Hour 7: Data Science, Part 2 - Accessible Visualizations
This lesson is to teach you about using the DataFrame object to create a chart in Quorum.Overview
One of the most powerful tools in data science is visual representations of information. Historically, however, these visual representations have been limited to the use of "alternative descriptions", or "alt text", for accessibility. In this lesson, you will explore accessible visualization, which is a way to automatically generate visualizations that are, in fact, still visual, but that are sent to the computer in such a way that no alternative description is required. You will use these accessible visualizations to understand a data set.
Goals
You have the following goals for this lesson:
- Learn about the kinds of visualizations that are available
- Construct and explore data using an accessible bar chart, or other chart types
- Discuss keyboard controls in the accessible visualizations you have created
- Learn how to customize the appearance of a chart
Warm up
Data visualization is an important tool for conveying information, and it can appear in your everyday lives. What kind of data visualizations have you seen before and where do you find it useful?
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Alternative Descriptions | Alternative description describes an image or may provide additional context |
Charts | A Chart is a graphical representation of data |
Bar Chart | A Bar Chart is a graphical representation to display categories of data through rectangular bars. Bar Charts can be displayed horizontally and vertically. |
Code
You will be using the following new pieces of code:
Quorum Code | Code Example | Explanation |
---|---|---|
use Libraries.Interface.Controls.Charts.BarChart | use Libraries.Interface.Controls.Charts.BarChart | A use statement lets you use a type of Object in your program. In this case, you are using the BarChart object. |
BarChart NAME | BarChart chart | A variable declaration that makes a new BarChart. You can use the assignment operator on bar chart with a DataFrame |
DataFrame:AddSelectedFactors(column) | frame:AddSelectedFactors("Breed Group") | Selects a column factor from the CSV file into the DataFrame (can be non-numerical values) |
DataFrame:BarChart() | frame:BarChart() | Creates a bar chart with the selected factors and columns |
BarChart:SetTitle(title) | chart:SetTitle("Life Expectancy of Dog Breeds") | Sets the title of the bar chart |
BarChart:SetYAxisTitle(title) | chart:SetYAxisTitle("Maximum Life Span") | Sets the y axis of the bar chart |
BarChart:SetXAxisTitle(title) | chart:SetXAxisTitle("Dog Breed Group") | Sets the x axis of the bar chart |
BarChart:SetColorPaletteToColorgorical() | chart:SetColorPaletteToColorgorical() | Sets the color palette to colorgorical |
BarChart:Display() | chart:Display() | Displays the chart |
CSTA Standards
This lesson covers the following standards:
- 3A-DA-11: Create interactive data visualizations using software tools to help others better understand realworld phenomena.
- 3A-AP-23: Document design decisions using text, graphics, presentations, and/or demonstrations in the development of complex programs.
- 3A-AP-21: Evaluate and refine computational artifacts to make them more usable and accessible.
Explore
There are many different kinds of data visualizations. While the term visualization is used here, all graphics in the Quorum programming language are accessible. Generating a bar chart does present the chart visually, but it also provides screen reader and accessibility information. Just like before, you generate charts with DataFrame objects.
When it comes to describing data, one of the best options to showcase them is visually because people can easily detect patterns and draw conclusions based off of graphs. It provides a visual summary of data rather than having to read and understand blocks of text. From an accessibility standpoint, this still holds. When Quorum generates accessibility information for, say, a chart, it is not described. All interactions and descriptions are generated automatically by the programming language without human intervention or description. There are no, so-called, alternative descriptions, because they are not needed. The following chart types are supported:
Chart | Usage |
---|---|
Bar Chart | To compare categorical data or summary statistics from 1+ groups |
Histogram | To portray sampling distribution with continuous independent variables |
Line Chart | To depict a single variable or multiple variables with respect to change over time |
Box Plot | To show the distribution of data of 1+ groups |
Scatter Plot | To visualize peaks and distributions in numerical data |
Violin Plot | To determine if two variables have a relationship or correlation |
Pie Chart | To show frequencies or percentages |
Bar Charts
Because bar charts visualize quantitative data, it is important that your variables within your x and y axis take discrete values and can be thought of as labels. Typically one axis should be a label or category and the other should represent something numeric. The numeric value is going to determine the length of each bar. Some tips to keep in mind for bar charts include:
- Always start your bars are plotted on zero. This allows for better readability.
- Keep your bars in a rectangular form. Curves or 3D effects make it more difficult to see what the true length is at the bar.
- Consider how you color your bars. Too many colors may distract viewers and might not be able to see any distinctions in categories. The automatically chosen colors are designed to be accessible, but there are limits to how the human eye can process colors.
Chart Accessibility
All charts are accessible by default. All can be navigated with the keyboard and screen reader, in addition to being visual. You may refer to this webpage to find out more general chart hotkeys, but to quickly navigate through the different areas in a bar chart you can use the following keys:
Action | Keys | Description |
---|---|---|
X-Axis Movement | Up Arrow Key | Move to next in sequence |
X-Axis Movement | Down Arrow Key | Move to previous in sequence |
X-Axis Movement | Right Arrow Key | Move to next in sequence |
X-Axis Movement | Left Arrow Key | Move to previous in sequence |
Value Movement | Page Up | Towards smallest valued point movement |
Value Movement | Page Down | Towards smallest valued point movement |
Move into Subgroup | Enter | Move to from a parent to child, for example within a chart to individual bars |
Move out of Subgroup | SHIFT + ENTER | Move from a child to a parent, for example from bars to the parent chart |
You can navigate with this chart accessible controls. Feel free to test out the navigational controls with a screen reader.
Creating a Bar Chart with DataFrames
While you know how to properly load in a CSV file through a DataFrame, the next step is to use that data to create a bar chart. Before you are able to create the chart, there are additional libraries and objects you will need in order to customize the bar chart.
use Libraries.Interface.Controls.Charts.BarChart
BarChart chart
This library adds the BarChart object which allows you to customize and label your chart to your liking. When you are on your BarChart object, take a look at the "Advice" category within the blocks tab. You will see all the available actions that your BarChart option can use. Important notes to consider while creating your chart are to ensure that your data is properly represented and individuals can draw meaningful conclusions.
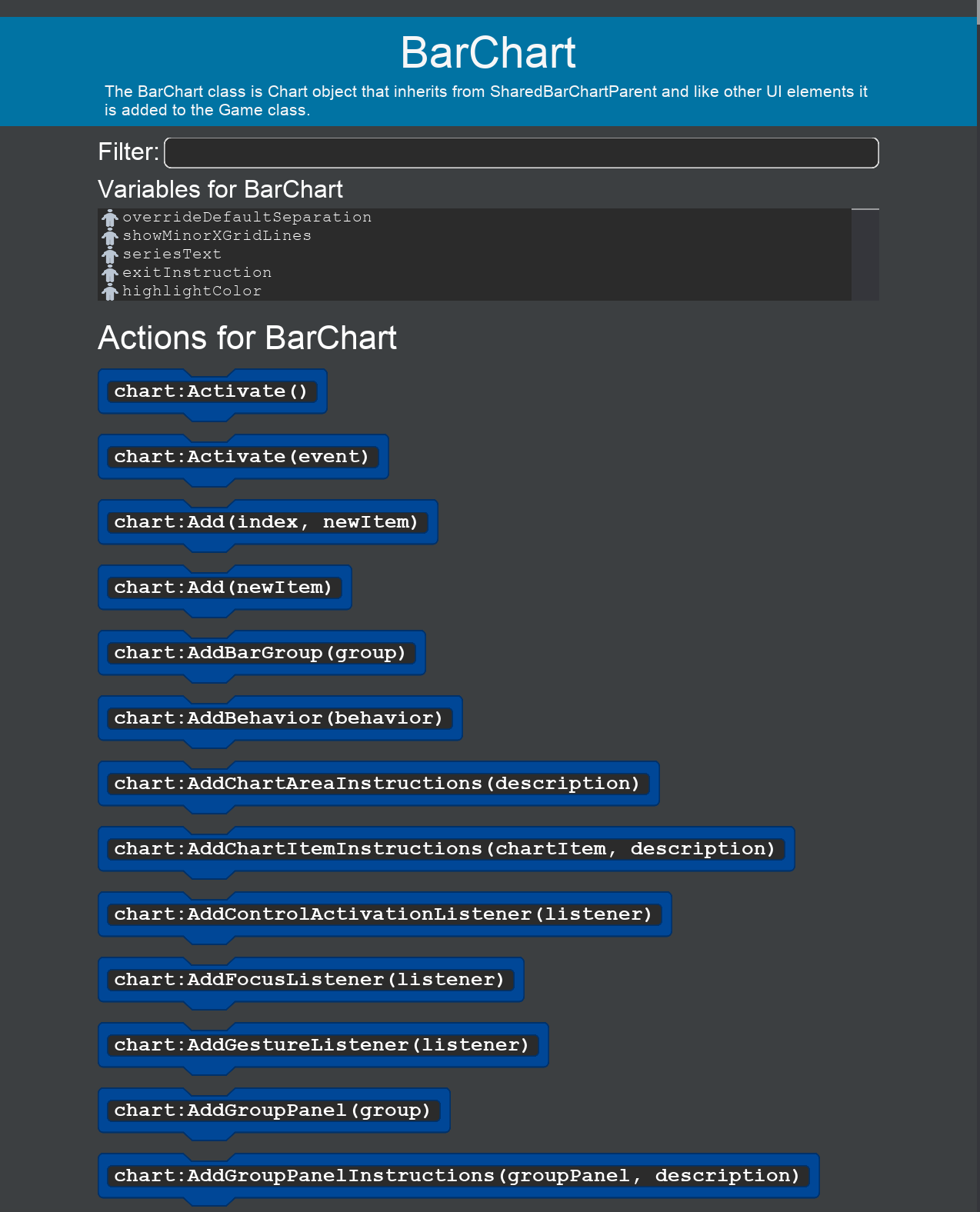
Actions that may be of interest for you while creating your chart include:
SetTitle(name)
: Changes the title of your bar chart.SetYAxisTitle(name)
: Changes the y axis of your bar chart.SetXAxisTitle(name)
: Changes the x axis of your bar chart.SetColorPaletteToColorgorical
: Changes the color palette of your bar chart to a colorgorical color palette. The Cologorical color palette caters towards creating distinctive color combinations for individuals with visual impairments while maintaining aesthetics.
Engage
In this activity, you will display information in a way that makes it easier to understand. You will use accessible barcharts to inspect and compare data about the Palmer Penguins. A common best practice of making any type of good chart is to give clear and concise context to the viewer, but what this means is at least partially subjective. Data visualization lacks in this area where many elements are inconsistent with the entire design. Common green thumbs to note when creating a chart are:
- Creating a descriptive title
- Add a subtitle to your chart for more context (if necessary)
- Label your x and y axis
- Choose a color palette that is distinguishable and clear to your viewers
What you will be working on is going through the entire process of using the DataFrame and building upon your existing knowledge by going through step by step to create a bar chart through the data you have gathered.
Directions
The goal of this activity is to create an accessible bar chart and to customize it to your liking. In the first task, you will create the chart. In the second, you will customize it.
Introducing the Palmer Penguins Dataset
Using real data can be helpful in thinking about data science, and as such consider the Palmer Penguins dataset, a collection of information about three different species of penguins observed on the islands of the Palmer Archipelago in Antarctica. First, download the Palmer Penguins dataset. Examine the CSV file, specifically at the column headers in the first row.
The data contains several categorical variables. The "species" column indicates one of three penguin species, "Chinstrap", "Gentoo", or "Adelie". The "island" column indicates which of the three islands in the Palmer Archipelago the penguin was found on. The "sex" column indicates if a penguin was "male" or "female". Finally, the "year" column indicates what year the measurements were collected for that row of data.
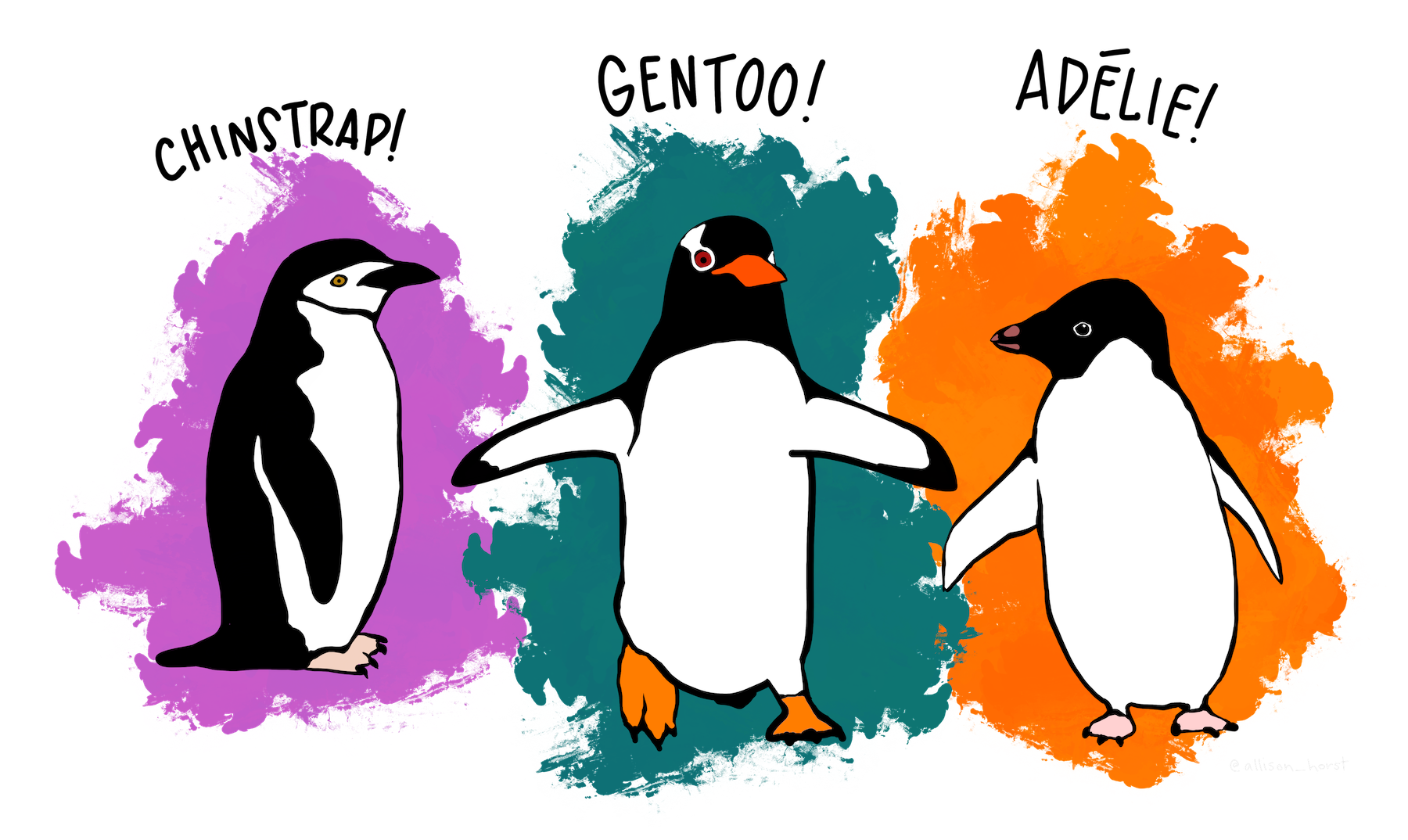
There are also several quantitative measures, or in other words, several values where numbers were measured for each penguin. These include the length and depth of the penguin’s bills in millimeters, the length of their flippers in millimeters, and their body mass weighed in grams.
It is worth noting that a few entries in the data read "NA", especially in the "sex" column. These are cases where that particular information could not be measured for a particular penguin. There are lots of potential questions you might ask about these penguins, and lots of potential answers you could get from the data.
Task 1: Create the Chart
First, create a new project for your code. Next, download the Palmer Penguins dataset and place it in your project if you have not done so already. You can do this by moving the file into the root directory of your project. The root directory is the folder that has the project’s name, and contains a "Project" and "SourceCode" folder.
On Windows, by default your project will be located in Documents/Quorum Studio. On Mac, by default, your project will be located in Users/Quorum Studio.
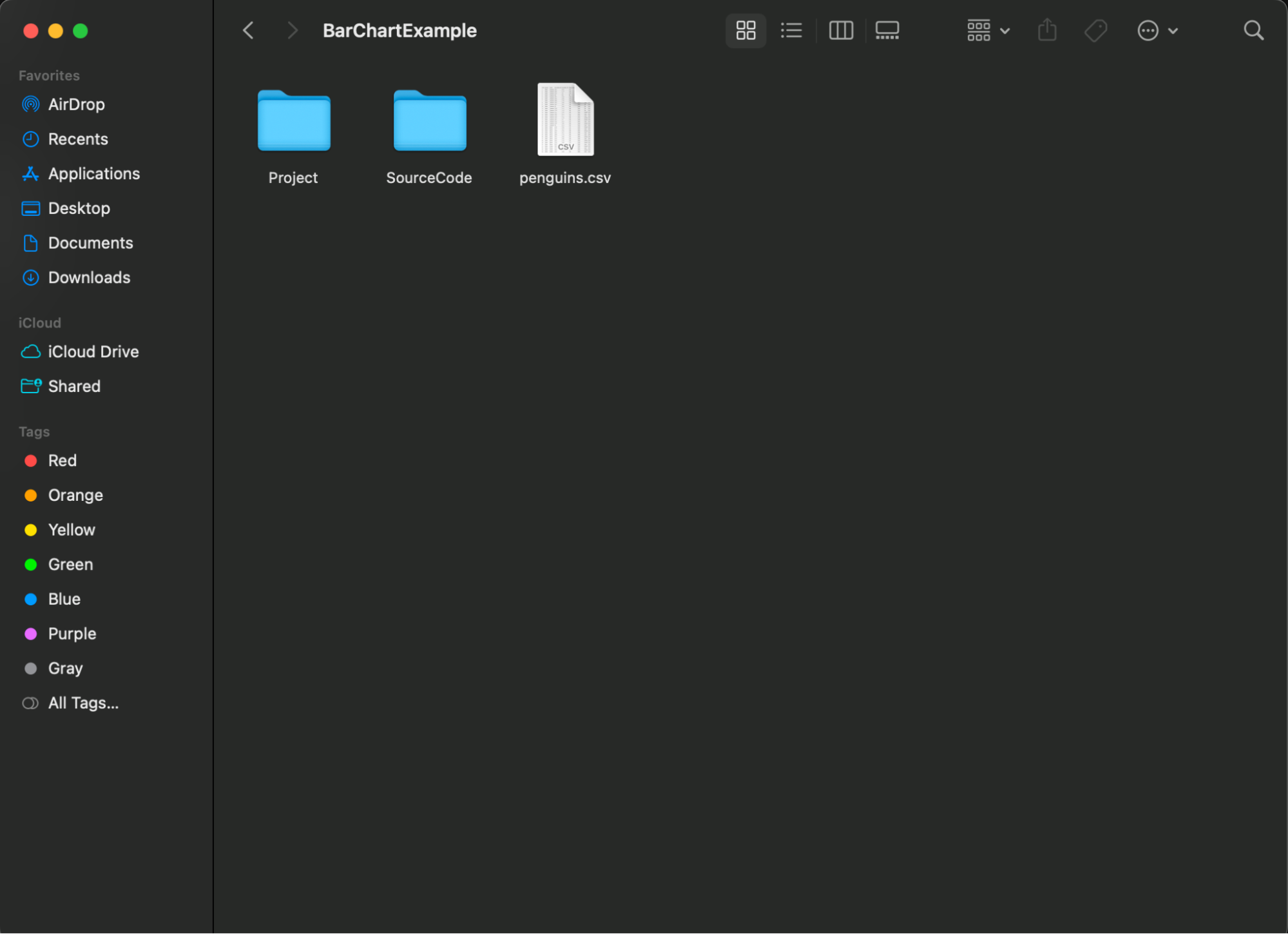
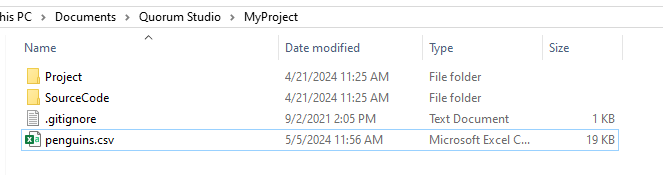
Next, you need to program your barchart. You need the following two libraries:
- Libraries.Compute.Statistics.DataFrame
- Libraries.Interface.Controls.Charts.BarChart
Including these libraries allows you to create the DataFrame object and now the BarChart object, which you can name as you see fit. For demonstration, the DataFrame will be named "frame" and the BarChart will be "chart."
To load in a file, you will want to use your frame object and use the keyboard or the mouse to put in the Load(location) block into the code editor. Inside where the location is, you will need to replace it with the location of the penguin CSV file. Typically, you can decide if you want to have a separate data folder for all your CSVs therefore, the path you would put inside the parameter would be "data/penguins.csv" however since you are working with only one dataset, it is not entirely necessary.
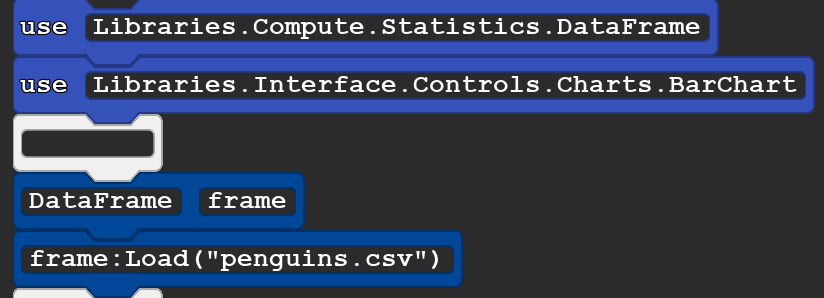
You will now want to select specific columns of the dataset and play around with it. Make sure you can see the actual dataset so you are able to correctly pull out the correct columns for your charts. This is where you will need the actions AddSelectedColumns() and AddSelectedFactors().
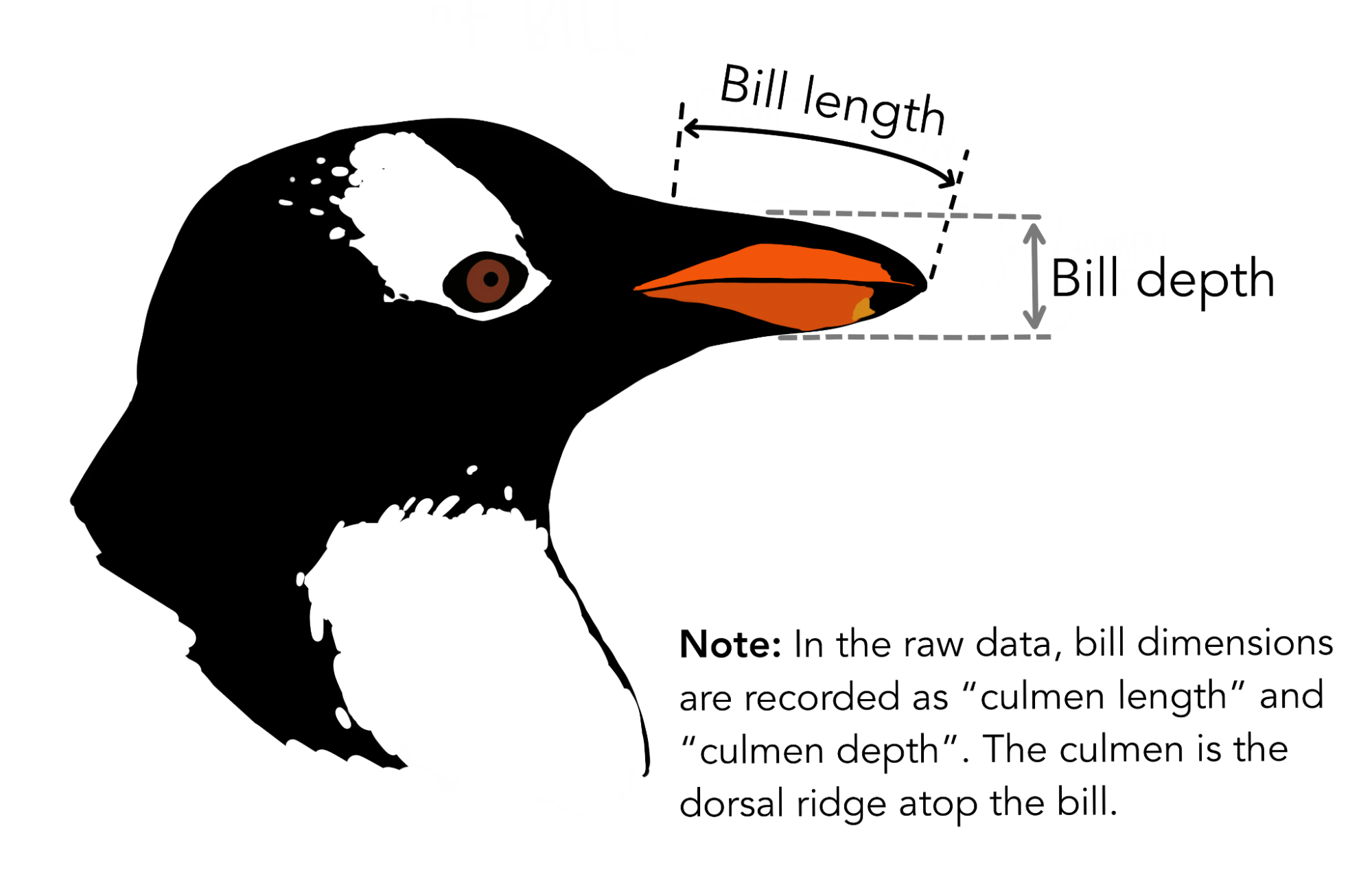
The difference between the two actions is that columns will typically take all the numerical data you have whereas the factors will separate these numerical data points into categories. Remember that you call the actions such as:
frame:AddSelectedFactors("species")
frame:AddSelectedColumns("bill_length_mm").

Next, the following line of code creates the bar chart and places is in a variable:
BarChart chart = frame:BarChart()
Finally, you call the action chart:Display()
.
A game window pops up when you run the program that displays your chart. It does not look like much, but this is where you can learn how to customize it to your liking such as fixing labels and adding different color palettes to your chart.
See if you can add more columns to create more bars inside your bar chart. You may run into errors in this part, but that is okay. An easy way to look at adding more to your chart is to take a look at the numerical values left in the dataset. Possibly there are more ways to categorize these three penguin species.
Task 2: Customize Your Chart
Use the palette and examine the various actions that can be called. These may include the title, color options, and others. Change your chart to have custom details and then examine visual changes, or those with accessibility devices, and examine how they vary. The following is one example of how you could customize your chart.
Wrap up
Visualization, as it is sometimes called, has historically been a visual only area, with alternative descriptions created by a human to replace the visual. In this system, however, a visualization automatically generates many alternative descriptions automatically and lets you explore. Unlike some Artificial Intelligence (AI) systems, this does not hallucinate and is guaranteed to be a reflection of the data. Compare and contrast with each other the pros and cons of these approaches.
Next Tutorial
In the next tutorial, we will discuss Loops, which describes how to use loop blocks in Quorum.