Hour 4: Libraries and Objects
This lesson is to teach you about libraries and objects in Quorum.Overview
Imagine you are a musician and play the violin. While you can play the main melody of a song, imagine if you were part of an orchestra and you have additional accompaniment such as cellos, basses, violas, or more violins. The music you play altogether sounds stronger and there is the added harmony. As a whole, these other instruments work together to make music sound better. Computers have their own accompaniment: libraries. Basically, libraries are code that usually someone else wrote and in this lesson you are going to learn about them. They are useful because they let you write more advanced code much more quickly than inventing it all yourself.
Goals
You have the following goals for this lesson:
- Learn how to use libraries
- Create object variables
- Learn how to tell objects to take actions
Warm up
Some types of applications are harder to invent than others. For example, a variable is a relatively simple thing, whereas a self-driving car might take a generation or two to invent with many engineers working on it. For something you are interested in inventing, what kind of libraries do you think you might need that you do not immediately know how to invent yourself?
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Class | A custom data type. Classes are a plan for constructing these custom data types in memory |
Object | A class is a plan, whereas an object is one copy of that plan. |
Library | A library is a collection of code that defines a type of Object. Using a library lets you use the type of Object it describes. |
Package | A package is a categorized group of libraries. |
Action | Actions are tasks that can be performed by variables. Different Object types have different Actions available. |
Parameter | A parameter is a single piece of information that is input into an action when it executes. |
Standard Library | A set of libraries that are standard in a particular programming language. Most languages have invented their own standard library. |
Code
You will be using the following new pieces of code:
Quorum Code | Code Example | Explanation |
---|---|---|
use LIBRARY | use Libraries.Compute.Math | A use statement lets us use a type of Object in your program. |
TYPE NAME | Math math | A variable declaration that makes a new Object. Unlike primitive variables, Object variables do not need the "=" sign. |
NAME:ACTION() | math:Action() [ex. math:AbsoluteValue(-5)] | Instructs the variable to perform a specific, pre-programmed task |
CSTA Standards
This lesson covers the following standards:
- 1B-AP-14: Observe intellectual property rights and give appropriate attribution when creating or remixing programs.
- 3A-AP-15: Justify the selection of specific control structures when tradeoffs involve implementation, readability, and program performance, and explain the benefits and drawbacks of choices made.
- 3A-AP-18: Create artifacts by using procedures within a program, combinations of data and procedures, or independent but interrelated programs.
- 3A-AP-20: Evaluate licenses that limit or restrict use of computational artifacts when using resources such as libraries.
Explore
Sharing code can save time and effort. Many computer scientists have worked hard to create functionality and, if that code is under a license where others can use it, may be useful to others. In order to share code, programmers organize their work into libraries.
A library is a collection of code that is organized to handle a specific kind of task. You can bring libraries into any program to help solve problems using pre-written code. To give an example from math, while addition and subtraction are easy, many more complex mathematical operations exist (e.g., logarithms, trig functions). Hypothetically, you could reinvent all of these systems, but they are difficult to write and understand.
Quorum has many different libraries available, all of which under an open software license called BSD-3, which really just means they can be used for commercial or non-commercial purposes. Many other programming languages and environments exist with many being open source under a relatively open license.
While it would not make sense to use for light reading, the Quorum Standard Library index page has a list of all libraries. The Quorum Standard Library contains all pre-written code functions that can be used to make your programs easier to write. Here you will see a list of libraries on the left side of the page. The libraries are organized into groups called packages. Packages are named in a way that sort of resembles a web address, using words separated by periods.
One example of a library no rational person would want to write on their own is the Math class in the package named Libraries.Compute. Mathematical equations are important, no doubt, but dry and can be complex to figure out how to do them correctly and accurately. The center of the page shows documentation for the Math library, including a brief summary, an example, and a list of variables and actions.
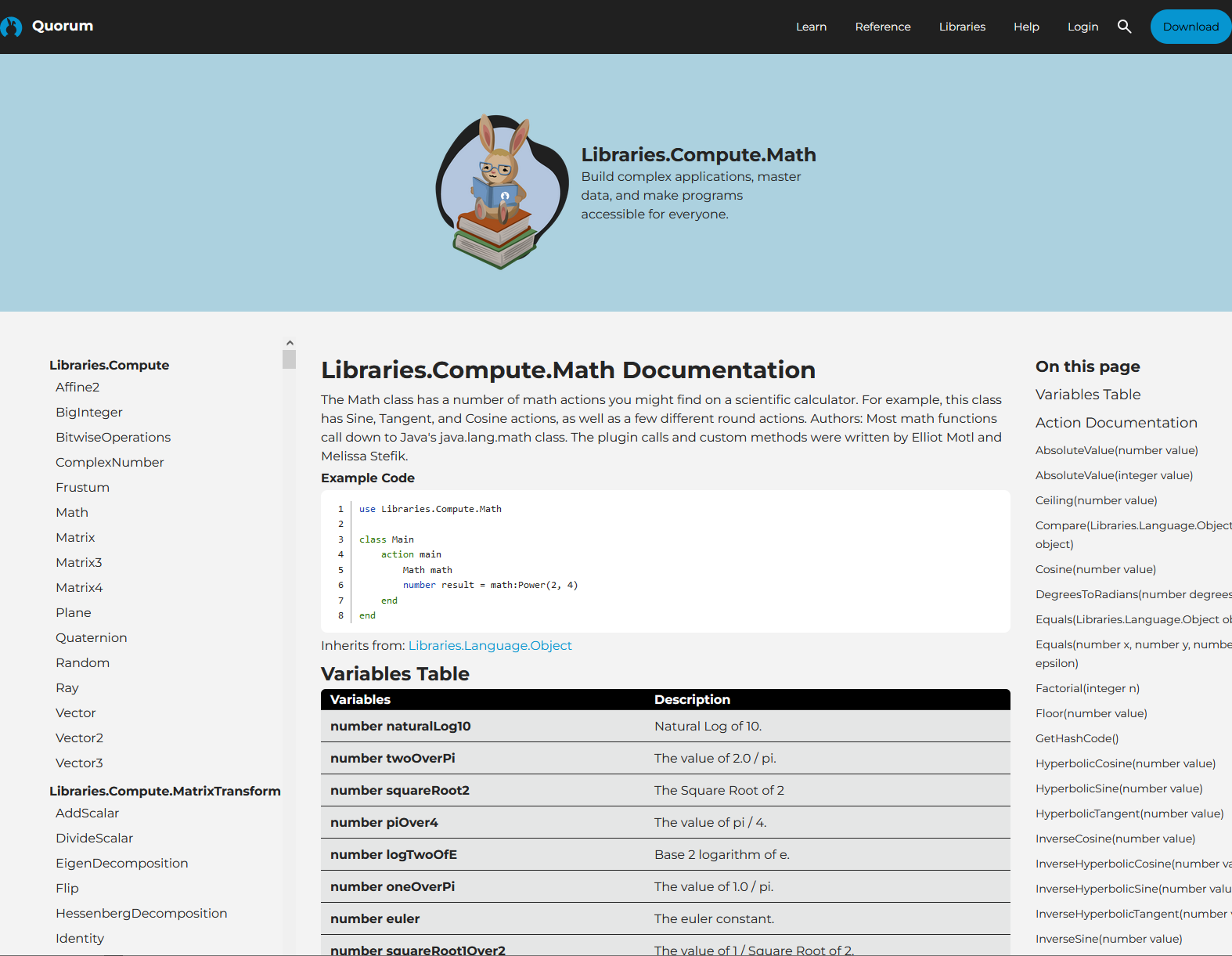
Programming languages often have quite a few libraries to choose from. Specifically in Quorum, for example, there are libraries for graphics, accessibility, many kinds of math, data science, gaming, robots, and much more. Normally, in a course on computer science, it would be unrealistic to learn it all and this is okay. Focusing on bigger picture concepts, while having fun in a domain of interest to you, is typical.
Math Library
Now imagine using the Math library. In Quorum Studio, you can again create a new Console project from the File menu, using the toolbar, or using the hotkey (Ctrl + P or COMMAND + P for Windows or Mac, respectively). Open the Main.quorum file in your new project.
In order to add a library to your program, you need a new block, the "use" block, which is located at the bottom of the "Action" category. Add the "use" block to your program. Your program should look like a red block that contains an error.
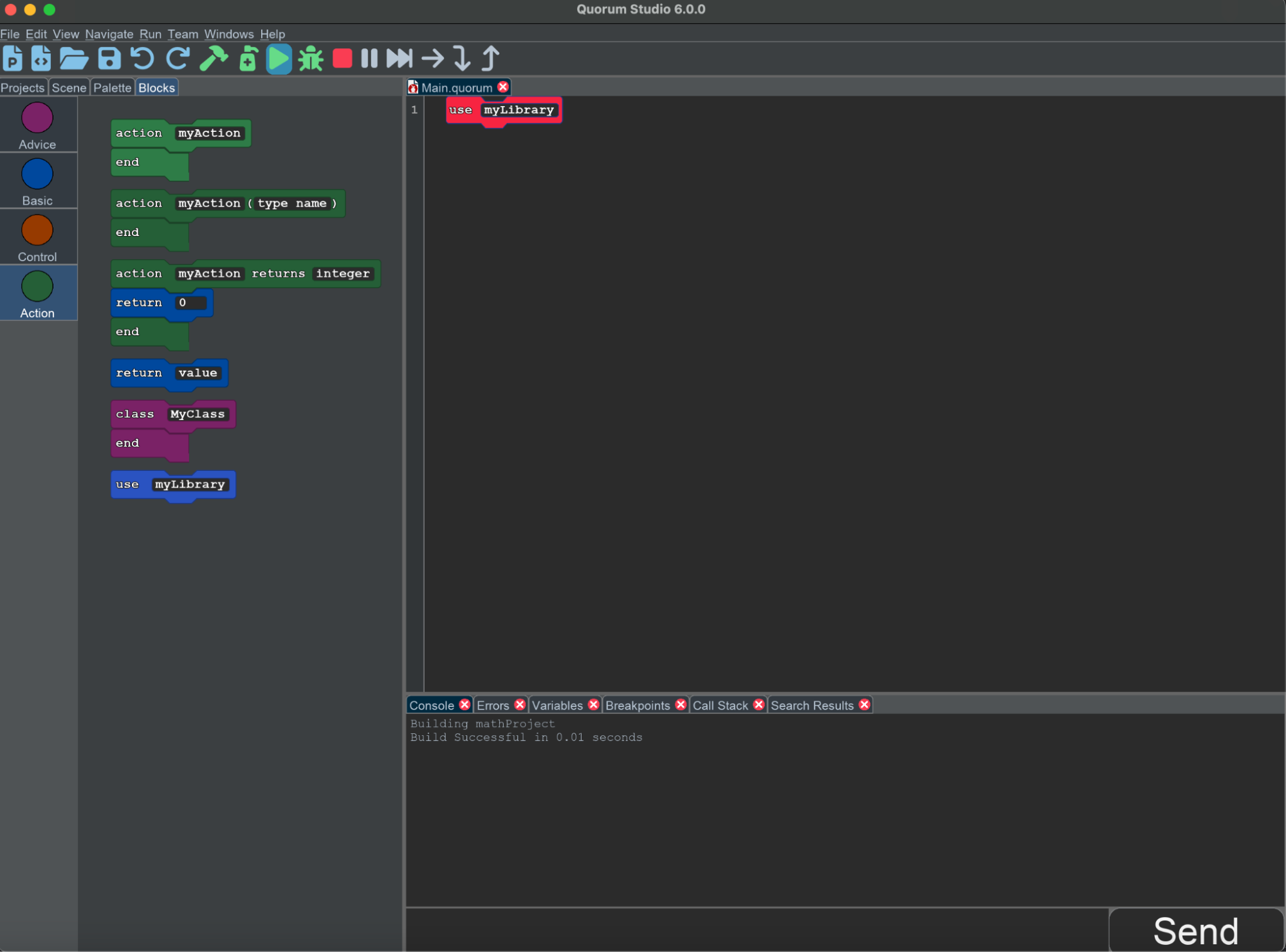
Inside the editable area of the block, it reads "myLibrary." This is a placeholder value that must be replaced with the name of the library you want. At this point, the block should visually flash red, indicating that it is an error. In a screen reading device, if you land on a block, it will say something similar to:
use myLibrary, ERROR + HINT. I could not locate the package named myLibrary. Are you sure you spelled it correctly?
For Math, the full name of the library is Libraries.Compute.Math.

With only this single line in the program, the block will still flash red, which seems wrong but is not. A single use block is telling the programming language you want access to a library, but you do not want to do anything with it. If you add more to the program, the block should stop flashing red.
If you then add a blank block. You can add it from the palette from the "Basic" category, or you can just select the "use" block in code and press Enter to add a new blank block beneath it. A blank block is exactly, and literally, equivalent to writing a line of code, blurring the line between blocks and text.
In this blank block, to use math, you need to write a line of code to make an object. This is similar to the variables in the last lesson, but this time, you are going to be using a special type. The variable type is "Math." The name can be anything that follows the variable naming rules, but in the example below, the name is "calculator."
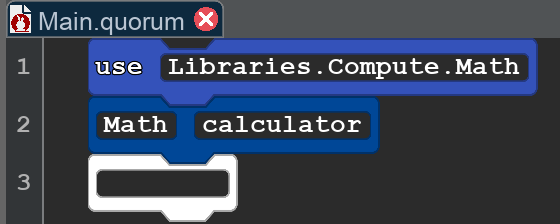
Although it looks different, this new block is making a new variable, just like you saw when you were working with the primitive types. Previously, you put simple values into your variables, like numbers or text. This time, you will put a complex value inside your container, called an Object. In this case, the object is a Math type, which is kind of like a scientific calculator you can use.
One approach to learning a library would be to read all of the documentation, which likely sounds similar in level of fun to watching paint dry. Another approach is to have the computer help us and learn on the fly. Inside the block palette, you can go to the Advice category. Then, select the line "Math calculator" in your code. This automatically tells the system to go lookup what that line of code does and provide you some information.
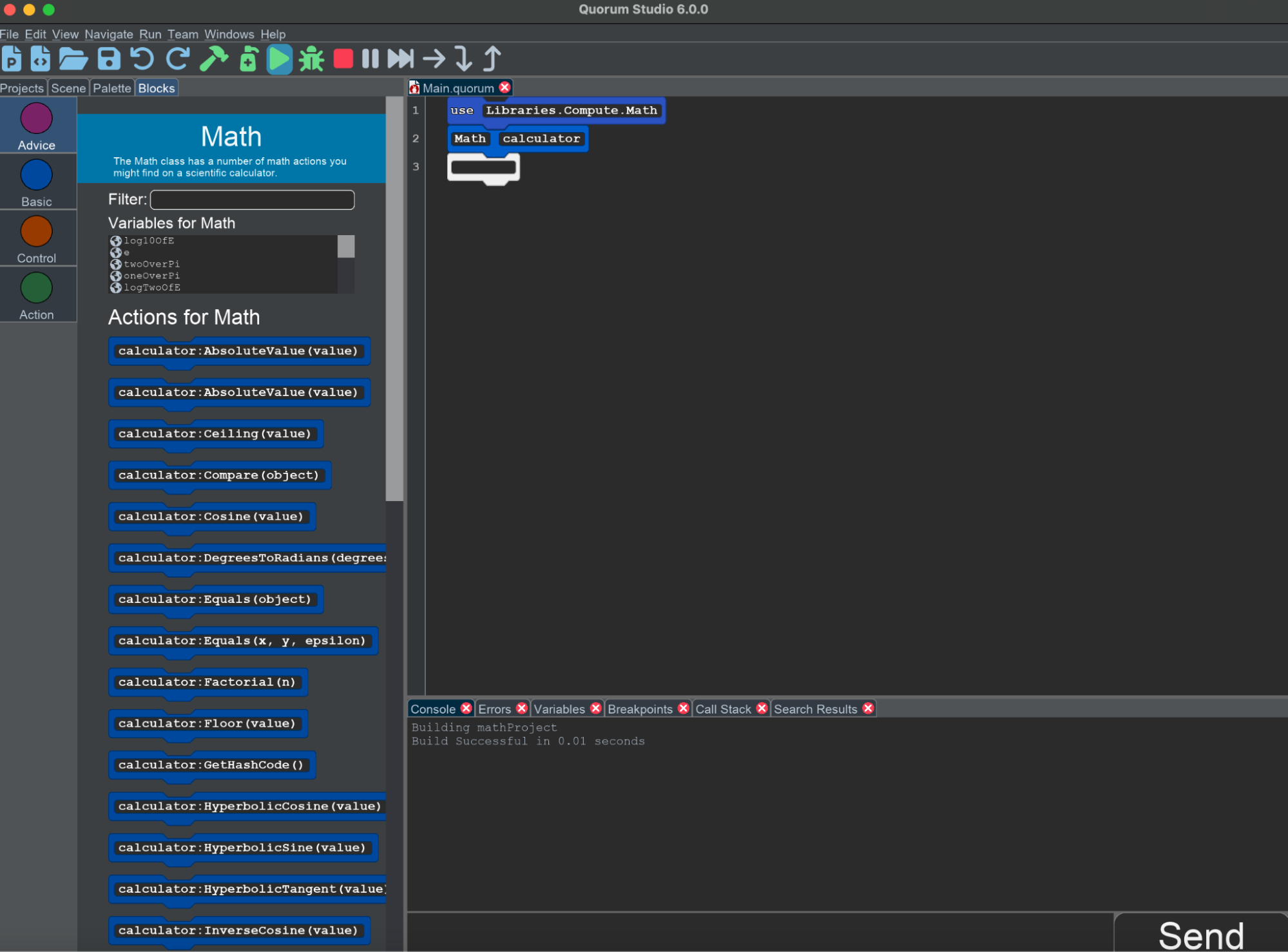
The Advice category provides hints about what you can do with a line of code or what it might mean. The most important part is the section labeled Actions. Actions are special tasks that Objects can perform. For example, the "Math" type object knows how to calculate absolute values or the cosine of an angle. Filters are available for searching through the options.
Actions have a specific structure when written as code. An action always begins with the name of the Object that is doing the task, followed by a colon. After the colon comes the name of the action that is being used, followed by a pair of parentheses. For example, with finding the Sine value of your "Math" object, the block of code would look like: calculator:Sine(value)
where value is placeholder for any numerical value.
Depending on the action, different tasks require a different amount of information inside the parenthesis. For example, calculating the cosine of an angle requires a number, which is the angle. The "RaiseToPower" action needs two pieces of information, the base number and the exponent. These pieces of information are called parameters.
Some actions do not need any parameters at all. Even if the action doesn’t need any extra information, though, the parenthesis are always added to the end of an action. The following is an example of using calculator:SquareRoot(value)
, which has an error in it.
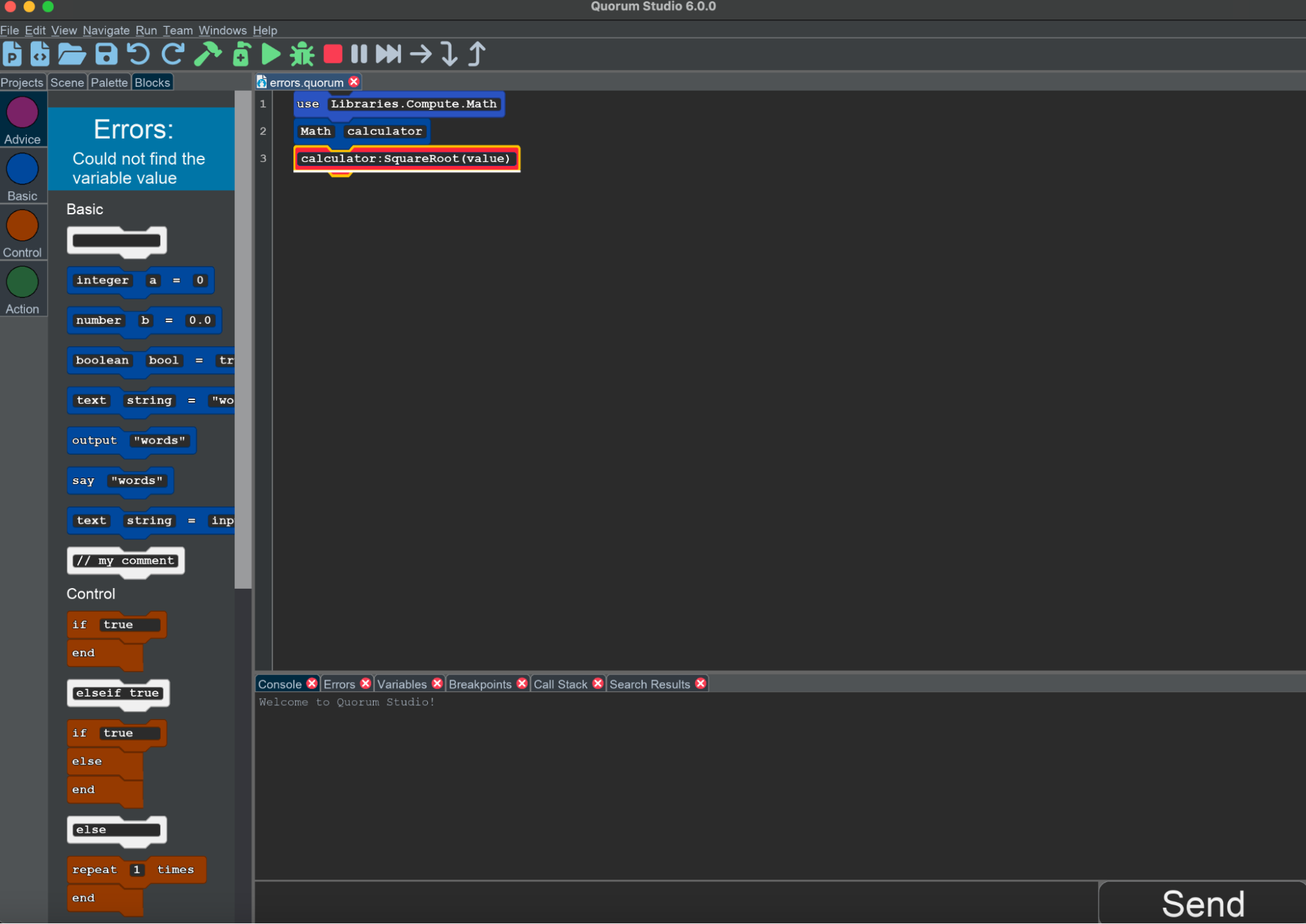
Just like when you put the "use" statement in, your new block has a placeholder in the text, the part that says "value." This placeholder is a parameter to the action is the name value, which does not exist. You might replace that word with a number, like 0. You can also put the value out to a variable or output it, like so in text:
number root = calculator:SquareRoot(4)
output root
Another view when graphically presented as the same code is as follows:
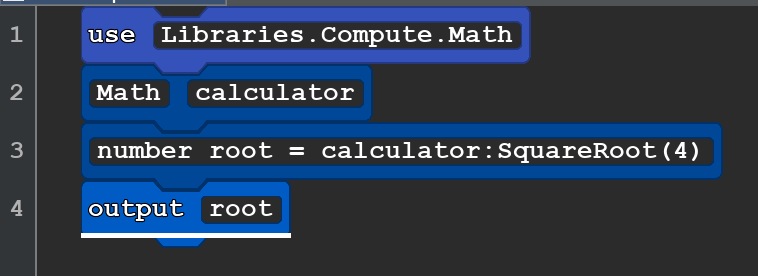
Engage
In this activity, you are going to go on a scavenger hunt to look for libraries that might interest you. You may be interested in learning to invent, or teaching others to invent, many different kinds of applications. This can vary in a seemingly endless number of ways. The goal here is to familiarize yourself with all of the options available in the current version of the standard library.
Directions
Pick a partner to do the scavenger hunt with. You are going to explore as a group different libraries for various kinds of applications. Your broad goal is to find two libraries in each of the following categories:
- Interfaces
- Data Science
- Computer Graphics
- Accessibility
- Two more categories of your choosing
As you go on your scavenger hunt, write down which libraries you think might be the most interesting and most useful. For the two you found the most interesting, look at the actions that the library can take and identify three of each you think might perform an interesting behavior.
You may refer to the following links on the Quorum website to get you started in finding these libraries: Quorum Standard Library index page and Quorum Language Reference page.
Wrap up
Once you are done, if time allows, each group can share aloud their top two most interesting libraries. Consider as a group what might be good targets for using in specifically computer science education.
Next Tutorial
In the next tutorial, we will discuss Boo Boo Management, which describes types of errors in Quorum.