Hour 2: Scaffolded Blocks Programming
This lesson is to teach you about the templates of different blocks in Quorum.Overview
As you grow, you learn many different kinds of natural languages. Depending on the culture and geographic region you grow up in, this might be English, Spanish, French, or many other languages. Programming languages are in some ways very similar, and in other ways very different, from natural languages. In some ways, they are hard to learn as what they tell computers to do can be esoteric. In others, since the languages are very exact, unlike natural language, they can be predictable.
Today, one innovation common when learning to code is the use of Integrated Development Environments (IDEs). Think about an IDE as your friend when programming because they are built for catering towards specific programming languages and can easily run the language through a concept of compiling. The idea is that humans need help when learning, and even experts need help as well, because programming can be messy and pretty complicated. For this hour, you will tinker with the environments and learn to navigate them.
Goals
You have the following goals for this lesson:
- Learn the basic keyboard and mouse operations for using blocks
- Learn about blank blocks and block templates
- Learn about accessibility devices with block programming
Warm up
Imagine two possible ways of learning English as a second language. In the first, you receive a list of words on a piece of paper, and their definitions in another native language. You then memorize the details and try to freeform link it together. In the second, this list of words is on the computer and can adapt to what is already on the screen as you go. The computer decides what words can be used and when. Traditionally, this is the distinction between text-based and block-based programming. What do you imagine are the pros and cons of both approaches?
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Block programming | Block programming is a style where visual blocks represent pieces of the computer code that can be manipulated through various forms of user input. |
Scaffolded Block Programming | A style of programming where the programming environment adapts as the user controls and manipulates visual elements on the screen to help the programmer know what to do. |
Block Palette | A region in a visual editor that contains information about the code in the editor |
Block Editor | A region in a visual editor that contains all of the lines of code in a users program. The editor can be manipulated with the keyboard, the mouse, or through the block palette. |
Code
You will be using the following new pieces of code:
Quorum Code | Code Example | Explanation |
---|---|---|
say | say "Hello, World" | A command that talks out loud through the computer's speaker |
output | output "Hello, World" | A command that sends text to the computer's console |
if | if true end | A command that allows the computer to make decisions on its own |
CSTA Standards
This lesson covers the following standards:
- 2-AP-13: Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
- 3A-CS-01: Explain how abstractions hide the underlying implementation details of computing systems embedded in everyday objects.
Explore
Visualization and graphics have become a hallmark of how students learn to code. Traditional approaches that make use of graphics vary and include ideas like drag and drop, animated characters, scientific visualization to represent data, 2D or 3D game programming, amongst a sea of other ideas. These ideas can be fun, compelling, and interesting, but have historically had one clear flaw: they were not always accessible to people with disabilities. In this exploration section, you will be exploring and tinkering with an Integrated Development Environment (IDE) designed with a new design philosophy sometimes called Born Accessible. While the term can be interpreted in many ways, in this context there is a simple question "How do you keep the graphics and the fun, but be inclusive of everyone?"
Before thinking about how to use such environments, it helps to consider whether block environments should be used at all. After all, professional programmers, near exclusively, use text and only text based environments to code. Beginners, typically in or before high school, tend to use such "block" environments. Such a common block environment students may have been previously exposed to use would be MIT's Scratch. The bottom line in academic research is that block environments do tend to help people learn, but they also tend to be rather short lived [2]. Students typically use them only for a few weeks. Further, if that learning is rather freeform, meaning students just tinker without direction, this may not lead to positive learning outcomes [1]. Put another way, blocks are helpful to learners, but they have limits and are not magically better for all people under all conditions. There are benefits, but limits.
In this explore section, the next step is to think about how to use blocks. You will explore, optionally, using several possible affordances, including the mouse, the keyboard, and a screen reader. You can either continue to use the project you made last time or make a new one. As a first step, consider what happens if you drag or use the keyboard to put multiple blocks into the environment, including at least one that has a beginning and an end. Here is an example of multiple output statements, say statements, and an if statement (which is for decision making). This might appear as follows:
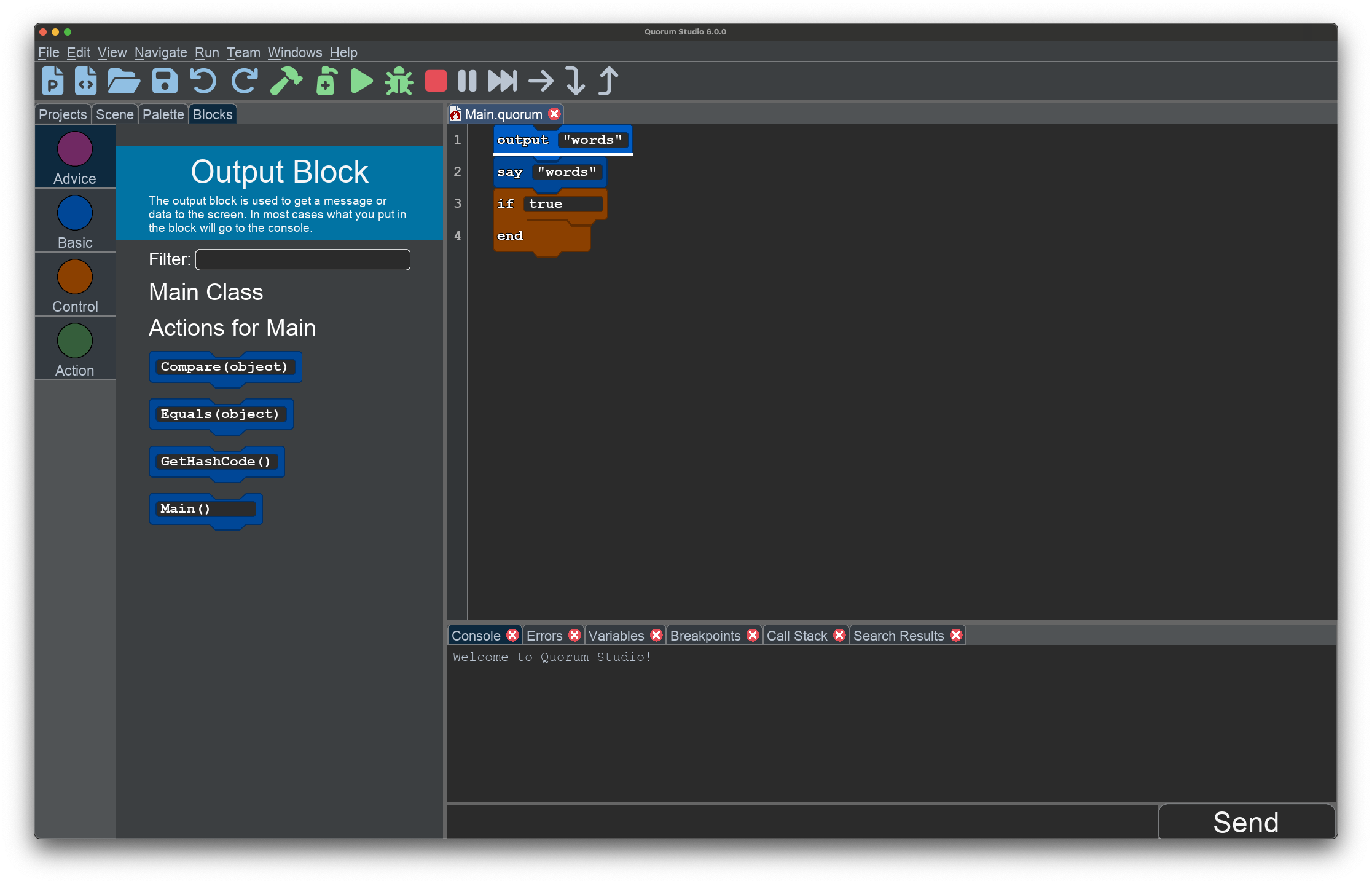
The Double Cursor
When using blocks, there are multiple ways to interact with the environment and the crucial one to understand is called the double cursor. Typical text editors have only one cursor, which is vertical, but block environments sometimes have two, as shown below:
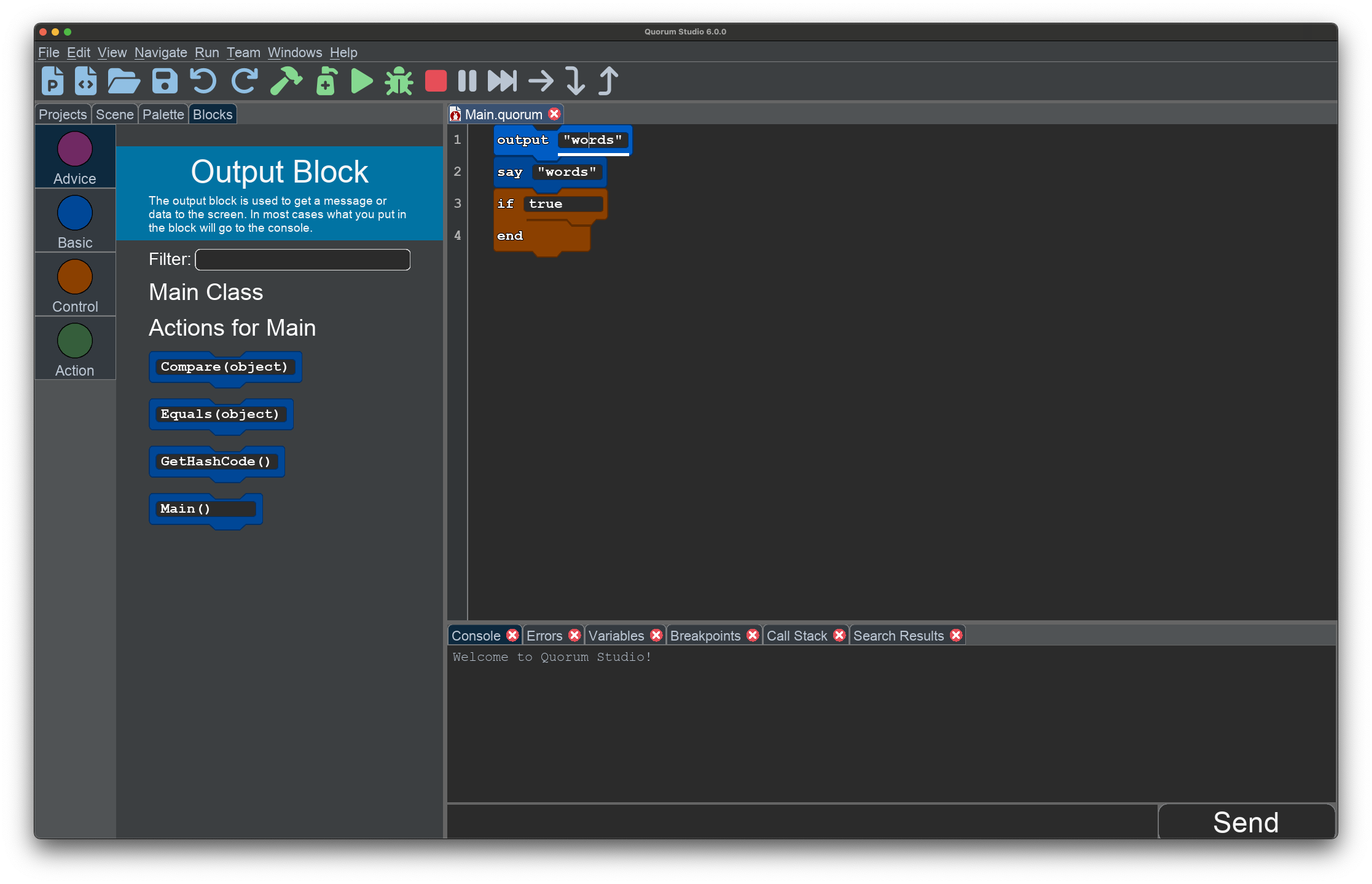
The vertical cursor exists inside of individual text fields or text boxes*. The horizontal cursor indicates the broader location of the cursor and indicates the line, or the location inside a line, of code that the user is currently working with. It provides a visual indicator for users, but also maps to accessibility technologies under the hood so they can obtain the same information. Moving up and down in the editor changes not just the cursor, but also provides information on what kind of code can be written and documentation about the line in focus. For example, moving the horizontal cursor down two lines changes the environment as so:
* The difference between a text field and a textbox is that text fields must be only one line. Textboxes can have multiple lines.
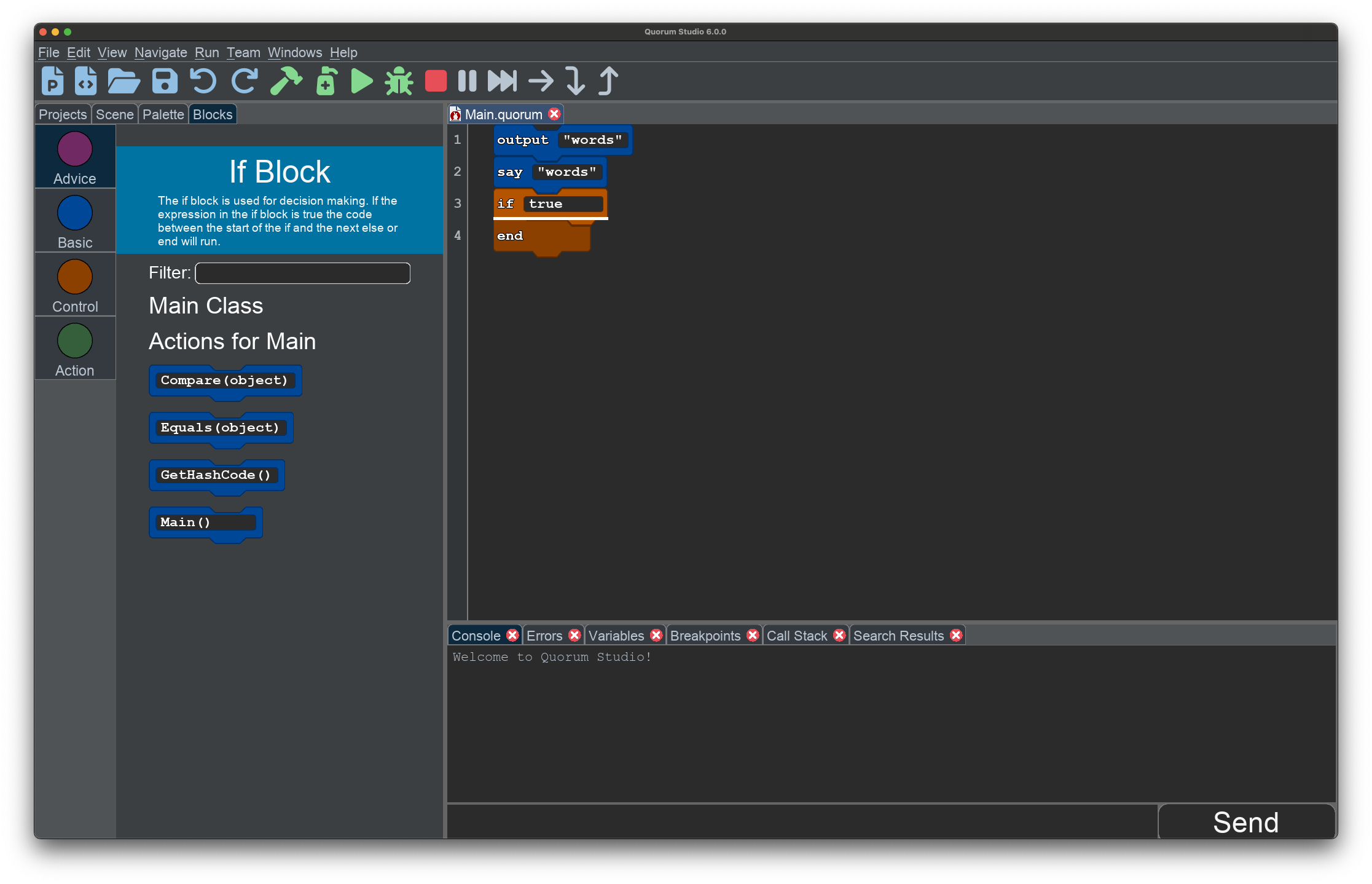
On the left hand side of the screen, a brief description of the block where the horizontal cursor currently resides. In this case, in a blue region are the words "If Block" in the palette with a description of the block. For users that understand code through sound, like a screen reader, or through touch, like Braille, this documentation is available by using the hotkey CTRL + 1 on Windows or COMMAND + 1 on Mac. From there, the tab key allows access through documentation, a filter, and a set of available commands.
A Quick Lesson on "If" Statements
The above block is known as a "if " statement, most commonly known as a conditional. Think about conditionals as a cause and effect situation. Say that you are planning to go outside and you want to dress appropriately:
- If the weather is sunny, then you may want to wear a shirt and some shorts
- If the weather is snowy, then you may want to wear a coat and various warm articles
- If the weather is rainy, then you may want to bring an umbrella and wear a light jacket
Notice how in these statements about the weather, they are structures using the phrase if…then to indicate decision making. You will go deeper learning with these conditionals in a future lesson, but for now, explore what they do and see how they change some of the "logic" in your way of programming.
Blank Blocks
While the palette is a primary way of adding blocks, especially for young users, evidence in the academic literature suggests novices only use blocks for a short time, generally a few weeks [2]. Past this time, learning benefits drop off, compared to text, although the literature is limited in the sense that longitudinal tracking of students has generally not happened. Given block environments commonly allow only the use of the mouse, this leads to transitional friction. The Quorum programming language is somewhere in between. There are blocks, which map 1 to 1 to the text. There is no need to switch or not switch. You can use the blocks if you like them and not if you do not, for learning or inventing anything you wish. You do not have to transition.
For interacting with the environment, while students can use the mouse, the same or nearly the same operations are available through the keyboard. Blocks can be used forever, for any possible program, through the use of the blank block. You can add a blank block by pressing the Enter key and can think of it as a temporary place where you can write in text for a short time.
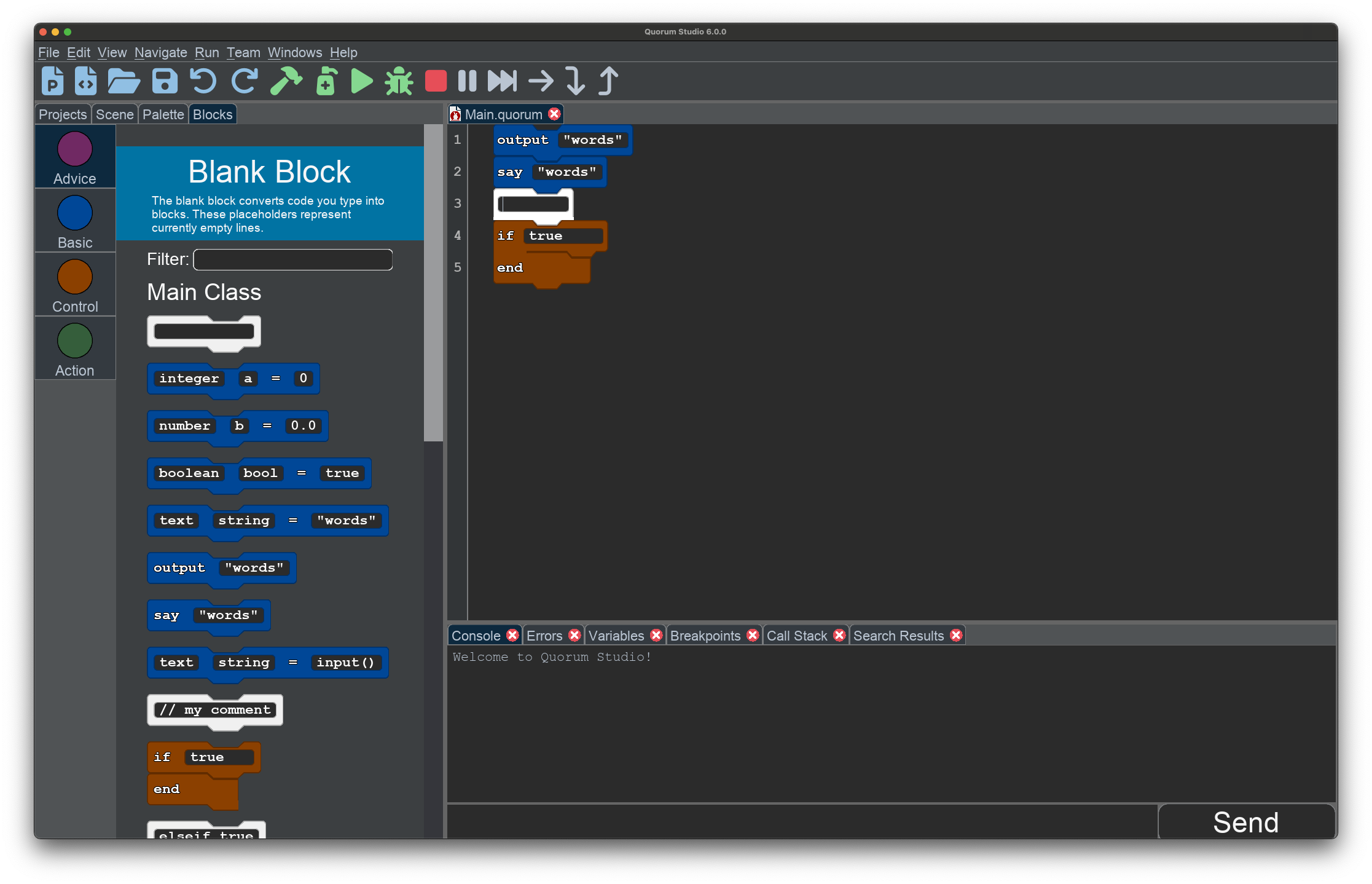
A blank block is white with a double cursor. On it, the horizontal cursor means that this block has the focus and the documentation states that the blank block converts code you type into blocks and are placeholders for empty lines. From this perspective, novices can use the palette as they see fit, as can professionals. The vertical cursor allows the user to write a raw line of code, from memory, which provides a form of a text mode without losing all of the benefits of a full switch between a text or blocks style mode.
Blank Block Templates
Once in a line of code, you can type normal Quorum code. Beginners may not know how to do so, which means they may favor the palette and this is okay. Professionals may already have the language memorized and thus may find it faster to type and this is also okay. You can also make use of templates, which are pre-programmed partial lines of code that can flesh out your code without needing drag and drop.
In addition to typing out full lines of code into a blank block, you can also use templates. These templates provide yet one more optional mechanism by which you can learn to code. The idea is you can type a short mnemonic and the block system will flesh out an entire line. Almost always, because you are typing code that is not a full line, this will temporarily trigger an error, but the system will know what to do. For example, imagine you typed the word "output" into a blank block. The system would show like so:
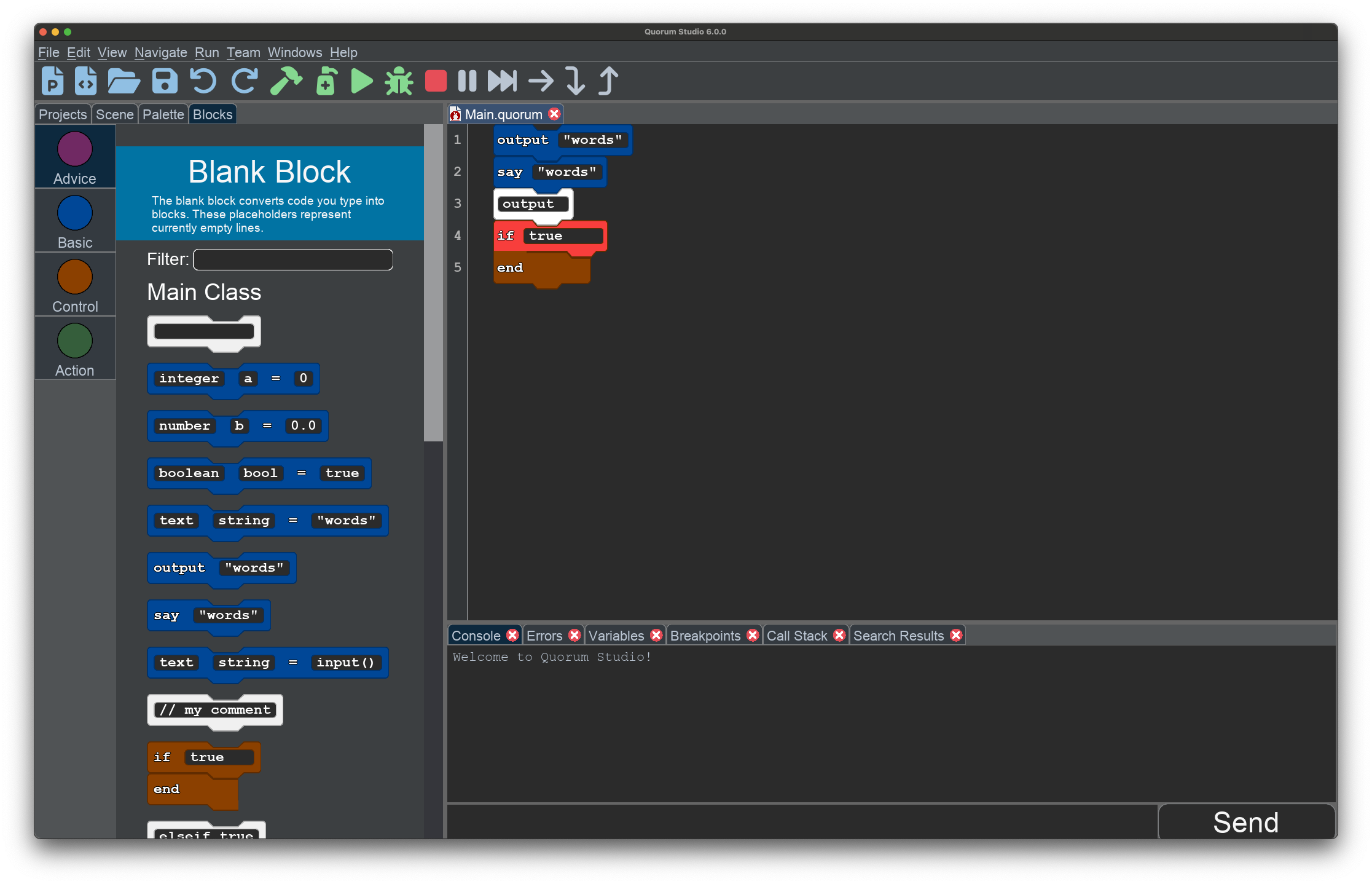
Notice that there is an error, colored in red, at the line of code after where you are typing. However, if you press the down arrow, or enter, the system knows that the word output is a common template and will automatically fix the error on your behalf.
You can see that after pressing enter or however you use it to complete the template, that block changes color to the correct output block shown in the first line.
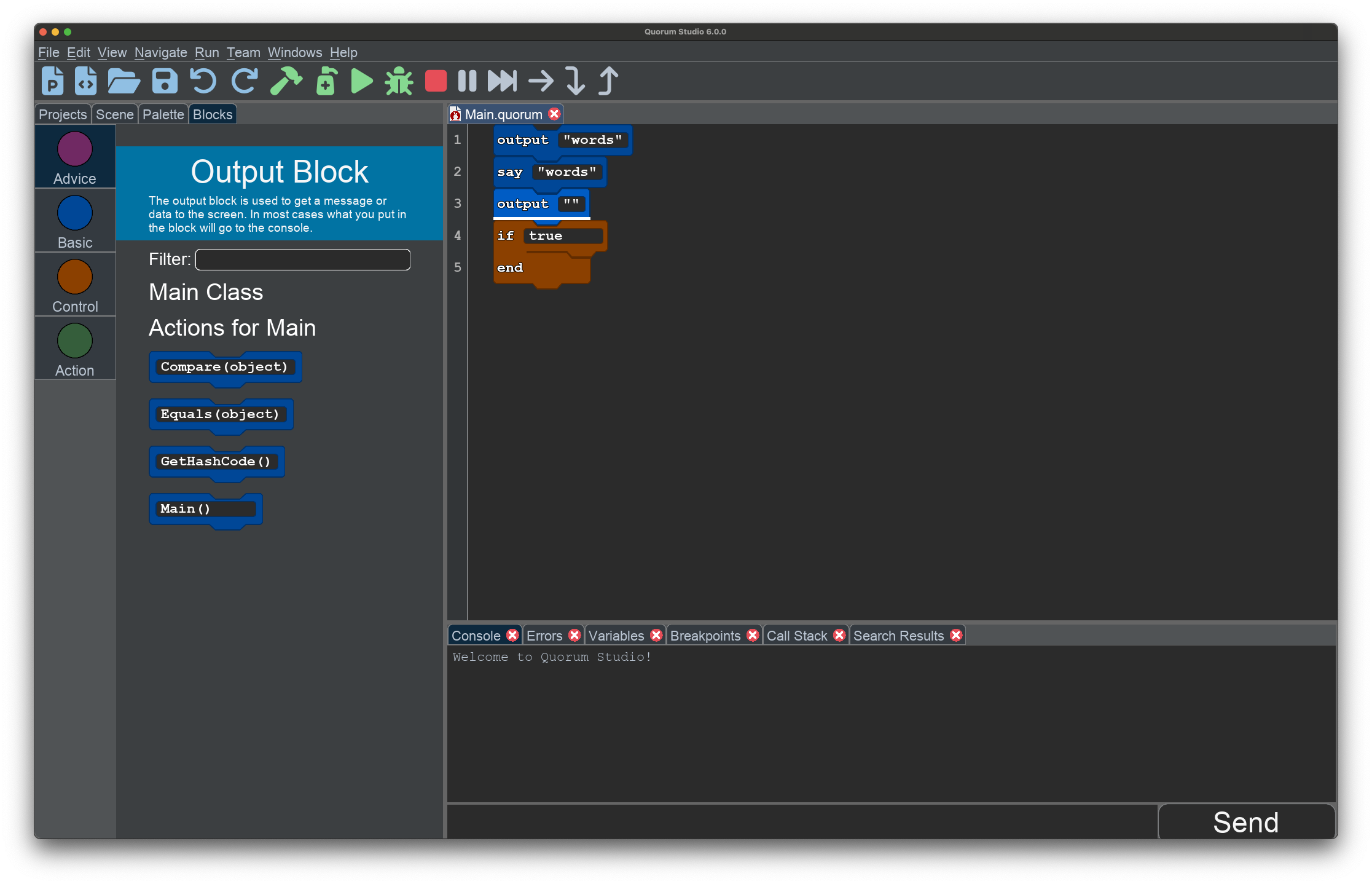
There are many templates that can be used in the system. Feel free to tinker with any of the following templates:
Basic Templates
These are basic templates that can be used to output to the screen, get input from the user, talk, or define variables.
Template | Code Example | Purpose |
---|---|---|
output | output "words" | Output to the console |
input | text string = input() | Get information from the console |
say | say "words" | Talk to the user through the speaker |
integer | integer a = 0 | Define a variable whole number without a decimal point |
number | number b = 0.0 | Define a variable with a decimal point |
boolean | boolean bool = true | Define a variable that can only be true or false |
text | text string = "words" | Define a variable with characters in it |
Control templates
These templates are different in that they may sometimes have a condition attached. For example, the template repeat (condition) times might be typed as repeat 5 times, which would create a loop that does what the name implies.
Template | Purpose |
---|---|
if | Control the flow of a program by making decisions |
if (condition) | Control the flow with an optional condition |
elseif | Control a second part of an if statement |
elseif (condition) | Control the second part with a condition |
else | Control a fallback part of an if statement |
repeat | Cause the computer to do something over and over |
repeat while | Use a particular kind of loop that executes with a condition |
repeat while (condition) | Same as repeat while, but specifying the condition |
repeat until | Similar to repeat while, except the condition is reversed |
repeat until (condition) | The same as repeat until, but with the condition specified |
repeat (integer) | Cause the computer to repeat a fixed integer number of times |
repeat (integer) times | Same command as before except the word times is used |
check | A rarely used command to check for errors |
detect | A rarely used command used to detect certain kinds of errors |
always | An even more rarely used commands that guarantees some code executes even if there are errors |
Structure templates
These final templates are rather advanced, but it is documented here for completeness. They are used for adjusting higher level structures in more complex computer programs.
Template | Purpose |
---|---|
action | This generates a default action with a default name |
action full line | Similar to action, except that you can type a full line of code and the system will respond |
blueprint | Create a placeholder action |
system | Create an action that requires a corresponding plugin. This is an extremely advanced feature and should only be used if careful |
on | Create a constructor |
on create | Create a constructor by typing the full name |
return | Create something to give back from an action |
class | This constructs a class |
shared | An advanced feature that creates a shared class, which the system guarantees there is only one copy of across a program |
Moving, Changing, and Deleting Code
Traditional block programming environments, if the user makes an error, require the user to drag the code to a trash can or otherwise re-enter it through one mechanism or another. Moving, changing, or adjusting code in the Quorum Blocks editor can be done through a variety of methods.
First, code can be dragged and dropped within the editor. Thus, moving a block inside of an if statement can be done through the mouse, like so:
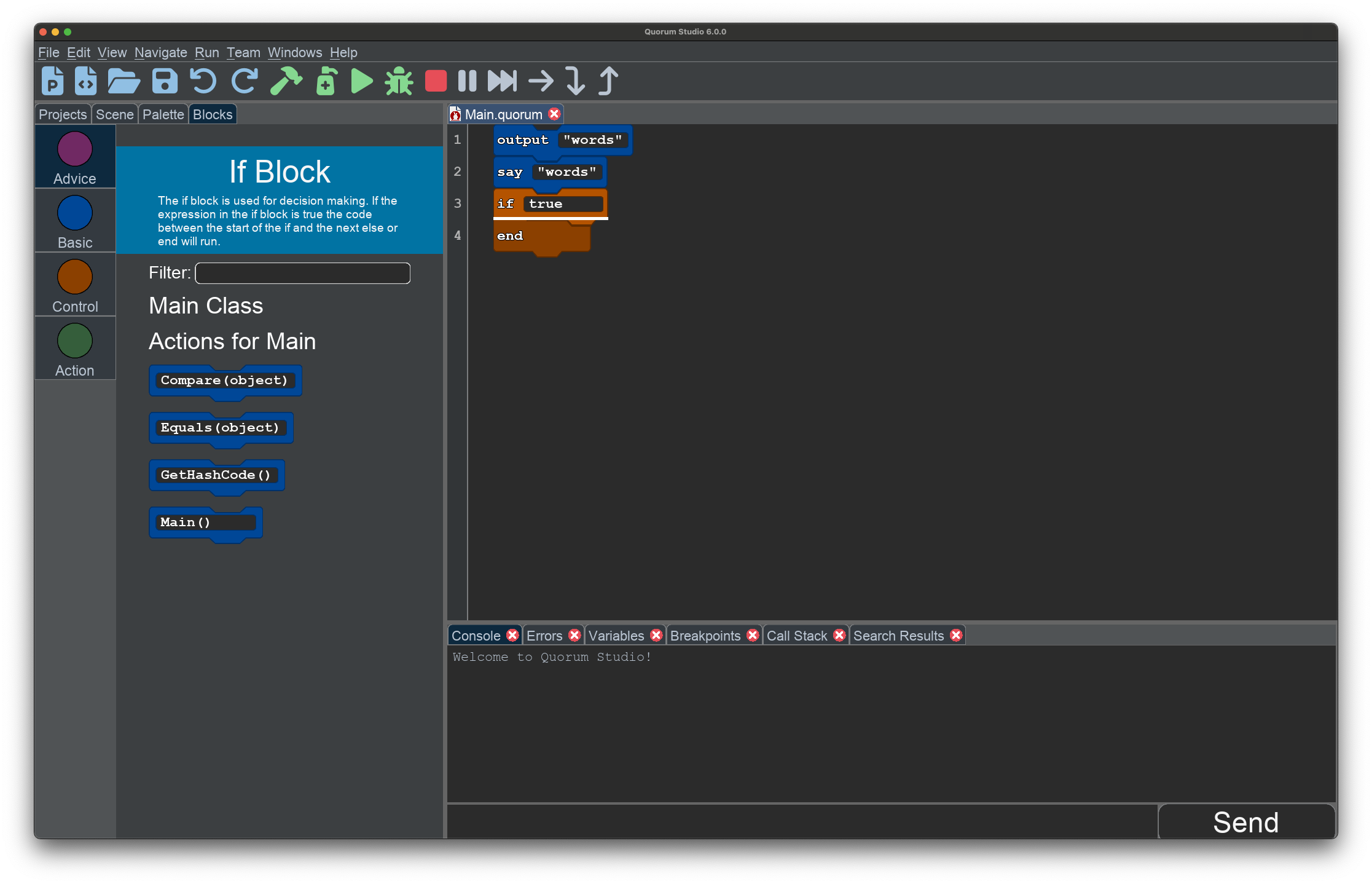
Second, while the mouse can be used, typical controls like cut, copy, or paste, serve the same function. Thus, pressing CTRL + X, or COMMAND + X, on Mac can also move a block. Third, you can delete a block by pressing the delete key.
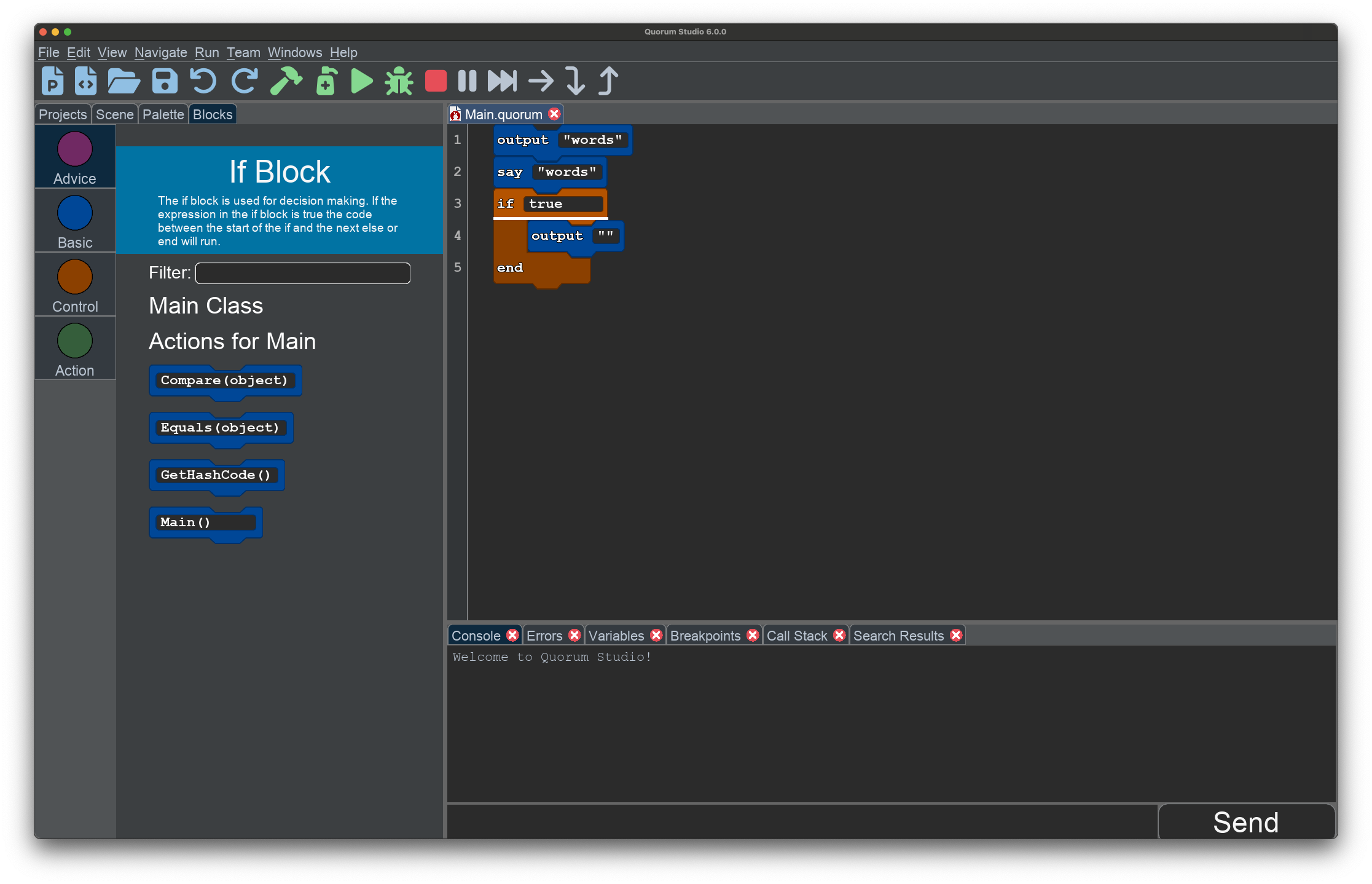
Screen Readers
As one final point, screen reading technologies and Braille devices can be used with the blocks. Such devices read the corresponding line, similar to a text editor, and provide additional context clues that were designed based on academic investigations [3, 4], then modified beyond this [5]. Screen reader users use the same commands as every other user.
To provide some hints on what screen readers say, it is important to know that different readers do provide somewhat different experiences. For example, NVDA, JAWS, and Voice Over do say different things, in part because every screen reader manufacturer gets the ultimate choice in the user experience.
For some hints at the experience, however, consider the if block. In such a block, where other blocks can be embedded, screen reader users are given additional information as they navigate. For example, landing the focus on the block "if true" would state "if true 0 blocks" if there was nothing inside the if statement. Similarly, as the user navigates, there are additional context clues, always at the end of a line of code, that may assist in navigation and discovering where you are in a program.
Past these navigation systems, there are a host of other navigation commands available. The following table provides a selection of the common ones:
Command | Purpose |
---|---|
Up Arrow | Navigation up, moves to the previous block |
Down Arrow | Navigation down, moves to the next block |
Tab | Navigation right, moves to the next part of a block, like the type or variable field, or next block |
Shift + Tab | Navigation left, moves to the previous part of a block, like the type or variable field, or the end of the previous block |
CTRL + SHIFT + H | Smart Navigation up, which jumps to the previous action in a long program |
CTRL + SHIFT + N | Smart Navigation down, which jumps to the next action in a long program |
CTRL + SHIFT + G or CTRL + SHIFT + B (Mac) | Smart Navigation left, which jumps up the current scope |
CTRL + SHIFT + J or CTRL + SHIFT + M (Mac) | Smart Navigation right, which jumps to the next scope |
COMMAND + G or CTRL + G | Jump to a specific line of code |
[1] J. Nathan Matias, Sayamindu Dasgupta, and Benjamin Mako Hill. 2016. Skill Progression in Scratch Revisited. In Proceedings of the 2016 CHI Conference on Human Factors in Computing Systems (CHI ’16). Association for Computing Machinery, New York, NY, USA, 1486–1490. https://doi.org/10.1145/2858036. 2858349
[2] David Weintrop. 2019. Block-Based Programming in Computer Science Education. Commun. ACM 62, 8 (jul 2019), 22–25. https://doi.org/10.1145/3341221
[3] Catherine M. Baker, Lauren R. Milne, and Richard E. Ladner. 2015. StructJumper: A Tool to Help Blind Programmers Navigate and Understand the Structure of Code. In Proceedings of the 33rd Annual ACM Conference on Human Factors in Computing Systems (Seoul, Republic of Korea) (CHI ’15). Association for Computing Machin- ery, New York, NY, USA, 3043–3052. https://doi.org/10.1145/2702123.2702589
[4] Ameer Armaly, Paige Rodeghero, and Collin McMillan. 2018. A Comparison of Program Comprehension Strategies by Blind and Sighted Programmers. In Proceedings of the 40th International Conference on Software Engineering (Gothen- burg, Sweden) (ICSE ’18). Association for Computing Machinery, New York, NY, USA, 788. https://doi.org/10.1145/3180155.3182544
[5] Andreas Stefik, Willliam Allee, Gabriel Contreras, Timothy Kluthe, Alex Hoffman, Brianna Blaser, and Richard Ladner. 2024. Accessible to Whom? Bringing Accessibility to Blocks. In Proceedings of the 55th ACM Technical Symposium on Computer Science Education V. 1 (SIGCSE 2024). Association for Computing Machinery, New York, NY, USA, 1286–1292. https://doi.org/10.1145/3626252.3630770
Engage
For this section, you will try out keyboard navigation in the editor using a screen reader, possibly for the first time. First, a screen reader is an accessibility device to speak out loud, or connect to Braille. There are many of them, but for this exercise NVDA for Windows or Voice Over for Mac are recommended, as both are free and work well. If you are not a screen reader user, this same exercise will highlight keyboard commands, which are used by any user.
Directions
You will now complete a series of three tasks. The overall goal is to document what a screen reader does while practicing using the keyboard or mouse to navigate.
Task 1: Installation
If you are on Windows, you may need to download and install NVDA. Once you have done so, you may need to reboot your computer. Note that you can turn it off with the mouse or with the default hotkey CAPS LOCK + Q. On Windows Laptops, the hotkey is INSERT + Q. On Mac, no installation is required and the command to turn it on or off is COMMAND + F5.
Task 2: Add if statements
While you have not yet discussed what they do, the next thing to do is to add a set of if statements, all inside of each other. You can do this with the block palette, the templates, or through any other mechanism you wish. Here is an example, program:
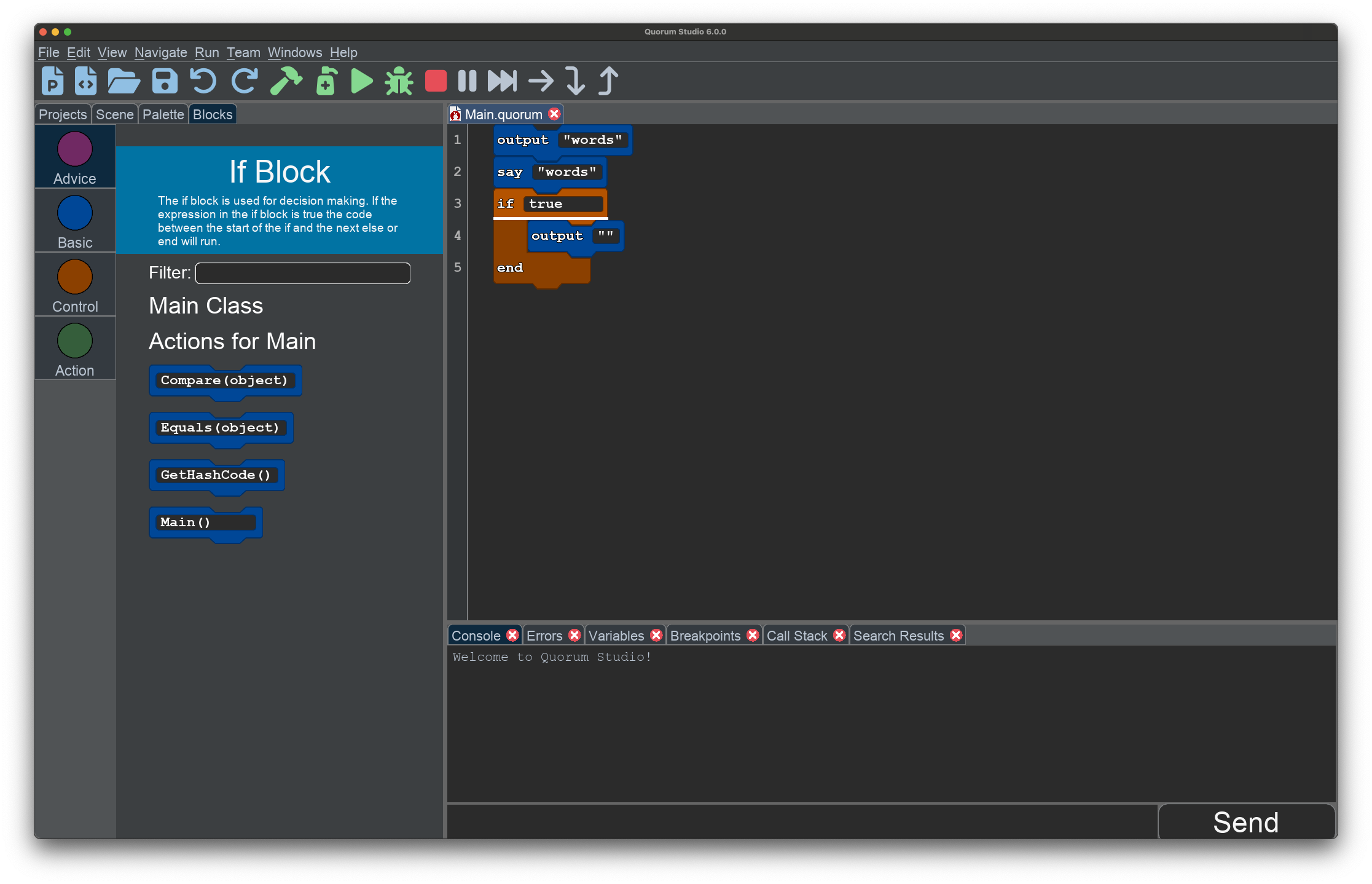
Your if statements will not make for a meaningful computer program, but you are tinkering and that is also an important part of computer science. Any combination of if statements is ok, but be creative and have fun with it.
Task 3: Document what a screen reader says
Once you have created your program, use either the keyboard or the mouse to move the double cursor around in the program you created. Notice that at different levels, and with different things inside of the blocks that the screen reader says different things. Try to find a pattern to what the screen reader says and see if you can derive what the system is doing. You do not need to write code to describe what the computer does, just think through what it might be doing and describe it in your own words.
The important part of this lesson is to recognize how voice over features contribute to adding necessary accessibility to an individual who wants to learn how to program. Think about, discuss, or write the answers to these questions:
- How would a student who requires a screen reader become more successful with a screen reader versus no screen reader when learning how to program?
- Were there any difficulties you faced when trying to understand the screen reader while it was describing these statements?
- Why is it important that technology should be "Born Accessible?"
Wrap up
Once you have completed all three tasks, work with a partner and discuss the pattern you found. Did they match? If not, see if you can improve your description of the pattern.
Next Tutorial
In the next tutorial, we will discuss Variables and Operators, which describes how create variables in Quorum.