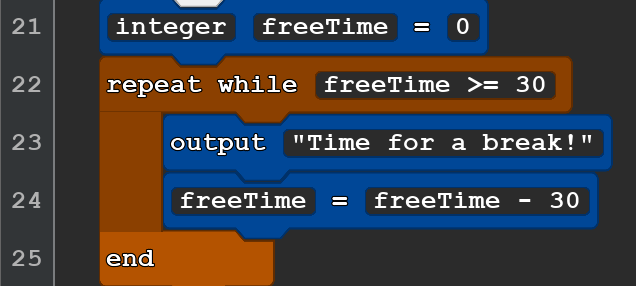
4. What happens in this program where the condition is always true?
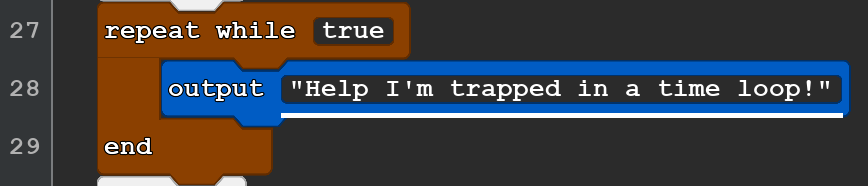
Part 2: Creating Loops
In this next part, you will practice creating loops on your own to solve some repetitive tasks.
Your robot friend has 4 oddly specific requests they would like help solving using loops:
- Output every number between 1 and 8.
- Output every odd number between 1 and 16.
- Calculate 2 to the 11th power.
- Compute 9! (9 * 8 * 7 * ... * 2 * 1)
Use each of the three types of loops at least once while solving the requests.
Wrap up
Take a few minutes to consider the three kinds of loops. While it might seem on the surface important to teach or understand all three, in practice there is significant overlap between them. In fact, you can write any program using only "repeat while" or "repeat until" loops. Given that, what are the pros and cons of teaching students all three right when they are first learning the concept?
Next Tutorial
In the next tutorial, we will discuss Conditionals, which describes how to create conditionals in Quorum.