Hour 3: Variables and Operators
This lesson is to teach you about variables and operators.Overview
When writing programs, you give the computer a set of instructions to perform. As part of these instructions, you can give it specific data, called variables, at each step. When you hear the term variables, you might relate this topic to saving information. Your computer might save items you download, data you are keeping track of, or information about how you login to a website. In this lesson, you will learn about how to store information in a program using variables. You will also learn how to use operators, like math symbols, to change and combine this stored information.
Goals
You have the following goals for this lesson:
- Learn how to store information with variables
- Use the four "primitive" variable types in Quorum
- Use operators to modify variables
Warm up
Consider a few examples of technology in your life. For each of the examples below, what kinds of data do you think are being stored or changed?
- Calculator
- Car speedometer
- Digital light switch
- e-Book
- Microwave
Can you think of other examples of technology that needs to store and change data?
Vocabulary
You will be learning about the following vocabulary words:
Term | Definition |
---|---|
Variable | A variable is a storage container. It has a name, a data type, and a value. |
integer | Integers are whole numbers. They can never have a decimal point. |
number | A number is a numerical value with a decimal point. |
text | Text is any combination of written symbols, like letters or numbers. |
boolean | A boolean variable can be only true or false. |
Primitive Variable | A primitive variable is a variable that stores one of the four basic types of information: integers, numbers, booleans, or text. |
Operator | An operator is a symbol that changes or combines pieces of data. The most common operators are math symbols like +, -, *, or /. |
Code
You will be using the following new pieces of code:
Quorum Code | Code Example | Explanation |
---|---|---|
integer NAME = VALUE | integer a = 0 | Creates a new variable called NAME that can store integers, and puts VALUE in the container. |
number NAME = VALUE | number b = 1.8 | Creates a new variable called NAME that can store numbers, and puts VALUE in the container. |
text NAME = VALUE | text string = "McDonald’s" | Creates a new variable called NAME that can store text, and puts VALUE in the container. |
boolean NAME = VALUE | boolean bool = true | Creates a new variable called NAME that can store booleans, and puts VALUE in the container. |
CSTA Standards
This lesson covers the following standards:
- 2-AP-11: Create clearly named variables that represent different data types and perform operations on their values.
- 3A-DA-09: Translate between different bit representations of real-world phenomena, such as characters, numbers, and images.
- 3A-DA-10: Evaluate the tradeoffs in how data elements are organized and where data is stored.
Explore
Before you begin, you will need to make a new project to work on. As a reminder, you can make a new project by going to the File Menu and selecting the New Project button. For the project type, select "Console." A console project really means that it is a blank canvas and you can then have it create whatever you like. Once you have made your project, open the "Main.quorum" file in the project tree on the left. Then, open the "Blocks" tab in the left pane. For this lesson, open the "Basic" tab. Your project should look like the picture below.
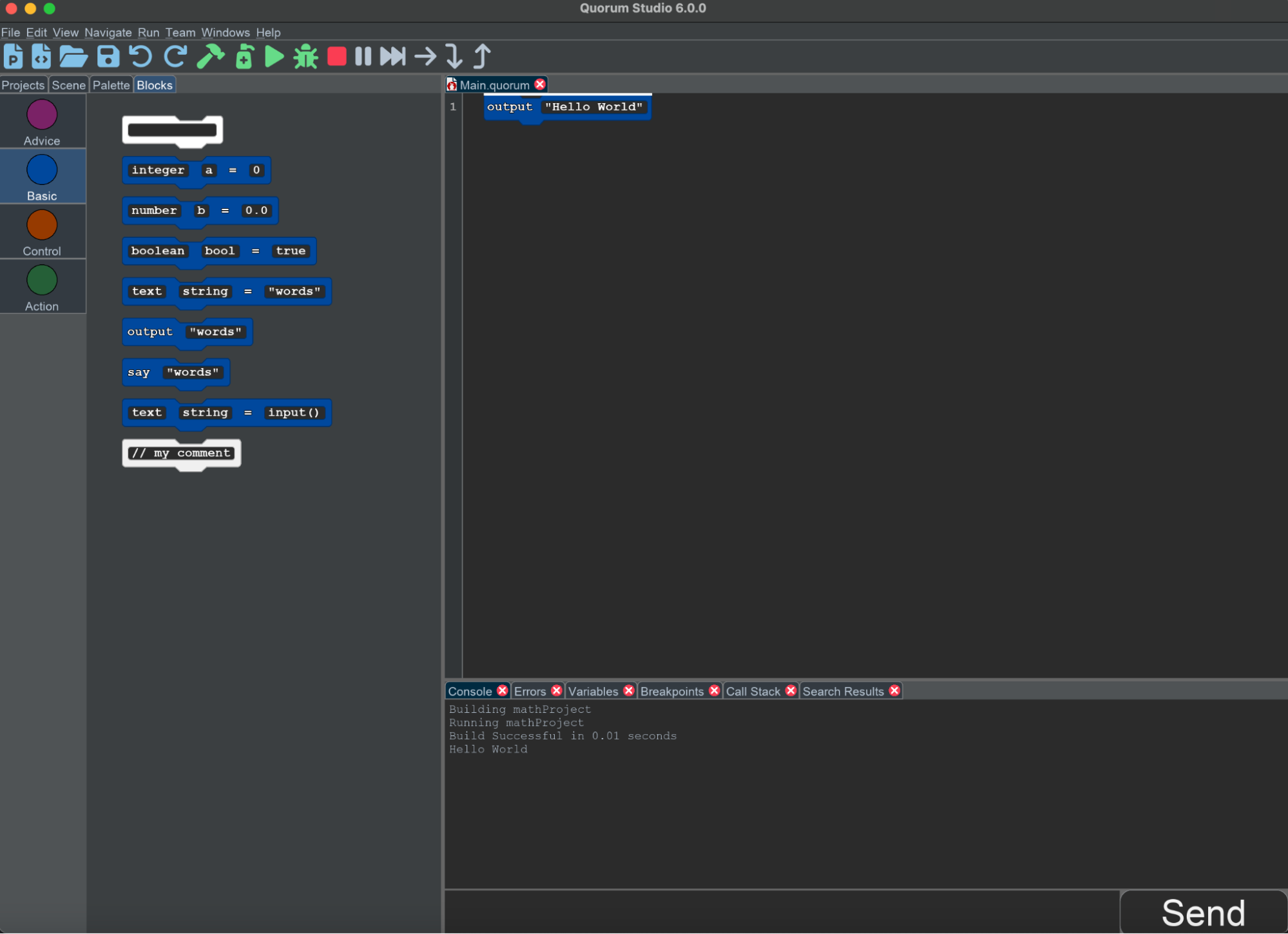
In previous lessons, when you have given instructions to the computer, like "output" or "say", you have given it specific words or other data to use on that line of code. You can tell an output or say statement to interact with a constant, like the integer 5, but you can also use variables.
A variable is a container for information. You can think of it like a box. The box has a label on it that describes what kind of stuff can go inside. The box also has a name written on it, so you can tell different boxes apart.
There are several blocks on the left side for variables. To start, think about one:
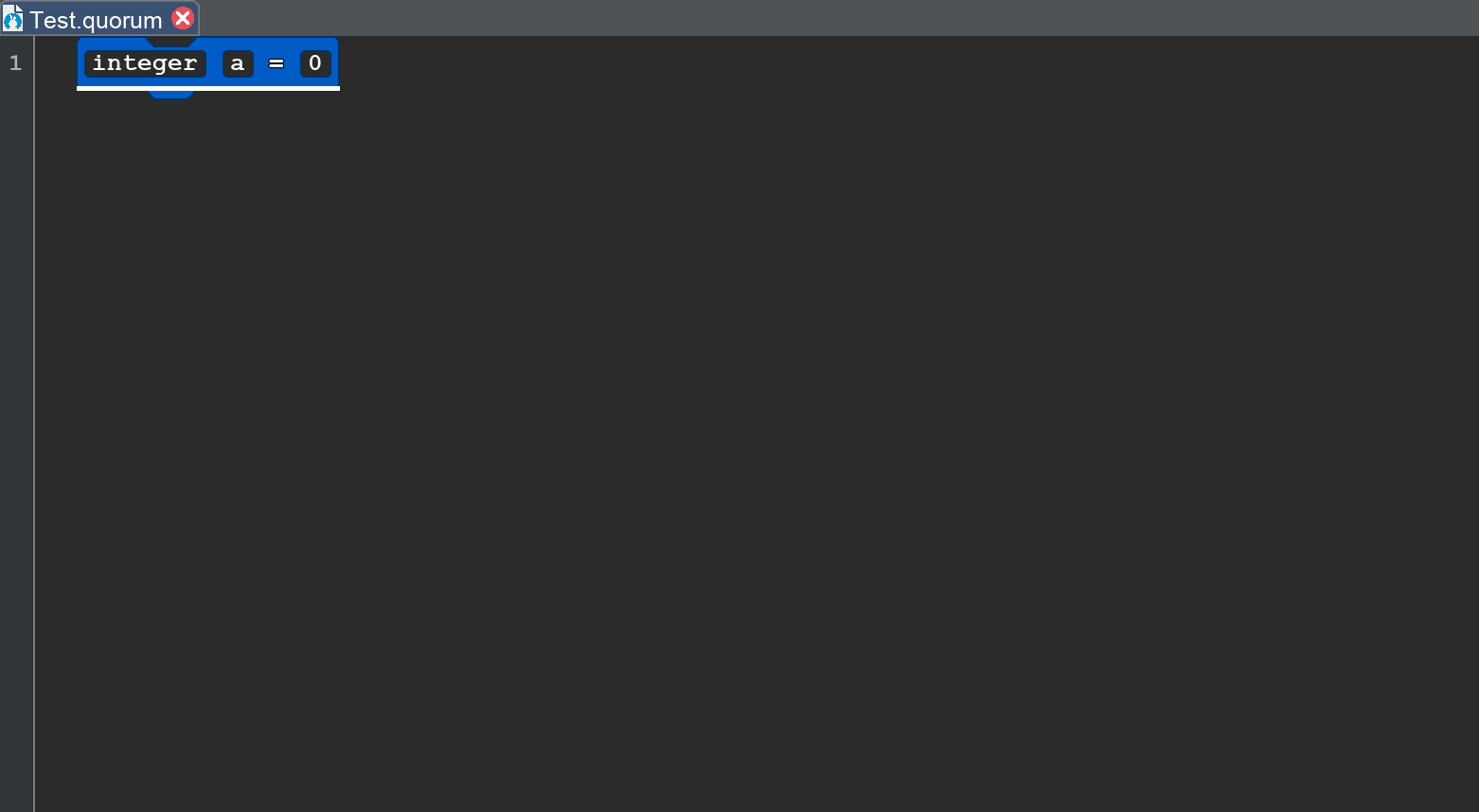
First, while the representation above is visual, computer programming often distinguishes between code and such visuals, like blocks. In the Quorum programming language, this distinction does not exist. The above is exactly, mathematically, identical to the line of code:
integer a = 0
Point being, throughout this lesson and the rest, just consider that while the language allows a block or text mode, what they both do is identical. It is purely personal preference which you or your students use. Both are accessible and any program can be written with either. In fact, when Quorum sends a block to be processed for creating the program you are writing, it is not using blocks at all. It just uses the text, but happens to have a visual representation that looks like a block and has extra information embedded for accessibility devices like screen readers. If the word block is referenced, thus, in this material, it is interchangeable with text.
This block creates a variable, and it has four parts. The first box reads "integer", which describes the type of information that can be stored in the variable. The second box reads "a", which is the variable's name. After the second box is an equal sign, which indicates assignment (which is just a fancy term for putting something in the variable box). The last box reads "0", which is the value of the variable, or in other words, the data being put in the box. For screen reader users, note that this type, name, value terminology is embedded into the screen reader cues, but varies slightly depending on platform and which screen reader is used.
An Introduction to Variables
Now consider how to make primitive variables, which in this context means one of the four basic types: integer, number, boolean, or text. To start, place the "integer a = 0" block at the top of your program. Then, place a "say" or "output" block after it.
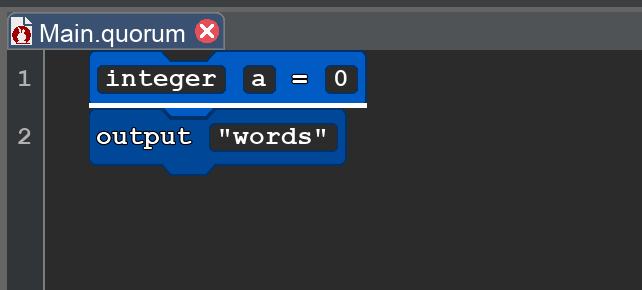
Your program now has a variable, but you are not doing anything with it yet. If you place the name of the variable into the info box on your statement, the result would look like this:
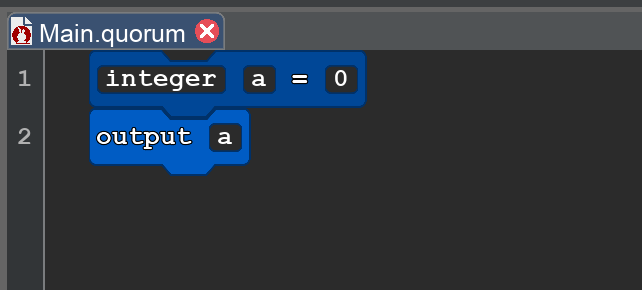
If you run your program, it will still output or say the integer 0, but it will do that by looking up what the value of the variable a is in memory, then use that. Remember, you can run your program from the Run menu by selecting the Run option, or by clicking the green "play" button on the toolbar, or by using the hotkey (Ctrl+R on Windows, or COMMAND+R on Mac).
When you run the program, it should output the value of the variable, which is 0.
Valid and Invalid Variable Names
In order to make your output statement use your variable, you have to give it a name. In big programs, there are often many variables, so giving them meaningful names that represent the kind of purpose they hold can be helpful to human readers. A variable name can be almost anything, but there a few rules:
- Variable names must start with a letter.
- A name can be made of lowercase letters, uppercase letters, numbers, and the underscore symbol, "_".
- Names cannot contain spaces or other special characters.
- While quirky, variables cannot end with the underscore character.
Variable Types
The first box of your variable block describes the "type" of the variable. The block palette on the left side has four kinds of variables:
- Integer are whole numbers. They can be positive or negative, but they never have a decimal point.
- Number are numerical values with a decimal point.
- Text is any sequence of text, including letters, numbers, special characters, etc. To indicate that something is text information instead of code, text is wrapped in quotation marks.
- Boolean are data with exactly two possible values: true or false.
These four data types are the building blocks of data and sometimes called primitive types. The reason is a bit complex, but has to do with how the computer stores certain kinds of data in memory as a program executes. In Quorum, it is also somewhat misleading, because text values are not stored this way. In any case, these are at least common types. The following shows a larger example of using several types of variables:
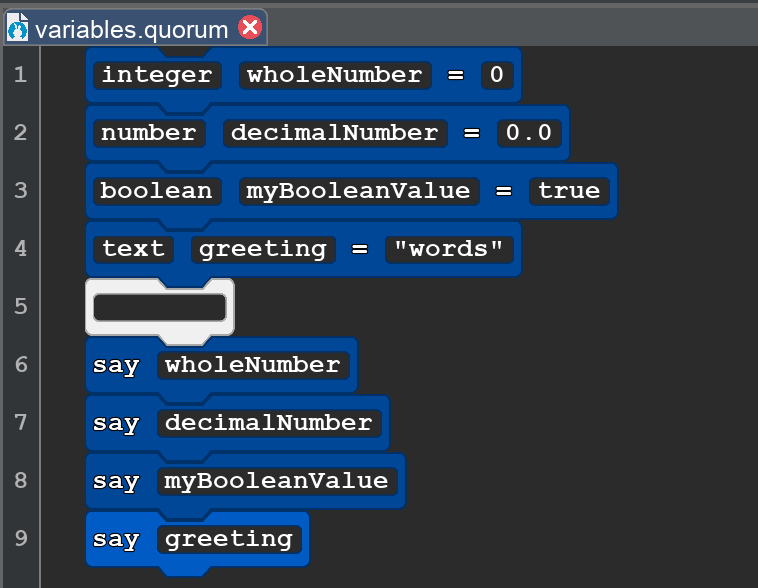
While the above is an image with an alternative description, in code, because the blocks are exactly equivalent to text, if you were to select all blocks and copy paste, you would have the following text:
integer wholeNumber = 0
number decimalNumber = 0.0
boolean myBooleanValue = true
text greetings = "words"
say wholeNumber
say decimalNumber
say myBooleanValue
say greetings
Operators
While programming, you often need to use math to run calculations. Fortunately, the operators young people learn are largely very similar to what programming languages do. The kind of operators you can use, and what they do, does, however, depend on the data type.
The operators described here all work in the same way. One value is placed on the left, another on the right, and an operator is placed in the middle. For example, "1 + 2" is a mathematical expression with the "plus" operator placed between the integers 1 and 2. The expression calculates how you might expect with an answer of 3.
Numerical Operators
For integers and numbers, you can use the four basic operations of math: addition (+), subtraction (-), multiplication (*), division (/). Integers also have modulus (mod), which calculates the remainder after integer division.
In your program, change the values of your integer and number variables or make a math expression in each using operators to calculate a new value. The image below shows one possible way of doing this.
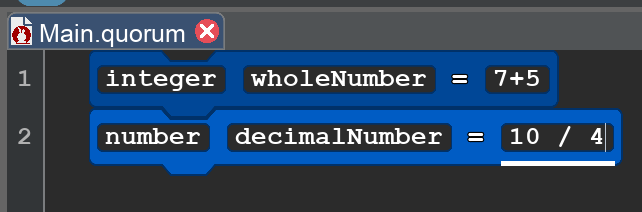
Again copy pasting from these blocks reveals is it identical to text, giving the following:
integer wholeNumber = 7 + 5
number decimalNumber = 10 / 4
Boolean Operators
You can also use comparison operators on integers and numbers. These are equals (=), less than (<), greater than (>), less than or equal to (<=), greater than or equal to (>=), and not equal (not=). These operations produce boolean values. For example, "5 < 6" produces the boolean value "true".
Boolean values also use equal (=) and not equal (not=). They also use the logical operators "and" and "or" to combine values. The "and" operator produces the boolean value true only if both the left and right value are also true, or produces false if either of them are false. "or" produces the boolean value true if at least one of the two values are true, and only produces false if both values are false.
Booleans also have a special operator, called "not". Unlike the other operators discussed here, "not" only takes one value, not two. "not" flips the value of true or false. For example, "not true" produces false, and "not false" produces true.

Again, while the above looks like a block, it is identical to the following text:
boolean myBooleanValue = true or false
Text Operators
Text values only use one operator, which is the plus symbol (+). When used for text, the plus symbol performs concatenation, or in other words, it adds one piece of text to the end of another. For example, "hello " + "world" would produce "hello world". This idea of concatenation is a higher level topic when it comes to text variable management, but the main idea to note is that you use it to combine text. Here is an example:

The text equivalent is identical:
text greeting = ''Hi '' + ''there!''
Here is one more example with:
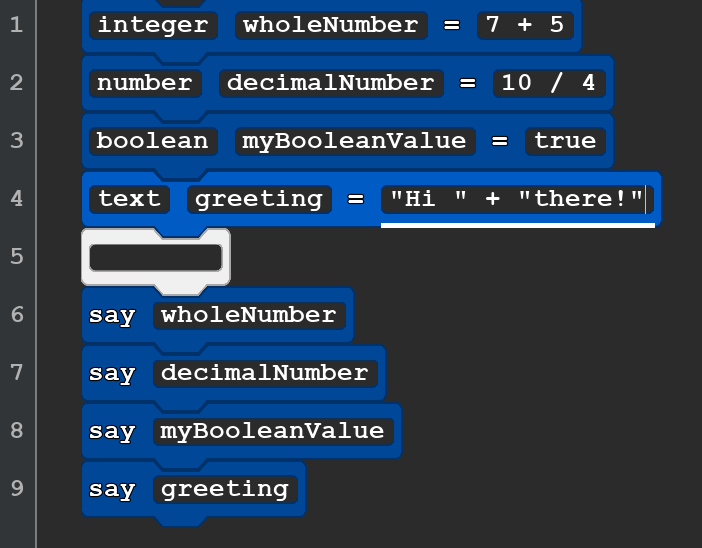
The text is again equivalent:
integer wholeNumber = 7 + 5
number decimalNumber = 10 / 4
boolean myBooleanValue = true or false
text greetings = "Hi " + "there!"
say wholeNumber
say decimalNumber
say myBooleanValue
say greetings
Inputs and Typecasting
In some circumstances, perhaps you want to retrieve user input for a variable to do something with it. The input() command allows you to take user input and save it into a text variable. This block is available for you to use within the "Basics" category.
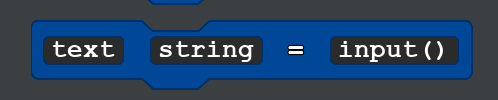
Inside of the parentheses of input, you are able to put a command or question in which the answer you type out will be saved into the text string variable. For example:

When you run this piece of code and input your answer into the console, whatever answer you send into Quorum saves the value into the milkFlavor variable. Regardless if your answer is a numerical value or text value, by default, it saves as a text value. Therefore, another operator to know is learning how to convert text variables to integers or numbers using cast().
Typecasting is a useful method to convert these numbers into their correct types. In this block of code, the program is asking how many hours of sleep you get via the input method.

The lines of this program read in plain text:
text sleep = input("How many hours of sleep do you get?")
integer hours = cast(integer, sleep)
integer need8hours = 8 - hours
output "You get " + hours + " of sleep. You need " + need8hours + " hours to get the full 8 hours of sleep!"
Notice how the input saves as a text variable, although you are asking for an integer. You can use the action, cast(type, variableToCast) to convert the text value into an integer as it is shown in line 2. The program saves the value as an integer and can be used in calculations to get the answer in the output. Feel free to copy this code snippet onto Quorum Studio to see how it works.
Engage
After learning how to create variables and use these variables when working with operators, you will practice these skills on your own. You will be creating variables for each Quorum data type and outputting them into the console:
- Integer
- Number
- Text
- Boolean
Additionally, with all the datatypes you will also be practicing how to use the operators specific to each type. This section is a bit rote, but practice like this, even if brief, can help get a shared understanding of an extremely important concept in computer science.
Directions
One day out of the blue you and a partner leave your corporate life and want to move to country life. The hustle culture you have faced prior just became too much and now you suddenly want to live a slower and simple life. Using the money you had in the bank, you buy a small farm and want to grow vegetables and fruits. It is the start of spring and you have to plan out your whole season to grow vegetables effectively. You are going to write a small program to document your farm.
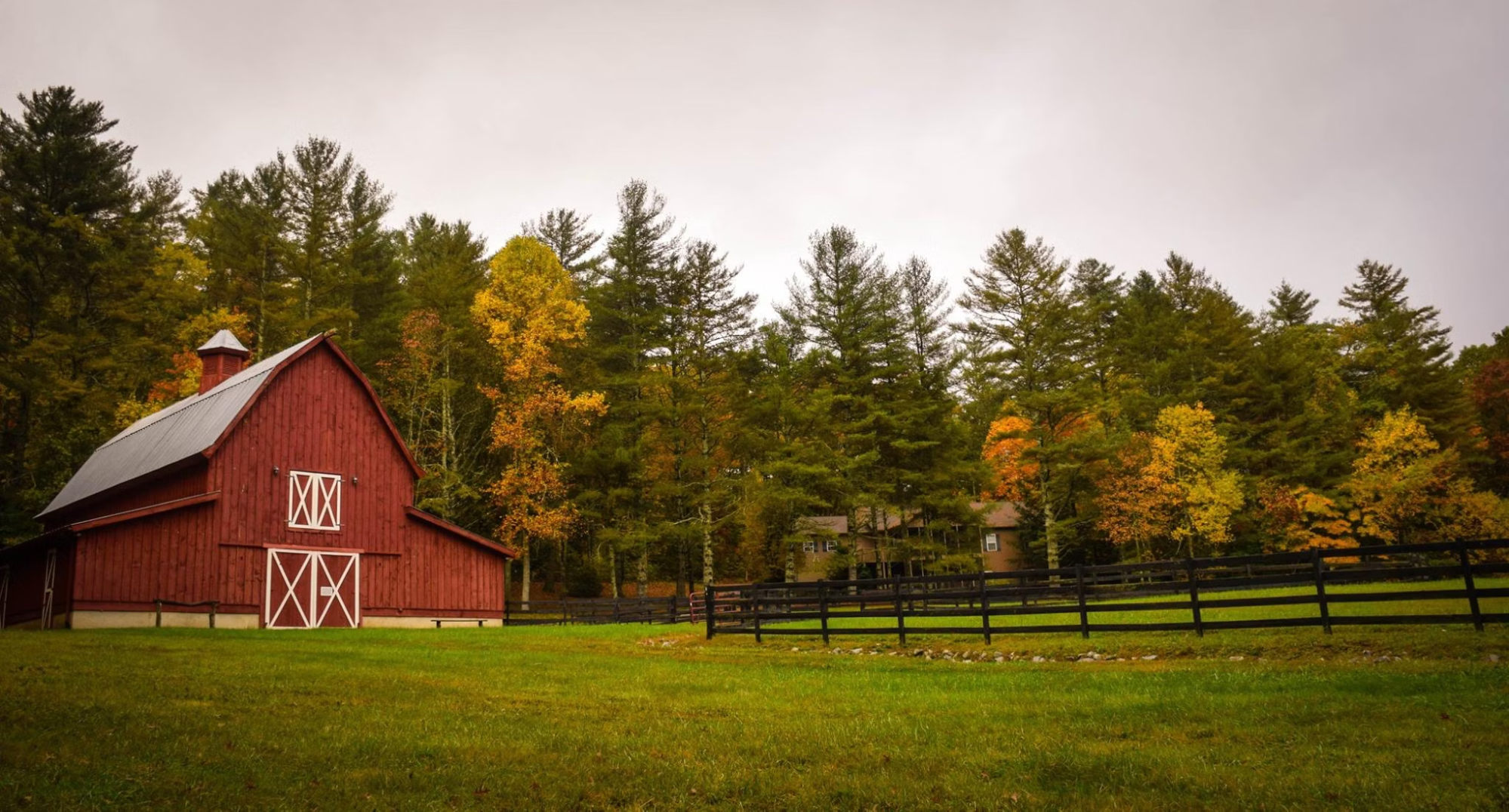
To get started on this program, you will need to download the following template to get started. Please note that if you run this program, it will lead to an error because variables that you need have not been created yet.
Task 1: Name Your Farm
You will first want to name your farm, so create a text variable, saving the value with the name. You can also practice using the text variable operation to add two words together. For example:
text name = "Mickey " + "Mouse " + "Farm"
Output your farm name with a little greeting to practice your text operations.
Task 2: Grow Your Crops
On your farm you have the choice to grow these or other types of fruits and vegetables:
- Apricots
- Apples
- Garlic
- Pineapples
- Strawberries
- Broccoli
- Lettuce
- Onions
- Mushrooms
- Turnips
You have 10 plots available on your land to grow your produce. You will then create integer variables and decide how much of each product you want to grow. After deciding what to grow, add up all the fruit and veggie variables in order to verify you have all 10 plots filled and save this in a new variable named total.
Then, use the output or say statement to display all your crops. You may follow the following format when outputting the crop amounts.

Task 3: Check Your Plots
Lastly, create 1 boolean variable: isPlotFilled and set it to false. Using the 1st if statement block provided and check if total = 10. If total = 10 set isPlotFilled equal to true and then output a statement saying the plot has been filled. Otherwise, if the plot has not been filled, modify the output inside of the else block to say that plot has not been filled.
When isPlotFilled is set to true, it will run the additional output statement in the template provided.
Sample Output
The following shows two sample outputs from the program.
Sample output when all plots are filled:
Welcome to Mango Girl Farm. In this farm you will find the following fruits and vegetables:
Apricots: 2
Broccoli: 5
Mushrooms: 3
You filed up all your plots!
Your hard work has been paid off! By fall you should have many crops to harvest!
Sample output when not all the plots are filled:
Welcome to Mango Girl Farm. In this farm you will find the following fruits and vegetables:
Apricots: 2
Broccoli: 5
Mushrooms: 2
You need to grow more crops!
Wrap up
Once you are finished with your program, discuss what you think might be common misunderstandings with students and variables. Are some harder than others? Are some operations easier to understand, or more important, than others?
Next Tutorial
In the next tutorial, we will discuss Libraries and Objects, which describes how to read documentation in the Standard Library and create objects.